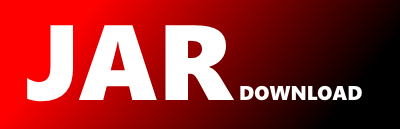
gov.nasa.worldwind.util.layertree.LayerTreeNode Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 United States Government as represented by the Administrator of the
* National Aeronautics and Space Administration.
* All Rights Reserved.
*/
package gov.nasa.worldwind.util.layertree;
import gov.nasa.worldwind.avlist.AVKey;
import gov.nasa.worldwind.layers.Layer;
import gov.nasa.worldwind.util.Logging;
import gov.nasa.worldwind.util.tree.BasicTreeNode;
/**
* A TreeNode
that represents a {@link gov.nasa.worldwind.layers.Layer}
.
*
* The node's selection state is synchronized with its Layer
's enabled state. {@link
* #isSelected()}
returns whether the node's Layer
is enabled. Calling {@link
* #setSelected(boolean)}
specifies both the the node's selection state, and whether its Layer
* should be enabled for rendering and selection.
*
* @author pabercrombie
* @version $Id: LayerTreeNode.java 1171 2013-02-11 21:45:02Z dcollins $
*/
public class LayerTreeNode extends BasicTreeNode
{
/** The layer node's default icon path. */
protected static final String DEFAULT_IMAGE = "images/16x16-icon-earth.png";
/**
* Indicates the Layer
this node represents. Initialized to a non-null
value during
* construction.
*/
protected Layer layer;
/**
* Creates a new LayerTreeNode
from the specified layer
. The node's name is set to the
* layer's name.
*
* @param layer the Layer
this node represents.
*
* @throws IllegalArgumentException if the layer
is null
.
*/
public LayerTreeNode(Layer layer)
{
super(layer != null ? layer.getName() : "");
if (layer == null)
{
String message = Logging.getMessage("nullValue.LayerIsNull");
Logging.logger().severe(message);
throw new IllegalArgumentException(message);
}
this.layer = layer;
this.initialize();
}
/** Initializes this node's image source. */
protected void initialize()
{
Object imageSource = this.layer.getValue(AVKey.IMAGE);
if (imageSource == null)
imageSource = DEFAULT_IMAGE;
this.setImageSource(imageSource);
}
/**
* Indicates whether this node's Layer
is enabled for rendering and selection.
*
* @return true
if the Layer
is enabled, otherwise false
.
*/
@Override
public boolean isSelected()
{
return this.layer.isEnabled();
}
/**
* Specifies whether this node's Layer
is enabled for rendering and selection. This sets both the
* node's selection state and its Layer
's enabled state.
*
* @param selected true
to enable the Layer
, otherwise false
.
*/
@Override
public void setSelected(boolean selected)
{
super.setSelected(selected);
this.layer.setEnabled(selected);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy