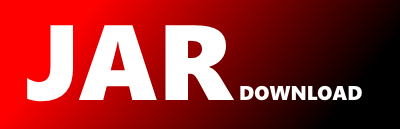
lombok.ast.ClassDecl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lombok-pg Show documentation
Show all versions of lombok-pg Show documentation
lombok-pg is a collection of extensions to Project Lombok
/*
* Copyright © 2011 Philipp Eichhorn
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package lombok.ast;
import static lombok.ast.Modifier.*;
import java.util.*;
import lombok.*;
@Getter
public class ClassDecl extends Statement {
protected final EnumSet modifiers = EnumSet.noneOf(Modifier.class);
private final List annotations = new ArrayList();
private final List typeParameters = new ArrayList();
private final List fields = new ArrayList();
private final List> methods = new ArrayList>();
private final List memberTypes = new ArrayList();
private final List superInterfaces = new ArrayList();
private final String name;
private TypeRef superclass;
private boolean local;
private boolean anonymous;
private boolean isInterface;
public ClassDecl(final String name) {
this.name = name;
}
public ClassDecl extending(final TypeRef type) {
superclass = type;
return this;
}
public ClassDecl implementing(final TypeRef type) {
superInterfaces.add(child(type));
return this;
}
public ClassDecl implementing(final List types) {
for (TypeRef type : types) implementing(type);
return this;
}
public ClassDecl makeLocal() {
local = true;
return this;
}
public ClassDecl makeAnonymous() {
anonymous = true;
return this;
}
public ClassDecl makeInterface() {
isInterface = true;
return this;
}
public ClassDecl makePrivate() {
return withModifier(PRIVATE);
}
public ClassDecl makeProtected() {
return withModifier(PROTECTED);
}
public ClassDecl makePublic() {
return withModifier(PUBLIC);
}
public ClassDecl makeStatic() {
return withModifier(STATIC);
}
public ClassDecl makeFinal() {
return withModifier(FINAL);
}
public ClassDecl withModifier(final Modifier modifier) {
modifiers.add(modifier);
return this;
}
public ClassDecl withMethod(final AbstractMethodDecl> method) {
methods.add(child(method));
return this;
}
public ClassDecl withMethods(final List> methods) {
for (AbstractMethodDecl> method : methods) withMethod(method);
return this;
}
public ClassDecl withField(final FieldDecl field) {
fields.add(child(field));
return this;
}
public ClassDecl withFields(final List fields) {
for (FieldDecl field : fields) withField(field);
return this;
}
public ClassDecl withType(final ClassDecl type) {
memberTypes.add(child(type));
return this;
}
public ClassDecl withTypeParameter(final TypeParam typeParameter) {
typeParameters.add(child(typeParameter));
return this;
}
public ClassDecl withTypeParameters(final List typeParameters) {
for (TypeParam typeParameter : typeParameters) withTypeParameter(typeParameter);
return this;
}
@Override
public RETURN_TYPE accept(final ASTVisitor v, final PARAMETER_TYPE p) {
return v.visitClassDecl(this, p);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy