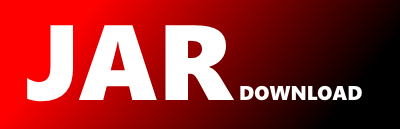
com.github.peterbecker.configuration.storage.YamlStore Maven / Gradle / Ivy
package com.github.peterbecker.configuration.storage;
import com.github.peterbecker.configuration.ConfigurationException;
import org.yaml.snakeyaml.DumperOptions;
import org.yaml.snakeyaml.Yaml;
import org.yaml.snakeyaml.constructor.Constructor;
import org.yaml.snakeyaml.representer.Representer;
import org.yaml.snakeyaml.resolver.Resolver;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.List;
import java.util.Map;
import java.util.Optional;
@SuppressWarnings("unchecked")
public class YamlStore implements Store {
private final Map data;
@SuppressWarnings("unchecked")
public YamlStore(Path resource) throws IOException {
Yaml yaml = new Yaml(
new Constructor(), // default
new Representer(), // default
new DumperOptions(), // default
new CustomResolver());
data = yaml.load(Files.newBufferedReader(resource));
}
@Override
public Optional getValue(Key key) throws ConfigurationException {
return getNode(data,key).map(Object::toString);
}
private Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy