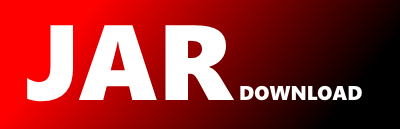
smallcheck.generators.GenFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-smallcheck Show documentation
Show all versions of java-smallcheck Show documentation
An implementation of SmallCheck for Java as a JUnit extension.
The newest version!
package smallcheck.generators;
import smallcheck.annotations.From;
import java.lang.reflect.*;
import java.util.*;
import java.util.function.Function;
/**
*
*/
public class GenFactory {
private Map> typeGenerators = initDefaultGenerators();
private List staticFactories = new ArrayList<>();
private static class StaticFactory {
Class> clazz;
Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy