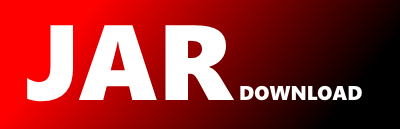
com.github.petruki.switcher.client.service.ClientServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of switcher-client Show documentation
Show all versions of switcher-client Show documentation
Switcher Client for working with Switcher API
package com.github.petruki.switcher.client.service;
import java.util.Map;
import javax.ws.rs.client.Client;
import javax.ws.rs.client.ClientBuilder;
import javax.ws.rs.client.Entity;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import org.apache.log4j.Logger;
import com.github.petruki.switcher.client.domain.AuthRequest;
import com.github.petruki.switcher.client.domain.AuthResponse;
import com.github.petruki.switcher.client.domain.Switcher;
import com.github.petruki.switcher.client.utils.SwitcherContextParam;
/**
* @author rogerio
* @since 2019-12-24
*/
public class ClientServiceImpl implements ClientService {
private static final Logger logger = Logger.getLogger(ClientServiceImpl.class);
private Client client;
public ClientServiceImpl() {
this.setClient(ClientBuilder.newClient());
}
public Response executeCriteriaService(final Map properties,
final Switcher switcher) throws Exception {
if (logger.isDebugEnabled()) {
logger.debug(String.format("switcher: %s", switcher));
}
final WebTarget myResource = client.target((String) properties.get(SwitcherContextParam.URL))
.queryParam(Switcher.KEY, switcher.getKey())
.queryParam(Switcher.SHOW_REASON, Boolean.TRUE)
.queryParam(Switcher.BYPASS_METRIC, properties.containsKey(Switcher.BYPASS_METRIC) ?
properties.get(Switcher.BYPASS_METRIC) : false);
final Response response = myResource.request(MediaType.APPLICATION_JSON)
.header(HEADER_AUTHORIZATION, String.format(TOKEN_TEXT, ((AuthResponse) properties.get(AUTH_RESPONSE)).getToken()))
.post(Entity.json(switcher.getInputRequest()));
return response;
}
public Response auth(final Map properties) throws Exception {
final AuthRequest authRequest = new AuthRequest();
authRequest.setDomain((String) properties.get(SwitcherContextParam.DOMAIN));
authRequest.setComponent((String) properties.get(SwitcherContextParam.COMPONENT));
authRequest.setEnvironment((String) properties.get(SwitcherContextParam.ENVIRONMENT));
final WebTarget myResource = client.target(String.format(AUTH_URL, properties.get(SwitcherContextParam.URL)));
final Response response = myResource.request(MediaType.APPLICATION_JSON)
.header(HEADER_APIKEY, properties.get(SwitcherContextParam.APIKEY))
.post(Entity.json(authRequest));
return response;
}
public void setClient(Client client) {
this.client = client;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy