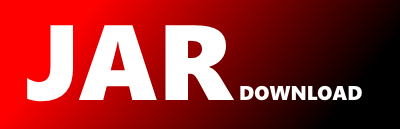
com.github.phantomthief.concurrent.TryWaitResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of more-lambdas Show documentation
Show all versions of more-lambdas Show documentation
Some useful lambda implements for Java 8.
package com.github.phantomthief.concurrent;
import static com.github.phantomthief.util.MoreSuppliers.lazy;
import static com.google.common.base.MoreObjects.toStringHelper;
import static java.util.stream.Collectors.toMap;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.CancellationException;
import java.util.concurrent.Future;
import java.util.concurrent.TimeoutException;
import java.util.function.Supplier;
import javax.annotation.Nonnull;
/**
* @author w.vela
* Created on 2018-06-25.
*/
class TryWaitResult {
private final Map, V> success;
private final Map, Throwable> failed;
private final Map, TimeoutException> timeout;
private final Map, CancellationException> cancel;
private final Map, K> futureMap;
private final Supplier
© 2015 - 2024 Weber Informatics LLC | Privacy Policy