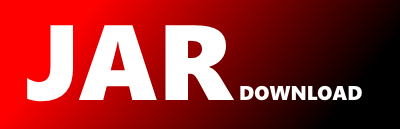
com.github.phantomthief.util.MoreFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of more-lambdas Show documentation
Show all versions of more-lambdas Show documentation
Some useful lambda implements for Java 8.
package com.github.phantomthief.util;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.base.Throwables.throwIfUnchecked;
import static java.util.Optional.ofNullable;
import static org.slf4j.LoggerFactory.getLogger;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.ForkJoinTask;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.BiPredicate;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Stream;
import javax.annotation.Nonnull;
import org.slf4j.Logger;
/**
* @author w.vela
*/
public final class MoreFunctions {
private static final Logger logger = getLogger(MoreFunctions.class);
private static final String FAIL_SAFE_MARK = "[fail safe]";
public static Optional catchingOptional(Callable callable) {
return ofNullable(catching(callable));
}
public static R catching(Callable callable) {
return catching(callable, e -> logger.error(FAIL_SAFE_MARK, e));
}
public static void runCatching(ThrowableRunnable callable) {
catching(() -> {
callable.run();
return null;
}, e -> logger.error(FAIL_SAFE_MARK, e));
}
public static R throwing(Callable callable) {
return catching(callable, throwable -> {
throwIfUnchecked(throwable);
throw new RuntimeException(throwable);
});
}
public static void runThrowing(ThrowableRunnable callable) {
catching(() -> {
callable.run();
return null;
}, throwable -> {
throwIfUnchecked(throwable);
throw new RuntimeException(throwable);
});
}
/**
* better use traditional try-catch for readability.
*/
@Deprecated
public static R catching(Callable callable,
ThrowableConsumer exceptionHandler) throws X {
try {
return callable.call();
} catch (Throwable e) {
exceptionHandler.accept(e);
return null;
}
}
public static R catching(ThrowableFunction function, T t) {
return catching(function, t, e -> logger.error(FAIL_SAFE_MARK, e));
}
public static R throwing(ThrowableFunction function, T t) {
return catching(function, t, throwable -> {
throwIfUnchecked(throwable);
throw new RuntimeException(throwable);
});
}
/**
* better use traditional try-catch for readability.
*/
@Deprecated
public static R catching(
ThrowableFunction function, T t,
ThrowableConsumer exceptionHandler) throws X {
try {
return function.apply(t);
} catch (Throwable e) {
exceptionHandler.accept(e);
return null;
}
}
/**
* @see #supplyParallel(ForkJoinPool, ThrowableSupplier)
*/
public static void runParallel(ForkJoinPool pool,
ThrowableRunnable func) throws X {
supplyParallel(pool, () -> {
func.run();
return null;
});
}
/**
* mainly use for {@link Stream#parallel()} with specific thread pool
* see https://stackoverflow.com/questions/21163108/custom-thread-pool-in-java-8-parallel-stream
*/
public static R supplyParallel(ForkJoinPool pool,
ThrowableSupplier func) throws X {
checkNotNull(pool);
Throwable[] throwable = { null };
ForkJoinTask task = pool.submit(() -> {
try {
return func.get();
} catch (Throwable e) {
throwable[0] = e;
return null;
}
});
R r;
try {
r = task.get();
} catch (ExecutionException | InterruptedException impossible) {
throw new AssertionError(impossible);
}
if (throwable[0] != null) {
//noinspection unchecked
throw (X) throwable[0];
}
return r;
}
public static void runWithThreadName(
@Nonnull Function name, @Nonnull ThrowableRunnable func) throws X {
supplyWithThreadName(name, () -> {
func.run();
return null;
});
}
public static T supplyWithThreadName(
@Nonnull Function name, @Nonnull ThrowableSupplier func)
throws X {
Thread currentThread = Thread.currentThread();
String originalThreadName = currentThread.getName();
String newName = name.apply(originalThreadName);
if (newName != null) {
currentThread.setName(newName);
}
try {
return func.get();
} finally {
currentThread.setName(originalThreadName);
}
}
public static Function, T> mapKv(BiFunction func) {
return entry -> func.apply(entry.getKey(), entry.getValue());
}
public static Predicate> filterKv(BiPredicate func) {
return entry -> func.test(entry.getKey(), entry.getValue());
}
public static Consumer> consumerKv(BiConsumer func) {
return entry -> func.accept(entry.getKey(), entry.getValue());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy