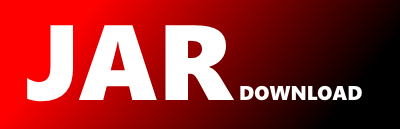
com.github.phantomthief.util.MorePredicates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of more-lambdas Show documentation
Show all versions of more-lambdas Show documentation
Some useful lambda implements for Java 8.
package com.github.phantomthief.util;
import static java.util.Collections.newSetFromMap;
import java.util.Objects;
import java.util.Random;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
/**
* @author w.vela
*/
public final class MorePredicates {
private MorePredicates() {
throw new UnsupportedOperationException();
}
public static Predicate not(Predicate predicate) {
return t -> !predicate.test(t);
}
public static Predicate applyOtherwise(Predicate predicate,
Consumer negateConsumer) {
return t -> {
boolean result = predicate.test(t);
if (!result) {
negateConsumer.accept(t);
}
return result;
};
}
public static Predicate distinctUsing(Function mapper) {
return new Predicate() {
private final Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy