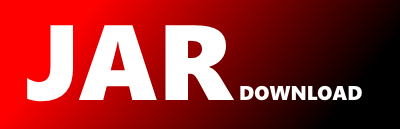
com.github.phantomthief.failover.impl.WeightFailover Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simple-failover Show documentation
Show all versions of simple-failover Show documentation
A simple failover library for Java
package com.github.phantomthief.failover.impl;
import static com.github.phantomthief.tuple.Tuple.tuple;
import static java.lang.Integer.MAX_VALUE;
import static java.lang.Math.max;
import static java.lang.Math.min;
import static java.util.Collections.emptySet;
import static java.util.Collections.unmodifiableList;
import static java.util.stream.Collectors.toSet;
import static org.slf4j.LoggerFactory.getLogger;
import java.io.Closeable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map.Entry;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.ThreadLocalRandom;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.function.Consumer;
import java.util.function.IntUnaryOperator;
import java.util.function.Predicate;
import javax.annotation.Nullable;
import org.slf4j.Logger;
import com.github.phantomthief.failover.Failover;
import com.github.phantomthief.tuple.TwoTuple;
import com.github.phantomthief.util.MoreSuppliers.CloseableSupplier;
import com.google.common.collect.ImmutableList;
/**
* 基于权重的failover,失败减,成功加,这是个早期的实现,用的也非常多,
* 现在建议使用{@link com.github.phantomthief.failover.SimpleFailover SimpleFailover}接口
* 和{@link PriorityFailover}实现。
*
* 需要注意的是:1、WeightFailover的权重是整数。2、WeightFailover不区分初始权重和最大权重(即初始权重总是等于最大权重)。
*
*
* 请先阅读README.md,可以到这里在线阅读。
*
*
* @author w.vela
*/
public class WeightFailover implements Failover, Closeable {
private static final Logger logger = getLogger(WeightFailover.class);
private final IntUnaryOperator failReduceWeight;
private final IntUnaryOperator successIncreaseWeight;
private final ConcurrentMap initWeightMap;
private final ConcurrentMap currentWeightMap;
@SuppressWarnings("checkstyle:VisibilityModifier")
final CloseableSupplier> recoveryFuture;
private final Consumer onMinWeight;
private final int minWeight;
/**
* {@code null} if this feature is off.
*/
private final Integer weightOnMissingNode;
/**
* 用于实现基于上下文的重试过滤逻辑
*/
@Nullable
private final Predicate filter;
@SuppressWarnings("checkstyle:VisibilityModifier")
AtomicBoolean closed = new AtomicBoolean(false);
private AtomicInteger allAvailableVersion = new AtomicInteger();
@SuppressWarnings({"checkstyle:VisibilityModifier"})
private static class AllAvailable {
int version;
List allAvailable;
}
private volatile AllAvailable allAvailable;
WeightFailover(WeightFailoverBuilder builder) {
this.minWeight = builder.minWeight;
this.failReduceWeight = builder.failReduceWeight;
this.successIncreaseWeight = builder.successIncreaseWeight;
this.initWeightMap = new ConcurrentHashMap<>(builder.initWeightMap);
this.currentWeightMap = new ConcurrentHashMap<>(builder.initWeightMap);
this.onMinWeight = builder.onMinWeight;
this.weightOnMissingNode = builder.weightOnMissingNode;
this.filter = builder.filter;
this.allAvailable = new AllAvailable<>();
this.allAvailable.allAvailable = ImmutableList.copyOf(builder.initWeightMap.keySet());
this.allAvailable.version = allAvailableVersion.get();
WeightFailoverCheckTask t = new WeightFailoverCheckTask<>(this, builder, closed,
initWeightMap, currentWeightMap, allAvailableVersion);
this.recoveryFuture = t.lazyFuture();
}
/**
* better use {@link #newGenericBuilder()} for type safe
*/
@Deprecated
public static WeightFailoverBuilder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy