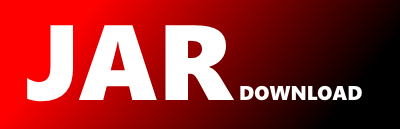
com.github.phantomthief.zookeeper.GenericZkBasedNodeBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zkconfig-resources Show documentation
Show all versions of zkconfig-resources Show documentation
A ZooKeeper based configuration resources holder
The newest version!
package com.github.phantomthief.zookeeper;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.apache.curator.framework.CuratorFramework;
import org.apache.curator.framework.recipes.cache.ChildData;
import org.apache.curator.framework.recipes.cache.NodeCache;
import org.apache.zookeeper.data.Stat;
import com.github.phantomthief.util.ThrowableBiConsumer;
import com.github.phantomthief.util.ThrowableBiFunction;
import com.github.phantomthief.util.ThrowableConsumer;
import com.github.phantomthief.util.ThrowableFunction;
import com.github.phantomthief.zookeeper.ZkBasedNodeResource.Builder;
import com.google.common.util.concurrent.ListenableFuture;
import com.google.common.util.concurrent.ListeningExecutorService;
/**
* @author w.vela
* Created on 16/5/13.
*/
public class GenericZkBasedNodeBuilder {
private final Builder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy