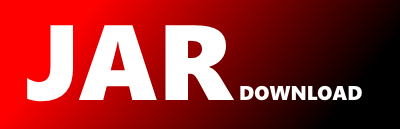
dds.Dds Maven / Gradle / Ivy
// Generated by jextract
package dds;
import java.lang.invoke.MethodHandle;
import java.lang.invoke.VarHandle;
import java.nio.ByteOrder;
import java.lang.foreign.*;
import static java.lang.foreign.ValueLayout.*;
public class Dds {
public static final OfByte C_CHAR = JAVA_BYTE;
public static final OfShort C_SHORT = JAVA_SHORT;
public static final OfInt C_INT = JAVA_INT;
public static final OfLong C_LONG = JAVA_LONG;
public static final OfLong C_LONG_LONG = JAVA_LONG;
public static final OfFloat C_FLOAT = JAVA_FLOAT;
public static final OfDouble C_DOUBLE = JAVA_DOUBLE;
public static final AddressLayout C_POINTER = RuntimeHelper.POINTER;
/**
* {@snippet :
* #define true 1
* }
*/
public static int true_() {
return (int)1L;
}
/**
* {@snippet :
* #define false 0
* }
*/
public static int false_() {
return (int)0L;
}
/**
* {@snippet :
* #define __bool_true_false_are_defined 1
* }
*/
public static int __bool_true_false_are_defined() {
return (int)1L;
}
/**
* {@snippet :
* #define DDS_VERSION 20900
* }
*/
public static int DDS_VERSION() {
return (int)20900L;
}
/**
* {@snippet :
* #define DDS_HANDS 4
* }
*/
public static int DDS_HANDS() {
return (int)4L;
}
/**
* {@snippet :
* #define DDS_SUITS 4
* }
*/
public static int DDS_SUITS() {
return (int)4L;
}
/**
* {@snippet :
* #define DDS_STRAINS 5
* }
*/
public static int DDS_STRAINS() {
return (int)5L;
}
/**
* {@snippet :
* #define MAXNOOFBOARDS 200
* }
*/
public static int MAXNOOFBOARDS() {
return (int)200L;
}
/**
* {@snippet :
* #define MAXNOOFTABLES 40
* }
*/
public static int MAXNOOFTABLES() {
return (int)40L;
}
/**
* {@snippet :
* #define RETURN_NO_FAULT 1
* }
*/
public static int RETURN_NO_FAULT() {
return (int)1L;
}
public static MethodHandle SetMaxThreads$MH() {
return RuntimeHelper.requireNonNull(constants$10.const$4,"SetMaxThreads");
}
/**
* {@snippet :
* void SetMaxThreads(int userThreads);
* }
*/
public static void SetMaxThreads(int userThreads) {
var mh$ = SetMaxThreads$MH();
try {
mh$.invokeExact(userThreads);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SetThreading$MH() {
return RuntimeHelper.requireNonNull(constants$10.const$6,"SetThreading");
}
/**
* {@snippet :
* int SetThreading(int code);
* }
*/
public static int SetThreading(int code) {
var mh$ = SetThreading$MH();
try {
return (int)mh$.invokeExact(code);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SetResources$MH() {
return RuntimeHelper.requireNonNull(constants$11.const$1,"SetResources");
}
/**
* {@snippet :
* void SetResources(int maxMemoryMB, int maxThreads);
* }
*/
public static void SetResources(int maxMemoryMB, int maxThreads) {
var mh$ = SetResources$MH();
try {
mh$.invokeExact(maxMemoryMB, maxThreads);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle FreeMemory$MH() {
return RuntimeHelper.requireNonNull(constants$11.const$3,"FreeMemory");
}
/**
* {@snippet :
* void FreeMemory(,...);
* }
*/
public static void FreeMemory(Object... x0) {
var mh$ = FreeMemory$MH();
try {
mh$.invokeExact(x0);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SolveBoard$MH() {
return RuntimeHelper.requireNonNull(constants$11.const$5,"SolveBoard");
}
/**
* {@snippet :
* int SolveBoard(struct deal dl, int target, int solutions, int mode, struct futureTricks* futp, int threadIndex);
* }
*/
public static int SolveBoard(MemorySegment dl, int target, int solutions, int mode, MemorySegment futp, int threadIndex) {
var mh$ = SolveBoard$MH();
try {
return (int)mh$.invokeExact(dl, target, solutions, mode, futp, threadIndex);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SolveBoardPBN$MH() {
return RuntimeHelper.requireNonNull(constants$12.const$1,"SolveBoardPBN");
}
/**
* {@snippet :
* int SolveBoardPBN(struct dealPBN dlpbn, int target, int solutions, int mode, struct futureTricks* futp, int thrId);
* }
*/
public static int SolveBoardPBN(MemorySegment dlpbn, int target, int solutions, int mode, MemorySegment futp, int thrId) {
var mh$ = SolveBoardPBN$MH();
try {
return (int)mh$.invokeExact(dlpbn, target, solutions, mode, futp, thrId);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle CalcDDtable$MH() {
return RuntimeHelper.requireNonNull(constants$12.const$3,"CalcDDtable");
}
/**
* {@snippet :
* int CalcDDtable(struct ddTableDeal tableDeal, struct ddTableResults* tablep);
* }
*/
public static int CalcDDtable(MemorySegment tableDeal, MemorySegment tablep) {
var mh$ = CalcDDtable$MH();
try {
return (int)mh$.invokeExact(tableDeal, tablep);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle CalcDDtablePBN$MH() {
return RuntimeHelper.requireNonNull(constants$12.const$5,"CalcDDtablePBN");
}
/**
* {@snippet :
* int CalcDDtablePBN(struct ddTableDealPBN tableDealPBN, struct ddTableResults* tablep);
* }
*/
public static int CalcDDtablePBN(MemorySegment tableDealPBN, MemorySegment tablep) {
var mh$ = CalcDDtablePBN$MH();
try {
return (int)mh$.invokeExact(tableDealPBN, tablep);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle CalcAllTables$MH() {
return RuntimeHelper.requireNonNull(constants$13.const$1,"CalcAllTables");
}
/**
* {@snippet :
* int CalcAllTables(struct ddTableDeals* dealsp, int mode, int trumpFilter[5], struct ddTablesRes* resp, struct allParResults* presp);
* }
*/
public static int CalcAllTables(MemorySegment dealsp, int mode, MemorySegment trumpFilter, MemorySegment resp, MemorySegment presp) {
var mh$ = CalcAllTables$MH();
try {
return (int)mh$.invokeExact(dealsp, mode, trumpFilter, resp, presp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle CalcAllTablesPBN$MH() {
return RuntimeHelper.requireNonNull(constants$13.const$2,"CalcAllTablesPBN");
}
/**
* {@snippet :
* int CalcAllTablesPBN(struct ddTableDealsPBN* dealsp, int mode, int trumpFilter[5], struct ddTablesRes* resp, struct allParResults* presp);
* }
*/
public static int CalcAllTablesPBN(MemorySegment dealsp, int mode, MemorySegment trumpFilter, MemorySegment resp, MemorySegment presp) {
var mh$ = CalcAllTablesPBN$MH();
try {
return (int)mh$.invokeExact(dealsp, mode, trumpFilter, resp, presp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SolveAllBoards$MH() {
return RuntimeHelper.requireNonNull(constants$13.const$4,"SolveAllBoards");
}
/**
* {@snippet :
* int SolveAllBoards(struct boardsPBN* bop, struct solvedBoards* solvedp);
* }
*/
public static int SolveAllBoards(MemorySegment bop, MemorySegment solvedp) {
var mh$ = SolveAllBoards$MH();
try {
return (int)mh$.invokeExact(bop, solvedp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SolveAllBoardsBin$MH() {
return RuntimeHelper.requireNonNull(constants$13.const$5,"SolveAllBoardsBin");
}
/**
* {@snippet :
* int SolveAllBoardsBin(struct boards* bop, struct solvedBoards* solvedp);
* }
*/
public static int SolveAllBoardsBin(MemorySegment bop, MemorySegment solvedp) {
var mh$ = SolveAllBoardsBin$MH();
try {
return (int)mh$.invokeExact(bop, solvedp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SolveAllChunks$MH() {
return RuntimeHelper.requireNonNull(constants$14.const$1,"SolveAllChunks");
}
/**
* {@snippet :
* int SolveAllChunks(struct boardsPBN* bop, struct solvedBoards* solvedp, int chunkSize);
* }
*/
public static int SolveAllChunks(MemorySegment bop, MemorySegment solvedp, int chunkSize) {
var mh$ = SolveAllChunks$MH();
try {
return (int)mh$.invokeExact(bop, solvedp, chunkSize);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SolveAllChunksBin$MH() {
return RuntimeHelper.requireNonNull(constants$14.const$2,"SolveAllChunksBin");
}
/**
* {@snippet :
* int SolveAllChunksBin(struct boards* bop, struct solvedBoards* solvedp, int chunkSize);
* }
*/
public static int SolveAllChunksBin(MemorySegment bop, MemorySegment solvedp, int chunkSize) {
var mh$ = SolveAllChunksBin$MH();
try {
return (int)mh$.invokeExact(bop, solvedp, chunkSize);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SolveAllChunksPBN$MH() {
return RuntimeHelper.requireNonNull(constants$14.const$3,"SolveAllChunksPBN");
}
/**
* {@snippet :
* int SolveAllChunksPBN(struct boardsPBN* bop, struct solvedBoards* solvedp, int chunkSize);
* }
*/
public static int SolveAllChunksPBN(MemorySegment bop, MemorySegment solvedp, int chunkSize) {
var mh$ = SolveAllChunksPBN$MH();
try {
return (int)mh$.invokeExact(bop, solvedp, chunkSize);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle Par$MH() {
return RuntimeHelper.requireNonNull(constants$14.const$4,"Par");
}
/**
* {@snippet :
* int Par(struct ddTableResults* tablep, struct parResults* presp, int vulnerable);
* }
*/
public static int Par(MemorySegment tablep, MemorySegment presp, int vulnerable) {
var mh$ = Par$MH();
try {
return (int)mh$.invokeExact(tablep, presp, vulnerable);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle CalcPar$MH() {
return RuntimeHelper.requireNonNull(constants$14.const$6,"CalcPar");
}
/**
* {@snippet :
* int CalcPar(struct ddTableDeal tableDeal, int vulnerable, struct ddTableResults* tablep, struct parResults* presp);
* }
*/
public static int CalcPar(MemorySegment tableDeal, int vulnerable, MemorySegment tablep, MemorySegment presp) {
var mh$ = CalcPar$MH();
try {
return (int)mh$.invokeExact(tableDeal, vulnerable, tablep, presp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle CalcParPBN$MH() {
return RuntimeHelper.requireNonNull(constants$15.const$1,"CalcParPBN");
}
/**
* {@snippet :
* int CalcParPBN(struct ddTableDealPBN tableDealPBN, struct ddTableResults* tablep, int vulnerable, struct parResults* presp);
* }
*/
public static int CalcParPBN(MemorySegment tableDealPBN, MemorySegment tablep, int vulnerable, MemorySegment presp) {
var mh$ = CalcParPBN$MH();
try {
return (int)mh$.invokeExact(tableDealPBN, tablep, vulnerable, presp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SidesPar$MH() {
return RuntimeHelper.requireNonNull(constants$15.const$2,"SidesPar");
}
/**
* {@snippet :
* int SidesPar(struct ddTableResults* tablep, struct parResultsDealer sidesRes[2], int vulnerable);
* }
*/
public static int SidesPar(MemorySegment tablep, MemorySegment sidesRes, int vulnerable) {
var mh$ = SidesPar$MH();
try {
return (int)mh$.invokeExact(tablep, sidesRes, vulnerable);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle DealerPar$MH() {
return RuntimeHelper.requireNonNull(constants$15.const$4,"DealerPar");
}
/**
* {@snippet :
* int DealerPar(struct ddTableResults* tablep, struct parResultsDealer* presp, int dealer, int vulnerable);
* }
*/
public static int DealerPar(MemorySegment tablep, MemorySegment presp, int dealer, int vulnerable) {
var mh$ = DealerPar$MH();
try {
return (int)mh$.invokeExact(tablep, presp, dealer, vulnerable);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle DealerParBin$MH() {
return RuntimeHelper.requireNonNull(constants$15.const$5,"DealerParBin");
}
/**
* {@snippet :
* int DealerParBin(struct ddTableResults* tablep, struct parResultsMaster* presp, int dealer, int vulnerable);
* }
*/
public static int DealerParBin(MemorySegment tablep, MemorySegment presp, int dealer, int vulnerable) {
var mh$ = DealerParBin$MH();
try {
return (int)mh$.invokeExact(tablep, presp, dealer, vulnerable);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle SidesParBin$MH() {
return RuntimeHelper.requireNonNull(constants$16.const$0,"SidesParBin");
}
/**
* {@snippet :
* int SidesParBin(struct ddTableResults* tablep, struct parResultsMaster sidesRes[2], int vulnerable);
* }
*/
public static int SidesParBin(MemorySegment tablep, MemorySegment sidesRes, int vulnerable) {
var mh$ = SidesParBin$MH();
try {
return (int)mh$.invokeExact(tablep, sidesRes, vulnerable);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle ConvertToDealerTextFormat$MH() {
return RuntimeHelper.requireNonNull(constants$16.const$1,"ConvertToDealerTextFormat");
}
/**
* {@snippet :
* int ConvertToDealerTextFormat(struct parResultsMaster* pres, char* resp);
* }
*/
public static int ConvertToDealerTextFormat(MemorySegment pres, MemorySegment resp) {
var mh$ = ConvertToDealerTextFormat$MH();
try {
return (int)mh$.invokeExact(pres, resp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle ConvertToSidesTextFormat$MH() {
return RuntimeHelper.requireNonNull(constants$16.const$2,"ConvertToSidesTextFormat");
}
/**
* {@snippet :
* int ConvertToSidesTextFormat(struct parResultsMaster* pres, struct parTextResults* resp);
* }
*/
public static int ConvertToSidesTextFormat(MemorySegment pres, MemorySegment resp) {
var mh$ = ConvertToSidesTextFormat$MH();
try {
return (int)mh$.invokeExact(pres, resp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle AnalysePlayBin$MH() {
return RuntimeHelper.requireNonNull(constants$16.const$4,"AnalysePlayBin");
}
/**
* {@snippet :
* int AnalysePlayBin(struct deal dl, struct playTraceBin play, struct solvedPlay* solved, int thrId);
* }
*/
public static int AnalysePlayBin(MemorySegment dl, MemorySegment play, MemorySegment solved, int thrId) {
var mh$ = AnalysePlayBin$MH();
try {
return (int)mh$.invokeExact(dl, play, solved, thrId);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle AnalysePlayPBN$MH() {
return RuntimeHelper.requireNonNull(constants$16.const$6,"AnalysePlayPBN");
}
/**
* {@snippet :
* int AnalysePlayPBN(struct dealPBN dlPBN, struct playTracePBN playPBN, struct solvedPlay* solvedp, int thrId);
* }
*/
public static int AnalysePlayPBN(MemorySegment dlPBN, MemorySegment playPBN, MemorySegment solvedp, int thrId) {
var mh$ = AnalysePlayPBN$MH();
try {
return (int)mh$.invokeExact(dlPBN, playPBN, solvedp, thrId);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle AnalyseAllPlaysBin$MH() {
return RuntimeHelper.requireNonNull(constants$17.const$1,"AnalyseAllPlaysBin");
}
/**
* {@snippet :
* int AnalyseAllPlaysBin(struct boards* bop, struct playTracesBin* plp, struct solvedPlays* solvedp, int chunkSize);
* }
*/
public static int AnalyseAllPlaysBin(MemorySegment bop, MemorySegment plp, MemorySegment solvedp, int chunkSize) {
var mh$ = AnalyseAllPlaysBin$MH();
try {
return (int)mh$.invokeExact(bop, plp, solvedp, chunkSize);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle AnalyseAllPlaysPBN$MH() {
return RuntimeHelper.requireNonNull(constants$17.const$2,"AnalyseAllPlaysPBN");
}
/**
* {@snippet :
* int AnalyseAllPlaysPBN(struct boardsPBN* bopPBN, struct playTracesPBN* plpPBN, struct solvedPlays* solvedp, int chunkSize);
* }
*/
public static int AnalyseAllPlaysPBN(MemorySegment bopPBN, MemorySegment plpPBN, MemorySegment solvedp, int chunkSize) {
var mh$ = AnalyseAllPlaysPBN$MH();
try {
return (int)mh$.invokeExact(bopPBN, plpPBN, solvedp, chunkSize);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle GetDDSInfo$MH() {
return RuntimeHelper.requireNonNull(constants$17.const$4,"GetDDSInfo");
}
/**
* {@snippet :
* void GetDDSInfo(struct DDSInfo* info);
* }
*/
public static void GetDDSInfo(MemorySegment info) {
var mh$ = GetDDSInfo$MH();
try {
mh$.invokeExact(info);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
public static MethodHandle ErrorMessage$MH() {
return RuntimeHelper.requireNonNull(constants$17.const$6,"ErrorMessage");
}
/**
* {@snippet :
* void ErrorMessage(int code, char line[80]);
* }
*/
public static void ErrorMessage(int code, MemorySegment line) {
var mh$ = ErrorMessage$MH();
try {
mh$.invokeExact(code, line);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
/**
* {@snippet :
* #define TEXT_NO_FAULT "Success"
* }
*/
public static MemorySegment TEXT_NO_FAULT() {
return constants$18.const$0;
}
/**
* {@snippet :
* #define RETURN_UNKNOWN_FAULT -1
* }
*/
public static int RETURN_UNKNOWN_FAULT() {
return (int)-1L;
}
/**
* {@snippet :
* #define TEXT_UNKNOWN_FAULT "General error"
* }
*/
public static MemorySegment TEXT_UNKNOWN_FAULT() {
return constants$18.const$1;
}
/**
* {@snippet :
* #define RETURN_ZERO_CARDS -2
* }
*/
public static int RETURN_ZERO_CARDS() {
return (int)-2L;
}
/**
* {@snippet :
* #define TEXT_ZERO_CARDS "Zero cards"
* }
*/
public static MemorySegment TEXT_ZERO_CARDS() {
return constants$18.const$2;
}
/**
* {@snippet :
* #define RETURN_TARGET_TOO_HIGH -3
* }
*/
public static int RETURN_TARGET_TOO_HIGH() {
return (int)-3L;
}
/**
* {@snippet :
* #define TEXT_TARGET_TOO_HIGH "Target exceeds number of tricks"
* }
*/
public static MemorySegment TEXT_TARGET_TOO_HIGH() {
return constants$18.const$3;
}
/**
* {@snippet :
* #define RETURN_DUPLICATE_CARDS -4
* }
*/
public static int RETURN_DUPLICATE_CARDS() {
return (int)-4L;
}
/**
* {@snippet :
* #define TEXT_DUPLICATE_CARDS "Cards duplicated"
* }
*/
public static MemorySegment TEXT_DUPLICATE_CARDS() {
return constants$18.const$4;
}
/**
* {@snippet :
* #define RETURN_TARGET_WRONG_LO -5
* }
*/
public static int RETURN_TARGET_WRONG_LO() {
return (int)-5L;
}
/**
* {@snippet :
* #define TEXT_TARGET_WRONG_LO "Target is less than -1"
* }
*/
public static MemorySegment TEXT_TARGET_WRONG_LO() {
return constants$18.const$5;
}
/**
* {@snippet :
* #define RETURN_TARGET_WRONG_HI -7
* }
*/
public static int RETURN_TARGET_WRONG_HI() {
return (int)-7L;
}
/**
* {@snippet :
* #define TEXT_TARGET_WRONG_HI "Target is higher than 13"
* }
*/
public static MemorySegment TEXT_TARGET_WRONG_HI() {
return constants$19.const$0;
}
/**
* {@snippet :
* #define RETURN_SOLNS_WRONG_LO -8
* }
*/
public static int RETURN_SOLNS_WRONG_LO() {
return (int)-8L;
}
/**
* {@snippet :
* #define TEXT_SOLNS_WRONG_LO "Solutions parameter is less than 1"
* }
*/
public static MemorySegment TEXT_SOLNS_WRONG_LO() {
return constants$19.const$1;
}
/**
* {@snippet :
* #define RETURN_SOLNS_WRONG_HI -9
* }
*/
public static int RETURN_SOLNS_WRONG_HI() {
return (int)-9L;
}
/**
* {@snippet :
* #define TEXT_SOLNS_WRONG_HI "Solutions parameter is higher than 3"
* }
*/
public static MemorySegment TEXT_SOLNS_WRONG_HI() {
return constants$19.const$2;
}
/**
* {@snippet :
* #define RETURN_TOO_MANY_CARDS -10
* }
*/
public static int RETURN_TOO_MANY_CARDS() {
return (int)-10L;
}
/**
* {@snippet :
* #define TEXT_TOO_MANY_CARDS "Too many cards"
* }
*/
public static MemorySegment TEXT_TOO_MANY_CARDS() {
return constants$19.const$3;
}
/**
* {@snippet :
* #define RETURN_SUIT_OR_RANK -12
* }
*/
public static int RETURN_SUIT_OR_RANK() {
return (int)-12L;
}
/**
* {@snippet :
* #define TEXT_SUIT_OR_RANK "currentTrickSuit or currentTrickRank has wrong data"
* }
*/
public static MemorySegment TEXT_SUIT_OR_RANK() {
return constants$19.const$4;
}
/**
* {@snippet :
* #define RETURN_PLAYED_CARD -13
* }
*/
public static int RETURN_PLAYED_CARD() {
return (int)-13L;
}
/**
* {@snippet :
* #define TEXT_PLAYED_CARD "Played card also remains in a hand"
* }
*/
public static MemorySegment TEXT_PLAYED_CARD() {
return constants$19.const$5;
}
/**
* {@snippet :
* #define RETURN_CARD_COUNT -14
* }
*/
public static int RETURN_CARD_COUNT() {
return (int)-14L;
}
/**
* {@snippet :
* #define TEXT_CARD_COUNT "Wrong number of remaining cards in a hand"
* }
*/
public static MemorySegment TEXT_CARD_COUNT() {
return constants$20.const$0;
}
/**
* {@snippet :
* #define RETURN_THREAD_INDEX -15
* }
*/
public static int RETURN_THREAD_INDEX() {
return (int)-15L;
}
/**
* {@snippet :
* #define TEXT_THREAD_INDEX "Thread index is not 0 .. maximum"
* }
*/
public static MemorySegment TEXT_THREAD_INDEX() {
return constants$20.const$1;
}
/**
* {@snippet :
* #define RETURN_MODE_WRONG_LO -16
* }
*/
public static int RETURN_MODE_WRONG_LO() {
return (int)-16L;
}
/**
* {@snippet :
* #define TEXT_MODE_WRONG_LO "Mode parameter is less than 0"
* }
*/
public static MemorySegment TEXT_MODE_WRONG_LO() {
return constants$20.const$2;
}
/**
* {@snippet :
* #define RETURN_MODE_WRONG_HI -17
* }
*/
public static int RETURN_MODE_WRONG_HI() {
return (int)-17L;
}
/**
* {@snippet :
* #define TEXT_MODE_WRONG_HI "Mode parameter is higher than 2"
* }
*/
public static MemorySegment TEXT_MODE_WRONG_HI() {
return constants$20.const$3;
}
/**
* {@snippet :
* #define RETURN_TRUMP_WRONG -18
* }
*/
public static int RETURN_TRUMP_WRONG() {
return (int)-18L;
}
/**
* {@snippet :
* #define TEXT_TRUMP_WRONG "Trump is not in 0 .. 4"
* }
*/
public static MemorySegment TEXT_TRUMP_WRONG() {
return constants$20.const$4;
}
/**
* {@snippet :
* #define RETURN_FIRST_WRONG -19
* }
*/
public static int RETURN_FIRST_WRONG() {
return (int)-19L;
}
/**
* {@snippet :
* #define TEXT_FIRST_WRONG "First is not in 0 .. 2"
* }
*/
public static MemorySegment TEXT_FIRST_WRONG() {
return constants$20.const$5;
}
/**
* {@snippet :
* #define RETURN_PLAY_FAULT -98
* }
*/
public static int RETURN_PLAY_FAULT() {
return (int)-98L;
}
/**
* {@snippet :
* #define TEXT_PLAY_FAULT "AnalysePlay input error"
* }
*/
public static MemorySegment TEXT_PLAY_FAULT() {
return constants$21.const$0;
}
/**
* {@snippet :
* #define RETURN_PBN_FAULT -99
* }
*/
public static int RETURN_PBN_FAULT() {
return (int)-99L;
}
/**
* {@snippet :
* #define TEXT_PBN_FAULT "PBN string error"
* }
*/
public static MemorySegment TEXT_PBN_FAULT() {
return constants$21.const$1;
}
/**
* {@snippet :
* #define RETURN_TOO_MANY_BOARDS -101
* }
*/
public static int RETURN_TOO_MANY_BOARDS() {
return (int)-101L;
}
/**
* {@snippet :
* #define TEXT_TOO_MANY_BOARDS "Too many boards requested"
* }
*/
public static MemorySegment TEXT_TOO_MANY_BOARDS() {
return constants$21.const$2;
}
/**
* {@snippet :
* #define RETURN_THREAD_CREATE -102
* }
*/
public static int RETURN_THREAD_CREATE() {
return (int)-102L;
}
/**
* {@snippet :
* #define TEXT_THREAD_CREATE "Could not create threads"
* }
*/
public static MemorySegment TEXT_THREAD_CREATE() {
return constants$21.const$3;
}
/**
* {@snippet :
* #define RETURN_THREAD_WAIT -103
* }
*/
public static int RETURN_THREAD_WAIT() {
return (int)-103L;
}
/**
* {@snippet :
* #define TEXT_THREAD_WAIT "Something failed waiting for thread to end"
* }
*/
public static MemorySegment TEXT_THREAD_WAIT() {
return constants$21.const$4;
}
/**
* {@snippet :
* #define RETURN_THREAD_MISSING -104
* }
*/
public static int RETURN_THREAD_MISSING() {
return (int)-104L;
}
/**
* {@snippet :
* #define TEXT_THREAD_MISSING "Multi-threading system not present"
* }
*/
public static MemorySegment TEXT_THREAD_MISSING() {
return constants$21.const$5;
}
/**
* {@snippet :
* #define RETURN_NO_SUIT -201
* }
*/
public static int RETURN_NO_SUIT() {
return (int)-201L;
}
/**
* {@snippet :
* #define TEXT_NO_SUIT "Denomination filter vector has no entries"
* }
*/
public static MemorySegment TEXT_NO_SUIT() {
return constants$22.const$0;
}
/**
* {@snippet :
* #define RETURN_TOO_MANY_TABLES -202
* }
*/
public static int RETURN_TOO_MANY_TABLES() {
return (int)-202L;
}
/**
* {@snippet :
* #define TEXT_TOO_MANY_TABLES "Too many DD tables requested"
* }
*/
public static MemorySegment TEXT_TOO_MANY_TABLES() {
return constants$22.const$1;
}
/**
* {@snippet :
* #define RETURN_CHUNK_SIZE -301
* }
*/
public static int RETURN_CHUNK_SIZE() {
return (int)-301L;
}
/**
* {@snippet :
* #define TEXT_CHUNK_SIZE "Chunk size is less than 1"
* }
*/
public static MemorySegment TEXT_CHUNK_SIZE() {
return constants$22.const$2;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy