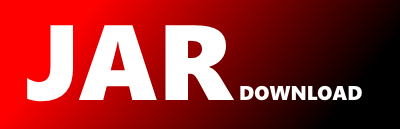
dds.Dds Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dds4j Show documentation
Show all versions of dds4j Show documentation
Wrapper around the Double Dummy Solver C++ Library.
The newest version!
// Generated by jextract
package dds;
import java.lang.invoke.*;
import java.lang.foreign.*;
import java.nio.ByteOrder;
import java.util.*;
import java.util.function.*;
import java.util.stream.*;
import static java.lang.foreign.ValueLayout.*;
import static java.lang.foreign.MemoryLayout.PathElement.*;
public class Dds {
Dds() {
// Should not be called directly
}
static final Arena LIBRARY_ARENA = Arena.ofAuto();
static final boolean TRACE_DOWNCALLS = Boolean.getBoolean("jextract.trace.downcalls");
static void traceDowncall(String name, Object... args) {
String traceArgs = Arrays.stream(args)
.map(Object::toString)
.collect(Collectors.joining(", "));
System.out.printf("%s(%s)\n", name, traceArgs);
}
static MemorySegment findOrThrow(String symbol) {
return SYMBOL_LOOKUP.find(symbol)
.orElseThrow(() -> new UnsatisfiedLinkError("unresolved symbol: " + symbol));
}
static MethodHandle upcallHandle(Class> fi, String name, FunctionDescriptor fdesc) {
try {
return MethodHandles.lookup().findVirtual(fi, name, fdesc.toMethodType());
} catch (ReflectiveOperationException ex) {
throw new AssertionError(ex);
}
}
static MemoryLayout align(MemoryLayout layout, long align) {
return switch (layout) {
case PaddingLayout p -> p;
case ValueLayout v -> v.withByteAlignment(align);
case GroupLayout g -> {
MemoryLayout[] alignedMembers = g.memberLayouts().stream()
.map(m -> align(m, align)).toArray(MemoryLayout[]::new);
yield g instanceof StructLayout ?
MemoryLayout.structLayout(alignedMembers) : MemoryLayout.unionLayout(alignedMembers);
}
case SequenceLayout s -> MemoryLayout.sequenceLayout(s.elementCount(), align(s.elementLayout(), align));
};
}
static final SymbolLookup SYMBOL_LOOKUP = SymbolLookup.loaderLookup()
.or(Linker.nativeLinker().defaultLookup());
public static final ValueLayout.OfBoolean C_BOOL = ValueLayout.JAVA_BOOLEAN;
public static final ValueLayout.OfByte C_CHAR = ValueLayout.JAVA_BYTE;
public static final ValueLayout.OfShort C_SHORT = ValueLayout.JAVA_SHORT;
public static final ValueLayout.OfInt C_INT = ValueLayout.JAVA_INT;
public static final ValueLayout.OfLong C_LONG_LONG = ValueLayout.JAVA_LONG;
public static final ValueLayout.OfFloat C_FLOAT = ValueLayout.JAVA_FLOAT;
public static final ValueLayout.OfDouble C_DOUBLE = ValueLayout.JAVA_DOUBLE;
public static final AddressLayout C_POINTER = ValueLayout.ADDRESS
.withTargetLayout(MemoryLayout.sequenceLayout(java.lang.Long.MAX_VALUE, JAVA_BYTE));
public static final ValueLayout.OfLong C_LONG = ValueLayout.JAVA_LONG;
private static class SetMaxThreads {
public static final FunctionDescriptor DESC = FunctionDescriptor.ofVoid(
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SetMaxThreads"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* void SetMaxThreads(int userThreads)
* }
*/
public static FunctionDescriptor SetMaxThreads$descriptor() {
return SetMaxThreads.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* void SetMaxThreads(int userThreads)
* }
*/
public static MethodHandle SetMaxThreads$handle() {
return SetMaxThreads.HANDLE;
}
/**
* {@snippet lang=c :
* void SetMaxThreads(int userThreads)
* }
*/
public static void SetMaxThreads(int userThreads) {
var mh$ = SetMaxThreads.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SetMaxThreads", userThreads);
}
mh$.invokeExact(userThreads);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class SetThreading {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SetThreading"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int SetThreading(int code)
* }
*/
public static FunctionDescriptor SetThreading$descriptor() {
return SetThreading.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int SetThreading(int code)
* }
*/
public static MethodHandle SetThreading$handle() {
return SetThreading.HANDLE;
}
/**
* {@snippet lang=c :
* int SetThreading(int code)
* }
*/
public static int SetThreading(int code) {
var mh$ = SetThreading.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SetThreading", code);
}
return (int)mh$.invokeExact(code);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class SetResources {
public static final FunctionDescriptor DESC = FunctionDescriptor.ofVoid(
Dds.C_INT,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SetResources"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* void SetResources(int maxMemoryMB, int maxThreads)
* }
*/
public static FunctionDescriptor SetResources$descriptor() {
return SetResources.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* void SetResources(int maxMemoryMB, int maxThreads)
* }
*/
public static MethodHandle SetResources$handle() {
return SetResources.HANDLE;
}
/**
* {@snippet lang=c :
* void SetResources(int maxMemoryMB, int maxThreads)
* }
*/
public static void SetResources(int maxMemoryMB, int maxThreads) {
var mh$ = SetResources.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SetResources", maxMemoryMB, maxThreads);
}
mh$.invokeExact(maxMemoryMB, maxThreads);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
/**
* Variadic invoker class for:
* {@snippet lang=c :
* void FreeMemory()
* }
*/
public static class FreeMemory {
private static final FunctionDescriptor BASE_DESC = FunctionDescriptor.ofVoid( );
private static final MemorySegment ADDR = Dds.findOrThrow("FreeMemory");
private final MethodHandle handle;
private final FunctionDescriptor descriptor;
private final MethodHandle spreader;
private FreeMemory(MethodHandle handle, FunctionDescriptor descriptor, MethodHandle spreader) {
this.handle = handle;
this.descriptor = descriptor;
this.spreader = spreader;
}
/**
* Variadic invoker factory for:
* {@snippet lang=c :
* void FreeMemory()
* }
*/
public static FreeMemory makeInvoker(MemoryLayout... layouts) {
FunctionDescriptor desc$ = BASE_DESC.appendArgumentLayouts(layouts);
Linker.Option fva$ = Linker.Option.firstVariadicArg(BASE_DESC.argumentLayouts().size());
var mh$ = Linker.nativeLinker().downcallHandle(ADDR, desc$, fva$);
var spreader$ = mh$.asSpreader(Object[].class, layouts.length);
return new FreeMemory(mh$, desc$, spreader$);
}
/**
* {@return the specialized method handle}
*/
public MethodHandle handle() {
return handle;
}
/**
* {@return the specialized descriptor}
*/
public FunctionDescriptor descriptor() {
return descriptor;
}
public void apply(Object... x0) {
try {
if (TRACE_DOWNCALLS) {
traceDowncall("FreeMemory", x0);
}
spreader.invokeExact(x0);
} catch(IllegalArgumentException | ClassCastException ex$) {
throw ex$; // rethrow IAE from passing wrong number/type of args
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static class SolveBoard {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
deal.layout(),
Dds.C_INT,
Dds.C_INT,
Dds.C_INT,
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SolveBoard"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int SolveBoard(struct deal dl, int target, int solutions, int mode, struct futureTricks *futp, int threadIndex)
* }
*/
public static FunctionDescriptor SolveBoard$descriptor() {
return SolveBoard.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int SolveBoard(struct deal dl, int target, int solutions, int mode, struct futureTricks *futp, int threadIndex)
* }
*/
public static MethodHandle SolveBoard$handle() {
return SolveBoard.HANDLE;
}
/**
* {@snippet lang=c :
* int SolveBoard(struct deal dl, int target, int solutions, int mode, struct futureTricks *futp, int threadIndex)
* }
*/
public static int SolveBoard(MemorySegment dl, int target, int solutions, int mode, MemorySegment futp, int threadIndex) {
var mh$ = SolveBoard.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SolveBoard", dl, target, solutions, mode, futp, threadIndex);
}
return (int)mh$.invokeExact(dl, target, solutions, mode, futp, threadIndex);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class SolveBoardPBN {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
dealPBN.layout(),
Dds.C_INT,
Dds.C_INT,
Dds.C_INT,
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SolveBoardPBN"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int SolveBoardPBN(struct dealPBN dlpbn, int target, int solutions, int mode, struct futureTricks *futp, int thrId)
* }
*/
public static FunctionDescriptor SolveBoardPBN$descriptor() {
return SolveBoardPBN.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int SolveBoardPBN(struct dealPBN dlpbn, int target, int solutions, int mode, struct futureTricks *futp, int thrId)
* }
*/
public static MethodHandle SolveBoardPBN$handle() {
return SolveBoardPBN.HANDLE;
}
/**
* {@snippet lang=c :
* int SolveBoardPBN(struct dealPBN dlpbn, int target, int solutions, int mode, struct futureTricks *futp, int thrId)
* }
*/
public static int SolveBoardPBN(MemorySegment dlpbn, int target, int solutions, int mode, MemorySegment futp, int thrId) {
var mh$ = SolveBoardPBN.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SolveBoardPBN", dlpbn, target, solutions, mode, futp, thrId);
}
return (int)mh$.invokeExact(dlpbn, target, solutions, mode, futp, thrId);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class CalcDDtable {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
ddTableDeal.layout(),
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("CalcDDtable"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int CalcDDtable(struct ddTableDeal tableDeal, struct ddTableResults *tablep)
* }
*/
public static FunctionDescriptor CalcDDtable$descriptor() {
return CalcDDtable.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int CalcDDtable(struct ddTableDeal tableDeal, struct ddTableResults *tablep)
* }
*/
public static MethodHandle CalcDDtable$handle() {
return CalcDDtable.HANDLE;
}
/**
* {@snippet lang=c :
* int CalcDDtable(struct ddTableDeal tableDeal, struct ddTableResults *tablep)
* }
*/
public static int CalcDDtable(MemorySegment tableDeal, MemorySegment tablep) {
var mh$ = CalcDDtable.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("CalcDDtable", tableDeal, tablep);
}
return (int)mh$.invokeExact(tableDeal, tablep);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class CalcDDtablePBN {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
ddTableDealPBN.layout(),
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("CalcDDtablePBN"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int CalcDDtablePBN(struct ddTableDealPBN tableDealPBN, struct ddTableResults *tablep)
* }
*/
public static FunctionDescriptor CalcDDtablePBN$descriptor() {
return CalcDDtablePBN.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int CalcDDtablePBN(struct ddTableDealPBN tableDealPBN, struct ddTableResults *tablep)
* }
*/
public static MethodHandle CalcDDtablePBN$handle() {
return CalcDDtablePBN.HANDLE;
}
/**
* {@snippet lang=c :
* int CalcDDtablePBN(struct ddTableDealPBN tableDealPBN, struct ddTableResults *tablep)
* }
*/
public static int CalcDDtablePBN(MemorySegment tableDealPBN, MemorySegment tablep) {
var mh$ = CalcDDtablePBN.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("CalcDDtablePBN", tableDealPBN, tablep);
}
return (int)mh$.invokeExact(tableDealPBN, tablep);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class CalcAllTables {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("CalcAllTables"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int CalcAllTables(struct ddTableDeals *dealsp, int mode, int trumpFilter[5], struct ddTablesRes *resp, struct allParResults *presp)
* }
*/
public static FunctionDescriptor CalcAllTables$descriptor() {
return CalcAllTables.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int CalcAllTables(struct ddTableDeals *dealsp, int mode, int trumpFilter[5], struct ddTablesRes *resp, struct allParResults *presp)
* }
*/
public static MethodHandle CalcAllTables$handle() {
return CalcAllTables.HANDLE;
}
/**
* {@snippet lang=c :
* int CalcAllTables(struct ddTableDeals *dealsp, int mode, int trumpFilter[5], struct ddTablesRes *resp, struct allParResults *presp)
* }
*/
public static int CalcAllTables(MemorySegment dealsp, int mode, MemorySegment trumpFilter, MemorySegment resp, MemorySegment presp) {
var mh$ = CalcAllTables.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("CalcAllTables", dealsp, mode, trumpFilter, resp, presp);
}
return (int)mh$.invokeExact(dealsp, mode, trumpFilter, resp, presp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class CalcAllTablesPBN {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("CalcAllTablesPBN"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int CalcAllTablesPBN(struct ddTableDealsPBN *dealsp, int mode, int trumpFilter[5], struct ddTablesRes *resp, struct allParResults *presp)
* }
*/
public static FunctionDescriptor CalcAllTablesPBN$descriptor() {
return CalcAllTablesPBN.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int CalcAllTablesPBN(struct ddTableDealsPBN *dealsp, int mode, int trumpFilter[5], struct ddTablesRes *resp, struct allParResults *presp)
* }
*/
public static MethodHandle CalcAllTablesPBN$handle() {
return CalcAllTablesPBN.HANDLE;
}
/**
* {@snippet lang=c :
* int CalcAllTablesPBN(struct ddTableDealsPBN *dealsp, int mode, int trumpFilter[5], struct ddTablesRes *resp, struct allParResults *presp)
* }
*/
public static int CalcAllTablesPBN(MemorySegment dealsp, int mode, MemorySegment trumpFilter, MemorySegment resp, MemorySegment presp) {
var mh$ = CalcAllTablesPBN.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("CalcAllTablesPBN", dealsp, mode, trumpFilter, resp, presp);
}
return (int)mh$.invokeExact(dealsp, mode, trumpFilter, resp, presp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class SolveAllBoards {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SolveAllBoards"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int SolveAllBoards(struct boardsPBN *bop, struct solvedBoards *solvedp)
* }
*/
public static FunctionDescriptor SolveAllBoards$descriptor() {
return SolveAllBoards.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int SolveAllBoards(struct boardsPBN *bop, struct solvedBoards *solvedp)
* }
*/
public static MethodHandle SolveAllBoards$handle() {
return SolveAllBoards.HANDLE;
}
/**
* {@snippet lang=c :
* int SolveAllBoards(struct boardsPBN *bop, struct solvedBoards *solvedp)
* }
*/
public static int SolveAllBoards(MemorySegment bop, MemorySegment solvedp) {
var mh$ = SolveAllBoards.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SolveAllBoards", bop, solvedp);
}
return (int)mh$.invokeExact(bop, solvedp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class SolveAllBoardsBin {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SolveAllBoardsBin"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int SolveAllBoardsBin(struct boards *bop, struct solvedBoards *solvedp)
* }
*/
public static FunctionDescriptor SolveAllBoardsBin$descriptor() {
return SolveAllBoardsBin.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int SolveAllBoardsBin(struct boards *bop, struct solvedBoards *solvedp)
* }
*/
public static MethodHandle SolveAllBoardsBin$handle() {
return SolveAllBoardsBin.HANDLE;
}
/**
* {@snippet lang=c :
* int SolveAllBoardsBin(struct boards *bop, struct solvedBoards *solvedp)
* }
*/
public static int SolveAllBoardsBin(MemorySegment bop, MemorySegment solvedp) {
var mh$ = SolveAllBoardsBin.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SolveAllBoardsBin", bop, solvedp);
}
return (int)mh$.invokeExact(bop, solvedp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class SolveAllChunks {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SolveAllChunks"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int SolveAllChunks(struct boardsPBN *bop, struct solvedBoards *solvedp, int chunkSize)
* }
*/
public static FunctionDescriptor SolveAllChunks$descriptor() {
return SolveAllChunks.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int SolveAllChunks(struct boardsPBN *bop, struct solvedBoards *solvedp, int chunkSize)
* }
*/
public static MethodHandle SolveAllChunks$handle() {
return SolveAllChunks.HANDLE;
}
/**
* {@snippet lang=c :
* int SolveAllChunks(struct boardsPBN *bop, struct solvedBoards *solvedp, int chunkSize)
* }
*/
public static int SolveAllChunks(MemorySegment bop, MemorySegment solvedp, int chunkSize) {
var mh$ = SolveAllChunks.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SolveAllChunks", bop, solvedp, chunkSize);
}
return (int)mh$.invokeExact(bop, solvedp, chunkSize);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class SolveAllChunksBin {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SolveAllChunksBin"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int SolveAllChunksBin(struct boards *bop, struct solvedBoards *solvedp, int chunkSize)
* }
*/
public static FunctionDescriptor SolveAllChunksBin$descriptor() {
return SolveAllChunksBin.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int SolveAllChunksBin(struct boards *bop, struct solvedBoards *solvedp, int chunkSize)
* }
*/
public static MethodHandle SolveAllChunksBin$handle() {
return SolveAllChunksBin.HANDLE;
}
/**
* {@snippet lang=c :
* int SolveAllChunksBin(struct boards *bop, struct solvedBoards *solvedp, int chunkSize)
* }
*/
public static int SolveAllChunksBin(MemorySegment bop, MemorySegment solvedp, int chunkSize) {
var mh$ = SolveAllChunksBin.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SolveAllChunksBin", bop, solvedp, chunkSize);
}
return (int)mh$.invokeExact(bop, solvedp, chunkSize);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class SolveAllChunksPBN {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SolveAllChunksPBN"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int SolveAllChunksPBN(struct boardsPBN *bop, struct solvedBoards *solvedp, int chunkSize)
* }
*/
public static FunctionDescriptor SolveAllChunksPBN$descriptor() {
return SolveAllChunksPBN.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int SolveAllChunksPBN(struct boardsPBN *bop, struct solvedBoards *solvedp, int chunkSize)
* }
*/
public static MethodHandle SolveAllChunksPBN$handle() {
return SolveAllChunksPBN.HANDLE;
}
/**
* {@snippet lang=c :
* int SolveAllChunksPBN(struct boardsPBN *bop, struct solvedBoards *solvedp, int chunkSize)
* }
*/
public static int SolveAllChunksPBN(MemorySegment bop, MemorySegment solvedp, int chunkSize) {
var mh$ = SolveAllChunksPBN.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SolveAllChunksPBN", bop, solvedp, chunkSize);
}
return (int)mh$.invokeExact(bop, solvedp, chunkSize);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class Par {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("Par"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int Par(struct ddTableResults *tablep, struct parResults *presp, int vulnerable)
* }
*/
public static FunctionDescriptor Par$descriptor() {
return Par.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int Par(struct ddTableResults *tablep, struct parResults *presp, int vulnerable)
* }
*/
public static MethodHandle Par$handle() {
return Par.HANDLE;
}
/**
* {@snippet lang=c :
* int Par(struct ddTableResults *tablep, struct parResults *presp, int vulnerable)
* }
*/
public static int Par(MemorySegment tablep, MemorySegment presp, int vulnerable) {
var mh$ = Par.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("Par", tablep, presp, vulnerable);
}
return (int)mh$.invokeExact(tablep, presp, vulnerable);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class CalcPar {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
ddTableDeal.layout(),
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("CalcPar"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int CalcPar(struct ddTableDeal tableDeal, int vulnerable, struct ddTableResults *tablep, struct parResults *presp)
* }
*/
public static FunctionDescriptor CalcPar$descriptor() {
return CalcPar.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int CalcPar(struct ddTableDeal tableDeal, int vulnerable, struct ddTableResults *tablep, struct parResults *presp)
* }
*/
public static MethodHandle CalcPar$handle() {
return CalcPar.HANDLE;
}
/**
* {@snippet lang=c :
* int CalcPar(struct ddTableDeal tableDeal, int vulnerable, struct ddTableResults *tablep, struct parResults *presp)
* }
*/
public static int CalcPar(MemorySegment tableDeal, int vulnerable, MemorySegment tablep, MemorySegment presp) {
var mh$ = CalcPar.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("CalcPar", tableDeal, vulnerable, tablep, presp);
}
return (int)mh$.invokeExact(tableDeal, vulnerable, tablep, presp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class CalcParPBN {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
ddTableDealPBN.layout(),
Dds.C_POINTER,
Dds.C_INT,
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("CalcParPBN"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int CalcParPBN(struct ddTableDealPBN tableDealPBN, struct ddTableResults *tablep, int vulnerable, struct parResults *presp)
* }
*/
public static FunctionDescriptor CalcParPBN$descriptor() {
return CalcParPBN.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int CalcParPBN(struct ddTableDealPBN tableDealPBN, struct ddTableResults *tablep, int vulnerable, struct parResults *presp)
* }
*/
public static MethodHandle CalcParPBN$handle() {
return CalcParPBN.HANDLE;
}
/**
* {@snippet lang=c :
* int CalcParPBN(struct ddTableDealPBN tableDealPBN, struct ddTableResults *tablep, int vulnerable, struct parResults *presp)
* }
*/
public static int CalcParPBN(MemorySegment tableDealPBN, MemorySegment tablep, int vulnerable, MemorySegment presp) {
var mh$ = CalcParPBN.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("CalcParPBN", tableDealPBN, tablep, vulnerable, presp);
}
return (int)mh$.invokeExact(tableDealPBN, tablep, vulnerable, presp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class SidesPar {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SidesPar"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int SidesPar(struct ddTableResults *tablep, struct parResultsDealer sidesRes[2], int vulnerable)
* }
*/
public static FunctionDescriptor SidesPar$descriptor() {
return SidesPar.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int SidesPar(struct ddTableResults *tablep, struct parResultsDealer sidesRes[2], int vulnerable)
* }
*/
public static MethodHandle SidesPar$handle() {
return SidesPar.HANDLE;
}
/**
* {@snippet lang=c :
* int SidesPar(struct ddTableResults *tablep, struct parResultsDealer sidesRes[2], int vulnerable)
* }
*/
public static int SidesPar(MemorySegment tablep, MemorySegment sidesRes, int vulnerable) {
var mh$ = SidesPar.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SidesPar", tablep, sidesRes, vulnerable);
}
return (int)mh$.invokeExact(tablep, sidesRes, vulnerable);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class DealerPar {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_INT,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("DealerPar"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int DealerPar(struct ddTableResults *tablep, struct parResultsDealer *presp, int dealer, int vulnerable)
* }
*/
public static FunctionDescriptor DealerPar$descriptor() {
return DealerPar.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int DealerPar(struct ddTableResults *tablep, struct parResultsDealer *presp, int dealer, int vulnerable)
* }
*/
public static MethodHandle DealerPar$handle() {
return DealerPar.HANDLE;
}
/**
* {@snippet lang=c :
* int DealerPar(struct ddTableResults *tablep, struct parResultsDealer *presp, int dealer, int vulnerable)
* }
*/
public static int DealerPar(MemorySegment tablep, MemorySegment presp, int dealer, int vulnerable) {
var mh$ = DealerPar.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("DealerPar", tablep, presp, dealer, vulnerable);
}
return (int)mh$.invokeExact(tablep, presp, dealer, vulnerable);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class DealerParBin {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_INT,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("DealerParBin"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int DealerParBin(struct ddTableResults *tablep, struct parResultsMaster *presp, int dealer, int vulnerable)
* }
*/
public static FunctionDescriptor DealerParBin$descriptor() {
return DealerParBin.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int DealerParBin(struct ddTableResults *tablep, struct parResultsMaster *presp, int dealer, int vulnerable)
* }
*/
public static MethodHandle DealerParBin$handle() {
return DealerParBin.HANDLE;
}
/**
* {@snippet lang=c :
* int DealerParBin(struct ddTableResults *tablep, struct parResultsMaster *presp, int dealer, int vulnerable)
* }
*/
public static int DealerParBin(MemorySegment tablep, MemorySegment presp, int dealer, int vulnerable) {
var mh$ = DealerParBin.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("DealerParBin", tablep, presp, dealer, vulnerable);
}
return (int)mh$.invokeExact(tablep, presp, dealer, vulnerable);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class SidesParBin {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("SidesParBin"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int SidesParBin(struct ddTableResults *tablep, struct parResultsMaster sidesRes[2], int vulnerable)
* }
*/
public static FunctionDescriptor SidesParBin$descriptor() {
return SidesParBin.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int SidesParBin(struct ddTableResults *tablep, struct parResultsMaster sidesRes[2], int vulnerable)
* }
*/
public static MethodHandle SidesParBin$handle() {
return SidesParBin.HANDLE;
}
/**
* {@snippet lang=c :
* int SidesParBin(struct ddTableResults *tablep, struct parResultsMaster sidesRes[2], int vulnerable)
* }
*/
public static int SidesParBin(MemorySegment tablep, MemorySegment sidesRes, int vulnerable) {
var mh$ = SidesParBin.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("SidesParBin", tablep, sidesRes, vulnerable);
}
return (int)mh$.invokeExact(tablep, sidesRes, vulnerable);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class ConvertToDealerTextFormat {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("ConvertToDealerTextFormat"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int ConvertToDealerTextFormat(struct parResultsMaster *pres, char *resp)
* }
*/
public static FunctionDescriptor ConvertToDealerTextFormat$descriptor() {
return ConvertToDealerTextFormat.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int ConvertToDealerTextFormat(struct parResultsMaster *pres, char *resp)
* }
*/
public static MethodHandle ConvertToDealerTextFormat$handle() {
return ConvertToDealerTextFormat.HANDLE;
}
/**
* {@snippet lang=c :
* int ConvertToDealerTextFormat(struct parResultsMaster *pres, char *resp)
* }
*/
public static int ConvertToDealerTextFormat(MemorySegment pres, MemorySegment resp) {
var mh$ = ConvertToDealerTextFormat.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("ConvertToDealerTextFormat", pres, resp);
}
return (int)mh$.invokeExact(pres, resp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class ConvertToSidesTextFormat {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("ConvertToSidesTextFormat"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int ConvertToSidesTextFormat(struct parResultsMaster *pres, struct parTextResults *resp)
* }
*/
public static FunctionDescriptor ConvertToSidesTextFormat$descriptor() {
return ConvertToSidesTextFormat.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int ConvertToSidesTextFormat(struct parResultsMaster *pres, struct parTextResults *resp)
* }
*/
public static MethodHandle ConvertToSidesTextFormat$handle() {
return ConvertToSidesTextFormat.HANDLE;
}
/**
* {@snippet lang=c :
* int ConvertToSidesTextFormat(struct parResultsMaster *pres, struct parTextResults *resp)
* }
*/
public static int ConvertToSidesTextFormat(MemorySegment pres, MemorySegment resp) {
var mh$ = ConvertToSidesTextFormat.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("ConvertToSidesTextFormat", pres, resp);
}
return (int)mh$.invokeExact(pres, resp);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class AnalysePlayBin {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
deal.layout(),
playTraceBin.layout(),
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("AnalysePlayBin"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int AnalysePlayBin(struct deal dl, struct playTraceBin play, struct solvedPlay *solved, int thrId)
* }
*/
public static FunctionDescriptor AnalysePlayBin$descriptor() {
return AnalysePlayBin.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int AnalysePlayBin(struct deal dl, struct playTraceBin play, struct solvedPlay *solved, int thrId)
* }
*/
public static MethodHandle AnalysePlayBin$handle() {
return AnalysePlayBin.HANDLE;
}
/**
* {@snippet lang=c :
* int AnalysePlayBin(struct deal dl, struct playTraceBin play, struct solvedPlay *solved, int thrId)
* }
*/
public static int AnalysePlayBin(MemorySegment dl, MemorySegment play, MemorySegment solved, int thrId) {
var mh$ = AnalysePlayBin.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("AnalysePlayBin", dl, play, solved, thrId);
}
return (int)mh$.invokeExact(dl, play, solved, thrId);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class AnalysePlayPBN {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
dealPBN.layout(),
playTracePBN.layout(),
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("AnalysePlayPBN"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int AnalysePlayPBN(struct dealPBN dlPBN, struct playTracePBN playPBN, struct solvedPlay *solvedp, int thrId)
* }
*/
public static FunctionDescriptor AnalysePlayPBN$descriptor() {
return AnalysePlayPBN.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int AnalysePlayPBN(struct dealPBN dlPBN, struct playTracePBN playPBN, struct solvedPlay *solvedp, int thrId)
* }
*/
public static MethodHandle AnalysePlayPBN$handle() {
return AnalysePlayPBN.HANDLE;
}
/**
* {@snippet lang=c :
* int AnalysePlayPBN(struct dealPBN dlPBN, struct playTracePBN playPBN, struct solvedPlay *solvedp, int thrId)
* }
*/
public static int AnalysePlayPBN(MemorySegment dlPBN, MemorySegment playPBN, MemorySegment solvedp, int thrId) {
var mh$ = AnalysePlayPBN.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("AnalysePlayPBN", dlPBN, playPBN, solvedp, thrId);
}
return (int)mh$.invokeExact(dlPBN, playPBN, solvedp, thrId);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class AnalyseAllPlaysBin {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("AnalyseAllPlaysBin"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int AnalyseAllPlaysBin(struct boards *bop, struct playTracesBin *plp, struct solvedPlays *solvedp, int chunkSize)
* }
*/
public static FunctionDescriptor AnalyseAllPlaysBin$descriptor() {
return AnalyseAllPlaysBin.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int AnalyseAllPlaysBin(struct boards *bop, struct playTracesBin *plp, struct solvedPlays *solvedp, int chunkSize)
* }
*/
public static MethodHandle AnalyseAllPlaysBin$handle() {
return AnalyseAllPlaysBin.HANDLE;
}
/**
* {@snippet lang=c :
* int AnalyseAllPlaysBin(struct boards *bop, struct playTracesBin *plp, struct solvedPlays *solvedp, int chunkSize)
* }
*/
public static int AnalyseAllPlaysBin(MemorySegment bop, MemorySegment plp, MemorySegment solvedp, int chunkSize) {
var mh$ = AnalyseAllPlaysBin.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("AnalyseAllPlaysBin", bop, plp, solvedp, chunkSize);
}
return (int)mh$.invokeExact(bop, plp, solvedp, chunkSize);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class AnalyseAllPlaysPBN {
public static final FunctionDescriptor DESC = FunctionDescriptor.of(
Dds.C_INT,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_POINTER,
Dds.C_INT
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("AnalyseAllPlaysPBN"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* int AnalyseAllPlaysPBN(struct boardsPBN *bopPBN, struct playTracesPBN *plpPBN, struct solvedPlays *solvedp, int chunkSize)
* }
*/
public static FunctionDescriptor AnalyseAllPlaysPBN$descriptor() {
return AnalyseAllPlaysPBN.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* int AnalyseAllPlaysPBN(struct boardsPBN *bopPBN, struct playTracesPBN *plpPBN, struct solvedPlays *solvedp, int chunkSize)
* }
*/
public static MethodHandle AnalyseAllPlaysPBN$handle() {
return AnalyseAllPlaysPBN.HANDLE;
}
/**
* {@snippet lang=c :
* int AnalyseAllPlaysPBN(struct boardsPBN *bopPBN, struct playTracesPBN *plpPBN, struct solvedPlays *solvedp, int chunkSize)
* }
*/
public static int AnalyseAllPlaysPBN(MemorySegment bopPBN, MemorySegment plpPBN, MemorySegment solvedp, int chunkSize) {
var mh$ = AnalyseAllPlaysPBN.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("AnalyseAllPlaysPBN", bopPBN, plpPBN, solvedp, chunkSize);
}
return (int)mh$.invokeExact(bopPBN, plpPBN, solvedp, chunkSize);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class GetDDSInfo {
public static final FunctionDescriptor DESC = FunctionDescriptor.ofVoid(
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("GetDDSInfo"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* void GetDDSInfo(struct DDSInfo *info)
* }
*/
public static FunctionDescriptor GetDDSInfo$descriptor() {
return GetDDSInfo.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* void GetDDSInfo(struct DDSInfo *info)
* }
*/
public static MethodHandle GetDDSInfo$handle() {
return GetDDSInfo.HANDLE;
}
/**
* {@snippet lang=c :
* void GetDDSInfo(struct DDSInfo *info)
* }
*/
public static void GetDDSInfo(MemorySegment info) {
var mh$ = GetDDSInfo.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("GetDDSInfo", info);
}
mh$.invokeExact(info);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
private static class ErrorMessage {
public static final FunctionDescriptor DESC = FunctionDescriptor.ofVoid(
Dds.C_INT,
Dds.C_POINTER
);
public static final MethodHandle HANDLE = Linker.nativeLinker().downcallHandle(
Dds.findOrThrow("ErrorMessage"),
DESC);
}
/**
* Function descriptor for:
* {@snippet lang=c :
* void ErrorMessage(int code, char line[80])
* }
*/
public static FunctionDescriptor ErrorMessage$descriptor() {
return ErrorMessage.DESC;
}
/**
* Downcall method handle for:
* {@snippet lang=c :
* void ErrorMessage(int code, char line[80])
* }
*/
public static MethodHandle ErrorMessage$handle() {
return ErrorMessage.HANDLE;
}
/**
* {@snippet lang=c :
* void ErrorMessage(int code, char line[80])
* }
*/
public static void ErrorMessage(int code, MemorySegment line) {
var mh$ = ErrorMessage.HANDLE;
try {
if (TRACE_DOWNCALLS) {
traceDowncall("ErrorMessage", code, line);
}
mh$.invokeExact(code, line);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy