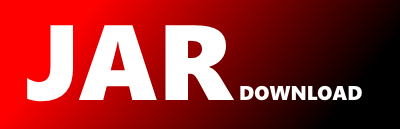
commonMain.com.strumenta.kotlinmultiplatform.Misc.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of antlr-kotlin-runtime-js Show documentation
Show all versions of antlr-kotlin-runtime-js Show documentation
Kotlin multiplatform port of ANTLR
The newest version!
/*
* Copyright (C) 2021 Strumenta
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.strumenta.kotlinmultiplatform
import kotlin.reflect.KClass
fun Array.indices(): List = this.indices.toList()
fun assert(condition: Boolean) = require(condition)
fun CharArray.convertToString(): String = this.joinToString("")
fun String.asCharArray(): CharArray = this.map { it }.toCharArray()
object Arrays {
fun equals(a: Array<*>?, b: Array<*>?): Boolean {
return (a == null && b == null) ||
((a != null && b != null) && a.contentEquals(b))
}
fun equals(a: IntArray?, b: IntArray?): Boolean {
return (a == null && b == null) ||
((a != null && b != null) && a.contentEquals(b))
}
}
expect class BitSet {
constructor()
fun set(bitIndex: Int)
fun clear(bitIndex: Int)
fun get(bitIndex: Int): Boolean
fun cardinality(): Int
fun nextSetBit(i: Int): Int
fun or(alts: BitSet)
}
//expect class ArrayList : List
expect object Collections {
fun > min(precedencePredicates: List): T
fun > max(precedencePredicates: List): T
}
object Math {
fun min(a: Int, b: Int): Int {
return kotlin.math.min(a, b)
}
fun max(a: Int, b: Int): Int {
return kotlin.math.max(a, b)
}
fun floor(d: Double): Double {
return kotlin.math.floor(d)
}
}
expect fun isCharUppercase(firstChar: Char): Boolean
expect fun isCharLowerCase(firstChar: Char): Boolean
//expect open class CopyOnWriteArrayList : MutableList {
//
//}
expect class WeakHashMap : MutableMap {
constructor()
}
expect class IdentityHashMap : MutableMap {
constructor()
}
expect class UUID {
constructor(most: Long, least: Long)
companion object {
fun fromString(encoded: String): UUID
}
}
expect fun errMessage(message: String)
fun outMessage(message: String) {
println(message)
}
expect fun Char.Companion.isSupplementaryCodePoint(codePoint: Int): Boolean
expect fun Char.Companion.toChars(codePoint: Int, resultArray: CharArray, resultIdx: Int): Int
expect fun Char.Companion.charCount(i: Int): Byte
expect fun Char.Companion.maxValue(): Char
expect fun arraycopy(src: Array, srcPos: Int, dest: Array, destPos: Int, length: Int)
expect fun arraycopy(src: IntArray, srcPos: Int, dest: IntArray, destPos: Int, length: Int)
expect class Type
expect fun Type.isInstance(any: Any?): Boolean
interface TypeDeclarator {
val classesByName: List>
}
expect fun TypeDeclarator.getType(name: String): Type
//expect fun toInt32(c: Char) : Int
expect inline fun synchronized(lock: Any, block: () -> R): R
© 2015 - 2024 Weber Informatics LLC | Privacy Policy