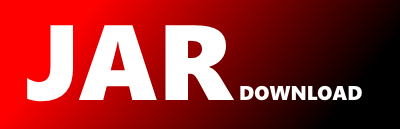
commonMain.piacenti.dslmaker.GenericParser.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dsl-maker-js Show documentation
Show all versions of dsl-maker-js Show documentation
Kotlin multiplatform library to facilitate creation of DSLs with ANTLR or a simple built in parser
package piacenti.dslmaker
import piacenti.dslmaker.abstraction.ProductionStep
import piacenti.dslmaker.errors.ParserException
import piacenti.dslmaker.interfaces.GrammarInventory
import piacenti.dslmaker.interfaces.Parser
import piacenti.dslmaker.structures.Grammar
import piacenti.dslmaker.structures.derivationgraph.DerivationGraph
import piacenti.dslmaker.structures.strategies.pretokenizestrategy.PreTokenizeExpressionMatcher
import piacenti.dslmaker.structures.strategies.pretokenizestrategy.Token
import piacenti.dslmaker.structures.strategies.pretokenizestrategy.Tokenizer
class GenericParser(grammar: Grammar):Parser {
var grammar: Grammar = grammar
set(grammar) {
field = grammar
derivationTree = DerivationGraph(field)
}
@Suppress("MemberVisibilityCanBePrivate")
var derivationTree: DerivationGraph = DerivationGraph(grammar)
private set
private var matcher: ExpressionMatcher? = null
override val highestIndexParsed: Int
get() = matcher!!.highestSuccessfulIndex
override val possibleValidTokensIfContinuingParsing: Set
get() = matcher!!.possibleValidTokensIfContinuingParsing
override val expectedTokenNotMatched: Set
get() = matcher!!.expectedTokenNotMatched
private fun getCustomEnumInstance(): List {
return grammar.container.tokens + grammar.container.productions
}
/**
* @param text
* @param matchEntireText
* @return
* @throws ParserException
*/
override fun parse(text: String, matchEntireText: Boolean , tokenizeFirst: Boolean): GenericParser {
if (tokenizeFirst) {
preTokenizeParse(derivationTree, grammar, text, matchEntireText)
} else {
matcher = ExpressionMatcher()
matcher!!.match(derivationTree, grammar, text, matchEntireText)
}
return this
}
private fun preTokenizeParse(derivationTree: DerivationGraph?, grammar: Grammar?, text: String,
matchEntireText: Boolean): GenericParser {
val tokens = getTokens(text)
val expressionMatcher = PreTokenizeExpressionMatcher()
expressionMatcher.match(derivationTree!!, grammar!!, text, tokens, matchEntireText)
return this
}
private fun getTokens(text: String): List {
val tokenDefinitions = getCustomEnumInstance()
val tokenizer = Tokenizer(grammar)
return tokenizer.tokenize(text)
}
@Suppress("MemberVisibilityCanBePrivate")
fun parseFind(text: String, regexDelimiter: String? = null): GenericParser {
matcher = ExpressionMatcher()
matcher!!.find(derivationTree, grammar, text, regexDelimiter)
return this
}
fun parseFind(text: String): GenericParser {
parseFind(text, null)
return this
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy