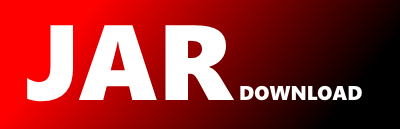
commonMain.piacenti.dslmaker.dsl.antlr.AntlrFormatterAndHighlighter.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dsl-maker-js Show documentation
Show all versions of dsl-maker-js Show documentation
Kotlin multiplatform library to facilitate creation of DSLs with ANTLR or a simple built in parser
package piacenti.dslmaker.dsl.antlr
import org.antlr.v4.kotlinruntime.CommonTokenStream
import org.antlr.v4.kotlinruntime.Token
import piacenti.dslmaker.dsl.InsertionType
interface AntlrFormatterAndHighlighter {
var exceptionOnFailure: Boolean
fun highlightAndFormat(text: String): String
fun format(text: String): String
fun highlight(text: String): String
fun highlightAndFormatComputations(text: String): AggregateComputations
fun formatComputations(text: String): AggregateComputations
fun highlightComputations(text: String): AggregateComputations
}
abstract class AntlrFormatterAndHighlighterBase : AntlrFormatterAndHighlighter {
override fun format(text: String): String {
return highlightAndFormat(text, format = true, highlight = false).result
}
override fun highlight(text: String): String {
return highlightAndFormat(text, format = false, highlight = true).result
}
override fun highlightAndFormat(text: String): String {
return highlightAndFormat(text, format = true, highlight = true).result
}
override fun highlightAndFormatComputations(text: String): AggregateComputations {
return highlightAndFormat(text, format = true, highlight = true).rewriter.allComputations
}
override fun formatComputations(text: String): AggregateComputations {
return highlightAndFormat(text, format = true, highlight = false).rewriter.allComputations
}
override fun highlightComputations(text: String): AggregateComputations {
return highlightAndFormat(text, format = false, highlight = true).rewriter.allComputations
}
protected abstract fun highlightAndFormat(text: String, format: Boolean, highlight: Boolean): AntlrFormatterAndHighlighterListener
}
interface AntlrFormatterAndHighlighterListener {
val highlight: Boolean
val format: Boolean
val tabType: String
val result: String get() = rewriter.text
val tokenStream: CommonTokenStream
val rewriter: FastTokenStreamRewriter
fun highlightToken(token: Token?, htmlClass: String, attributes: List> = emptyList()) {
if (token == null)
return
var attributeText = attributes.joinToString(separator = " ") { "${it.first}=${it.second}" }
if (attributes.isNotEmpty())
attributeText = " $attributeText"
rewriter.replace(token, "${token.text}")
}
fun highlightTokenRange(tokens: Pair, htmlClass: String, attributes: List> = emptyList()) {
val first = tokens.first
val second = tokens.second
if (first == null || second == null)
return
val attributeText = attributes.joinToString(separator = " ") { "${it.first}=${it.second}" }
rewriter.insertBefore(first, "", InsertionType.STYLE)
rewriter.insertAfter(second, "", InsertionType.STYLE)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy