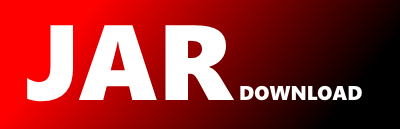
com.github.pms1.tppt.mirror.MirrorSpec Maven / Gradle / Ivy
package com.github.pms1.tppt.mirror;
import java.net.URI;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Arrays;
import java.util.Map;
import java.util.stream.Collectors;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.adapters.XmlAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
@XmlRootElement(name = "mirrorSpecification")
public class MirrorSpec {
static class PathAdapter extends XmlAdapter {
@Override
public Path unmarshal(String v) throws Exception {
return Paths.get(v);
}
@Override
public String marshal(Path v) throws Exception {
return v.toString();
}
}
@XmlElement
@XmlJavaTypeAdapter(PathAdapter.class)
public Path mirrorRepository;
@XmlElement
@XmlJavaTypeAdapter(PathAdapter.class)
public Path targetRepository;
@XmlElement(name = "sourceRepository")
public URI[] sourceRepositories;
@XmlElement(name = "iu")
public String[] ius;
@XmlElement(name = "excludeIu")
public String[] excludeIus;
@XmlElement
public AlgorithmType algorithm;
public static enum AlgorithmType {
slicer, permissiveSlicer, planner;
}
@XmlElement
public OfflineType offline;
public static enum OfflineType {
offline, online;
}
@XmlElement
public StatsType stats;
public static enum StatsType {
collect, suppress;
}
public static class Filter {
@XmlElement
Map filter;
}
static class MapAdapter extends XmlAdapter> {
public static class Entry {
public String key;
public String value;
}
private Entry from(Map.Entry e) {
Entry r = new Entry();
r.key = e.getKey();
r.value = e.getValue();
return r;
}
@Override
public MapAdapter.Entry[] marshal(Map v) throws Exception {
return v.entrySet().stream().map(this::from).toArray(s -> new MapAdapter.Entry[s]);
}
@Override
public Map unmarshal(MapAdapter.Entry[] v) throws Exception {
return Arrays.stream(v).collect(Collectors.toMap(e -> e.key, e -> e.value));
}
}
@XmlElement(name = "filter")
@XmlJavaTypeAdapter(MapAdapter.class)
public Map[] filters;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy