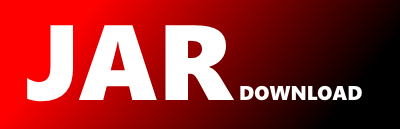
com.github.pojomvcc.RevisionObjectCacheMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pojo-mvcc Show documentation
Show all versions of pojo-mvcc Show documentation
A simple in-memory POJO Multi Version Concurrency Control (MVCC) cache.
The newest version!
package com.github.pojomvcc;
import java.util.*;
/**
* An implementation of the {@code java.util.Map} interface that retrieves all of it's
* values from a {@link RevisionObjectCache}.
*
* The returned {@code java.util.Map} is effectively a read-only {@code java.util.Map},
* that means that all methods which attempt to modify the underlying {@code java.util.Map}
* will throw a {@code java.lang.UnsupportedOperationException}.
*
* @author Aidan Morgan
*/
public class RevisionObjectCacheMap implements Map {
/**
* A {@code java.util.Map} of {@link K} to {@link V}.
*/
private Map valueMap;
/**
* Constructor.
*
* @param rootCache the {@code com.github.pojomvcc.impl.RootObjectCacheImpl} to initialise this
* {@link RevisionObjectCacheMap} from.
*/
public RevisionObjectCacheMap(RootObjectCache rootCache) {
valueMap = new HashMap(rootCache.size());
initialiseMap(rootCache);
}
/**
* Initalises the underlying {@code java.util.Map} from the provided {@link RootObjectCache}.
*
* @param rootCache
*/
private void initialiseMap(RootObjectCache rootCache) {
RevisionObjectCache cache = rootCache.checkout();
for (K key : cache.getKeys()) {
valueMap.put(key, cache.getElement(key));
}
cache.close();
}
/**
* @inheritDoc
*/
public int size() {
return valueMap.size();
}
/**
* @inheritDoc
*/
public boolean isEmpty() {
return valueMap.isEmpty();
}
/**
* @inheritDoc
*/
public boolean containsKey(Object key) {
return valueMap.containsKey(key);
}
/**
* @inheritDoc
*/
public boolean containsValue(Object value) {
return valueMap.containsValue(value);
}
/**
* @inheritDoc
*/
public V get(Object key) {
return valueMap.get(key);
}
/**
* Will throw a {@code java.lang.UnsupportedOperationException} if called.
*
* @inheritDoc
*/
public V put(K key, V value) {
throw new UnsupportedOperationException("Cannot add values to a RevisionObjectCacheMap.");
}
/**
* Will throw a {@code java.lang.UnsupportedOperationException} if called.
*
* @inheritDoc
*/
public V remove(Object key) {
throw new UnsupportedOperationException("Cannot remove values from a RevisionObjectCacheMap.");
}
/**
* Will throw a {@code java.lang.UnsupportedOperationException} if called.
*
* @inheritDoc
*/
public void putAll(Map extends K, ? extends V> m) {
throw new UnsupportedOperationException("Cannot add values to a RevisionObjectCacheMap.");
}
/**
* Will throw a {@code java.lang.UnsupportedOperationException} if called.
*
* @inheritDoc
*/
public void clear() {
throw new UnsupportedOperationException("Cannot remove values from a RevisionObjectCacheMap.");
}
/**
* @inheritDoc
*/
public Set keySet() {
return valueMap.keySet();
}
/**
* @inheritDoc
*/
public Collection values() {
return valueMap.values();
}
/**
* @inheritDoc
*/
public Set> entrySet() {
return valueMap.entrySet();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy