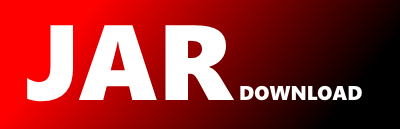
com.github.springtestdbunit.annotation.Annotations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-test-dbunit-core Show documentation
Show all versions of spring-test-dbunit-core Show documentation
Core module for the integration between the Spring testing framework and DBUnit
/*
* Copyright 2019 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.springtestdbunit.annotation;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import org.springframework.core.annotation.AnnotationUtils;
import com.github.springtestdbunit.DbUnitTestContext;
public class Annotations implements Iterable {
private final List classAnnotations;
private final List methodAnnotations;
private final List allAnnotations;
public Annotations(DbUnitTestContext context, Class extends Annotation> container, Class annotation) {
this.classAnnotations = getClassAnnotations(context.getTestClass(), container, annotation);
this.methodAnnotations = getMethodAnnotations(context.getTestMethod(), container, annotation);
List allAnnotations = new ArrayList(this.classAnnotations.size() + this.methodAnnotations.size());
allAnnotations.addAll(this.classAnnotations);
allAnnotations.addAll(this.methodAnnotations);
this.allAnnotations = Collections.unmodifiableList(allAnnotations);
}
/**
* Finds the annotations which have been declared at class level, on the class itself or any of its parents.
*
* @param element The class.
* @param container The type of container annotation to look for.
* @param annotation The type of annotation to look for.
* @return The list of annotations found on the class and its parents, can be empty but never {@code null}.
*/
private List getClassAnnotations(Class> element, Class extends Annotation> container, Class annotation) {
List annotations = new ArrayList();
addAnnotationToList(annotations, AnnotationUtils.findAnnotation(element, annotation));
addRepeatableAnnotationsToList(annotations, AnnotationUtils.findAnnotation(element, container));
return Collections.unmodifiableList(annotations);
}
/**
* Finds the annotations which have been declared at method level.
*
* @param element The method.
* @param container The type of container annotation to look for.
* @param annotation The type of annotation to look for.
* @return The list of annotations found on the method, can be empty but never {@code null}.
*/
private List getMethodAnnotations(Method element, Class extends Annotation> container, Class annotation) {
List annotations = new ArrayList();
addAnnotationToList(annotations, AnnotationUtils.findAnnotation(element, annotation));
addRepeatableAnnotationsToList(annotations, AnnotationUtils.findAnnotation(element, container));
return Collections.unmodifiableList(annotations);
}
private void addAnnotationToList(List annotations, T annotation) {
if (annotation != null) {
annotations.add(annotation);
}
}
@SuppressWarnings("unchecked")
private void addRepeatableAnnotationsToList(List annotations, Annotation container) {
if (container != null) {
T[] value = (T[]) AnnotationUtils.getValue(container);
for (T annotation : value) {
annotations.add(annotation);
}
}
}
public List getClassAnnotations() {
return this.classAnnotations;
}
public List getMethodAnnotations() {
return this.methodAnnotations;
}
public Iterator iterator() {
return this.allAnnotations.iterator();
}
public static Annotations get(DbUnitTestContext testContext,
Class extends Annotation> container, Class annotation) {
return new Annotations(testContext, container, annotation);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy