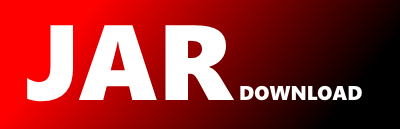
com.github.princesslana.eriscasper.action.ActionTuple Maven / Gradle / Ivy
package com.github.princesslana.eriscasper.action;
import com.github.princesslana.eriscasper.rest.Route;
import com.google.common.base.MoreObjects;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
/**
* Immutable implementation of {@link Action}.
*
* Use the static factory method to create immutable instances:
* {@code ActionTuple.of()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "Action"})
@Immutable
@CheckReturnValue
public final class ActionTuple implements Action {
private final Route route;
private final I data;
private ActionTuple(Route route, I data) {
this.route = Objects.requireNonNull(route, "route");
this.data = Objects.requireNonNull(data, "data");
}
private ActionTuple(ActionTuple original, Route route, I data) {
this.route = route;
this.data = data;
}
/**
* @return The value of the {@code route} attribute
*/
@Override
public Route getRoute() {
return route;
}
/**
* @return The value of the {@code data} attribute
*/
@Override
public I getData() {
return data;
}
/**
* Copy the current immutable object by setting a value for the {@link Action#getRoute() route} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for route
* @return A modified copy of the {@code this} object
*/
public final ActionTuple withRoute(Route value) {
if (this.route == value) return this;
Route newValue = Objects.requireNonNull(value, "route");
return new ActionTuple(this, newValue, this.data);
}
/**
* Copy the current immutable object by setting a value for the {@link Action#getData() data} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for data
* @return A modified copy of the {@code this} object
*/
public final ActionTuple withData(I value) {
if (this.data == value) return this;
I newValue = Objects.requireNonNull(value, "data");
return new ActionTuple(this, this.route, newValue);
}
/**
* This instance is equal to all instances of {@code ActionTuple} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@SuppressWarnings("unchecked")
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ActionTuple, ?>
&& equalTo((ActionTuple) another);
}
private boolean equalTo(ActionTuple another) {
return route.equals(another.route)
&& data.equals(another.data);
}
/**
* Computes a hash code from attributes: {@code route}, {@code data}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + route.hashCode();
h += (h << 5) + data.hashCode();
return h;
}
/**
* Prints the immutable value {@code Action} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Action")
.omitNullValues()
.add("route", route)
.add("data", data)
.toString();
}
/**
* Construct a new immutable {@code Action} instance.
* @param route The value for the {@code route} attribute
* @param data The value for the {@code data} attribute
* @return An immutable Action instance
*/
public static ActionTuple of(Route route, I data) {
return new ActionTuple(route, data);
}
/**
* Creates an immutable copy of a {@link Action} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param generic parameter I
* @param generic parameter O
* @param instance The instance to copy
* @return A copied immutable Action instance
*/
public static ActionTuple copyOf(Action instance) {
if (instance instanceof ActionTuple, ?>) {
return (ActionTuple) instance;
}
return ActionTuple.of(instance.getRoute(), instance.getData());
}
}