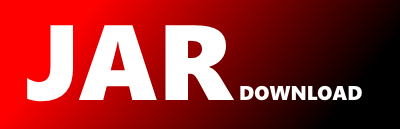
com.jarvis.cache.ICacheManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of autoload-cache-manager-api Show documentation
Show all versions of autoload-cache-manager-api Show documentation
The cache manager module of autoload-cache project
package com.jarvis.cache;
import com.jarvis.cache.exception.CacheCenterConnectionException;
import com.jarvis.cache.to.CacheKeyTO;
import com.jarvis.cache.to.CacheWrapper;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
/**
* 缓存管理
*
*
*/
public interface ICacheManager {
/**
* 当过期时间为0时,说明永不过期
*/
int NEVER_EXPIRE = 0;
/**
* 往缓存写数据
*
* @param cacheKey 缓存Key
* @param result 缓存数据
* @param method Method
* @throws CacheCenterConnectionException 缓存异常
*/
void setCache(final CacheKeyTO cacheKey, final CacheWrapper
© 2015 - 2025 Weber Informatics LLC | Privacy Policy