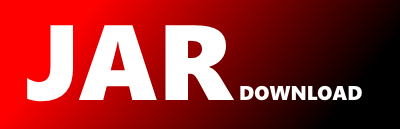
com.jarvis.cache.memcache.MemcachedCacheManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of autoload-cache-manager-memcache Show documentation
Show all versions of autoload-cache-manager-memcache Show documentation
The cache manager module of autoload-cache project
package com.jarvis.cache.memcache;
import com.jarvis.cache.ICacheManager;
import com.jarvis.cache.MSetParam;
import com.jarvis.cache.exception.CacheCenterConnectionException;
import com.jarvis.cache.to.CacheKeyTO;
import com.jarvis.cache.to.CacheWrapper;
import lombok.extern.slf4j.Slf4j;
import net.spy.memcached.MemcachedClient;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import java.util.*;
/**
* memcache缓存管理
*
*
*/
@Slf4j
public class MemcachedCacheManager implements ICacheManager {
private MemcachedClient memcachedClient;
public MemcachedCacheManager() {
}
@Override
public void setCache(final CacheKeyTO cacheKeyTO, final CacheWrapper
© 2015 - 2025 Weber Informatics LLC | Privacy Policy