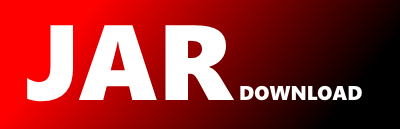
com.github.qq275860560.common.util.SignUtil Maven / Gradle / Ivy
package com.github.qq275860560.common.util;
import java.io.UnsupportedEncodingException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* @author [email protected]
*/
public class SignUtil {
private static Log log = LogFactory.getLog(SignUtil.class);
private SignUtil() {
}
public static final String DEFAULT_KEY = "key";
// 签名结果比较忽略大小写
public static boolean validate(Map map, String keyValue, String sign) {
return validate(map, DEFAULT_KEY, keyValue, sign);
}
// 签名结果比较忽略大小写
public static boolean validate(Map map, String keyName, String keyValue, String sign) {
if (StringUtils.isBlank(sign)) {
return false;
}
String resultSign = getSign(map, keyName, keyValue);
if (sign.toUpperCase().equals(resultSign.toUpperCase())) {
return true;
}
return false;
}
// 返回签名的大写字符串
public static String getSign(Map map, String keyValue) {
return getSign(map, DEFAULT_KEY, keyValue);
}
// 返回签名的大写字符串
public static String getSign(Map map, String keyName, String keyValue) {
// 过滤空值
HashMap temp = new HashMap();
for (Iterator> it = map.entrySet().iterator(); it.hasNext();) {
Entry entry = it.next();
if (StringUtils.isNotBlank(entry.getKey()) && StringUtils.isNotBlank(entry.getValue())) {
temp.put(entry.getKey(), entry.getValue());
}
}
// sort
List> paramsArray = new ArrayList>(temp.entrySet());
Collections.sort(paramsArray, new Comparator>() {
public int compare(Map.Entry o1, Map.Entry o2) {
return (o1.getKey()).toString().compareTo(o2.getKey());
}
});
// 拼接字符串
StringBuilder buff = new StringBuilder();
for (Entry entry : paramsArray) {
buff.append(entry.getKey()).append("=").append(entry.getValue()).append("&");
}
if (buff.length() > 0) {
buff.setLength(buff.length() - 1);
}
buff.append("&" + keyName + "=").append(keyValue);
String sourceStr = buff.toString();
try {
String resultSign = md5Encode(sourceStr, "UTF-8").toUpperCase();
// log.debug("sign source: [" + sourceStr + "], result sign: [" + resultSign +
// "]");
return resultSign;
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException("验证签名失败", e);
} catch (UnsupportedEncodingException e) {
throw new RuntimeException("验证签名失败", e);
}
}
private static String md5Encode(String origin, String charsetname)
throws NoSuchAlgorithmException, UnsupportedEncodingException {
MessageDigest md = MessageDigest.getInstance("MD5");
if (StringUtils.isBlank(charsetname)) {
return byteArrayToHexString(md.digest(new String(origin).getBytes()));
} else {
return byteArrayToHexString(md.digest(new String(origin).getBytes(charsetname)));
}
}
private static String byteArrayToHexString(byte b[]) {
StringBuffer sb = new StringBuffer();
for (int i = 0; i < b.length; i++) {
sb.append(byteToHexString(b[i]));
}
return sb.toString();
}
private static String byteToHexString(byte b) {
int n = b;
if (n < 0) {
n += 256;
}
int d1 = n / 16;
int d2 = n % 16;
return hexDigits[d1] + hexDigits[d2];
}
private static final String hexDigits[] = { "0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "a", "b", "c", "d",
"e", "f" };
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy