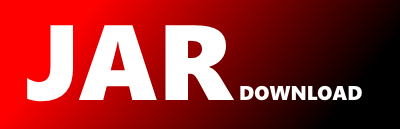
com.github.quartzwebui.utils.json.JSONWriter Maven / Gradle / Ivy
The newest version!
/*
* Copyright 1999-2017 Alibaba Group Holding Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.quartzwebui.utils.json;
import com.github.quartzwebui.utils.ExceptionUtils;
import javax.management.openmbean.CompositeData;
import javax.management.openmbean.TabularData;
import java.text.SimpleDateFormat;
import java.util.Collection;
import java.util.Date;
import java.util.Map;
public class JSONWriter {
private StringBuilder out;
public JSONWriter(){
this.out = new StringBuilder();
}
public void writeArrayStart() {
write('[');
}
public void writeComma() {
write(',');
}
public void writeArrayEnd() {
write(']');
}
public void writeNull() {
write("null");
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public void writeObject(Object o) {
if (o == null) {
writeNull();
return;
}
else if (o instanceof String) {
writeString((String) o);
return;
}
else if (o instanceof Number) {
write(o.toString());
return;
}
else if (o instanceof Boolean) {
write(o.toString());
return;
}
else if (o instanceof Date) {
writeDate((Date) o);
return;
}
else if (o instanceof Collection) {
writeArray((Collection) o);
return;
}
else if (o instanceof Throwable) {
writeError((Throwable) o);
return;
}
else if (o instanceof int[]) {
int[] array = (int[]) o;
write('[');
for (int i = 0; i < array.length; ++i) {
if (i != 0) {
write(',');
}
write(array[i]);
}
write(']');
return;
}
else if (o instanceof long[]) {
long[] array = (long[]) o;
write('[');
for (int i = 0; i < array.length; ++i) {
if (i != 0) {
write(',');
}
write(array[i]);
}
write(']');
return;
}
else if (o instanceof TabularData) {
writeTabularData((TabularData) o);
return;
}
else if (o instanceof CompositeData) {
writeCompositeData((CompositeData) o);
return;
}
else if (o instanceof Map) {
writeMap((Map) o);
return;
}
else {
Object o1 = new Object();
if (o == o1) {
write("null");
return;
}
try {
String objStr = o.getClass().getName();
writeString(objStr);
return;
} catch (Exception e) {
throw new IllegalArgumentException("not support type : " + o.getClass());
}
}
//throw new IllegalArgumentException("not support type : " + o.getClass());
}
public void writeDate(Date date) {
if (date == null) {
writeNull();
return;
}
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
writeString(dateFormat.format(date));
}
public void writeError(Throwable error) {
if (error == null) {
writeNull();
return;
}
write("{\"Class\":");
writeString(error.getClass().getName());
write(",\"Message\":");
writeString(error.getMessage());
write(",\"StackTrace\":");
writeString(ExceptionUtils.getStackTrace(error));
write('}');
}
public void writeArray(Object[] array) {
if (array == null) {
writeNull();
return;
}
write('[');
for (int i = 0; i < array.length; ++i) {
if (i != 0) {
write(',');
}
writeObject(array[i]);
}
write(']');
}
public void writeArray(Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy