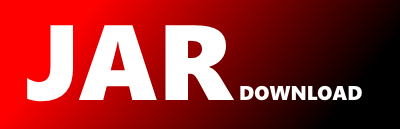
com.github.quintans.jdbc.sp.SqlParameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simplejdbc Show documentation
Show all versions of simplejdbc Show documentation
Utility class for an eazy JDBC
package com.github.quintans.jdbc.sp;
import java.sql.Types;
import com.github.quintans.jdbc.transformers.IRowTransformer;
/**
* SQL parameter used in calling a stored procedure
*
* @author paulo.quintans
*
*/
public class SqlParameter {
private boolean defined;
private SqlParameterType type;
private String name;
private Object value;
private int jdbcType;
private IRowTransformer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy