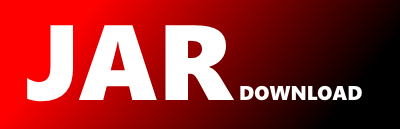
com.github.rahulsom.grooves.grails.BlockingSnapshotSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grooves-gorm Show documentation
Show all versions of grooves-gorm Show documentation
Integration with Grails' GORM API
package com.github.rahulsom.grooves.grails;
import com.github.rahulsom.grooves.api.AggregateType;
import com.github.rahulsom.grooves.api.events.BaseEvent;
import com.github.rahulsom.grooves.api.snapshots.Snapshot;
import com.github.rahulsom.grooves.queries.QuerySupport;
import com.github.rahulsom.grooves.queries.internal.BaseQuery;
import org.codehaus.groovy.runtime.DefaultGroovyMethods;
import org.grails.datastore.gorm.GormEntity;
import rx.Observable;
import java.util.Date;
import java.util.List;
import static com.github.rahulsom.grooves.grails.QueryUtil.LATEST_BY_POSITION;
import static com.github.rahulsom.grooves.grails.QueryUtil.LATEST_BY_TIMESTAMP;
import static com.github.rahulsom.grooves.grails.QueryUtil.SNAPSHOTS_BY_AGGREGATE;
import static org.codehaus.groovy.runtime.InvokerHelper.invokeStaticMethod;
import static rx.Observable.defer;
import static rx.Observable.empty;
import static rx.Observable.just;
/**
* Supplies Snapshots from a Blocking Gorm Source.
*
* @param The aggregate over which the query executes
* @param The type of the Event's id field
* @param The type of the Event
* @param The type of the Snapshot's id field
* @param The type of the Snapshot
*
* @author Rahul Somasunderam
*/
public interface BlockingSnapshotSource<
AggregateIdT,
AggregateT extends AggregateType,
EventIdT,
EventT extends BaseEvent,
SnapshotIdT,
SnapshotT extends Snapshot &
GormEntity,
QueryT extends BaseQuery
> extends QuerySupport {
SnapshotT detachSnapshot(SnapshotT snapshot);
@Override
default Observable getSnapshot(long maxPosition, AggregateT aggregate) {
return defer(() -> {
//noinspection unchecked
List snapshots = (List) (maxPosition == Long.MAX_VALUE ?
invokeStaticMethod(
getSnapshotClass(),
SNAPSHOTS_BY_AGGREGATE,
new Object[]{aggregate.getId(), LATEST_BY_POSITION}) :
invokeStaticMethod(
getSnapshotClass(),
QueryUtil.SNAPSHOTS_BY_POSITION,
new Object[]{aggregate.getId(), maxPosition, LATEST_BY_POSITION}));
return DefaultGroovyMethods.asBoolean(snapshots) ?
just(detachSnapshot(snapshots.get(0))) :
empty();
});
}
@Override
default Observable getSnapshot(Date maxTimestamp, AggregateT aggregate) {
return defer(() -> {
//noinspection unchecked
List snapshots = (List) (maxTimestamp == null ?
invokeStaticMethod(
getSnapshotClass(),
SNAPSHOTS_BY_AGGREGATE,
new Object[]{aggregate.getId(), LATEST_BY_TIMESTAMP}) :
invokeStaticMethod(
getSnapshotClass(),
QueryUtil.SNAPSHOTS_BY_TIMETTAMP,
new Object[]{aggregate.getId(), maxTimestamp, LATEST_BY_TIMESTAMP}));
return DefaultGroovyMethods.asBoolean(snapshots) ?
just(detachSnapshot(snapshots.get(0))) :
empty();
});
}
Class getSnapshotClass();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy