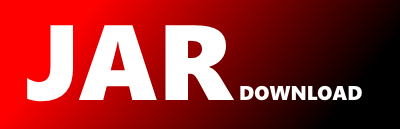
com.github.rahulsom.grooves.grails.GormQuerySupport Maven / Gradle / Ivy
Show all versions of grooves-gorm Show documentation
package com.github.rahulsom.grooves.grails;
import com.github.rahulsom.grooves.api.AggregateType;
import com.github.rahulsom.grooves.api.events.BaseEvent;
import com.github.rahulsom.grooves.api.snapshots.Snapshot;
import com.github.rahulsom.grooves.queries.QuerySupport;
import com.github.rahulsom.grooves.queries.internal.BaseQuery;
import org.grails.datastore.gorm.GormEntity;
import rx.Observable;
import java.util.Date;
import java.util.List;
/**
* Gorm Support for Query Util.
*
* This is the preferred way of writing Grooves applications with Grails.
*
* @param The aggregate over which the query executes
* @param The type of the Event's id field
* @param The type of the Event
* @param The type of the Snapshot's id field
* @param The type of the Snapshot
*
* @author Rahul Somasunderam
* @deprecated Use {@link BlockingEventSource} and {@link BlockingSnapshotSource} instead
*/
@Deprecated
// tag::documented[]
public interface GormQuerySupport<
AggregateIdT,
AggregateT extends AggregateType & GormEntity,
EventIdT,
EventT extends BaseEvent &
GormEntity,
SnapshotIdT,
SnapshotT extends Snapshot &
GormEntity,
QueryT extends BaseQuery
> extends QuerySupport, //<1>
BlockingEventSource,
BlockingSnapshotSource {
Class getEventClass(); // <2>
Class getSnapshotClass(); // <3>
@Override
default Observable getSnapshot(long maxPosition, AggregateT aggregate) {
return BlockingSnapshotSource.super
.getSnapshot(maxPosition, aggregate); // <4>
}
@Override
default Observable getSnapshot(Date maxTimestamp, AggregateT aggregate) {
return BlockingSnapshotSource.super
.getSnapshot(maxTimestamp, aggregate);
}
@Override
default Observable getUncomputedEvents(
AggregateT aggregate, SnapshotT lastSnapshot, long version) {
return BlockingEventSource.super
.getUncomputedEvents(aggregate, lastSnapshot, version);
}
@Override
default Observable getUncomputedEvents(
AggregateT aggregate, SnapshotT lastSnapshot, Date snapshotTime) {
return BlockingEventSource.super
.getUncomputedEvents(aggregate, lastSnapshot, snapshotTime);
}
}
// end::documented[]