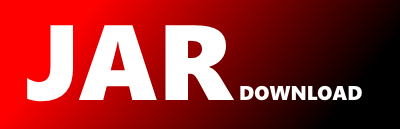
com.github.rahulsom.grooves.grails.RxEventSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grooves-gorm Show documentation
Show all versions of grooves-gorm Show documentation
Integration with Grails' GORM API
package com.github.rahulsom.grooves.grails;
import com.github.rahulsom.grooves.api.AggregateType;
import com.github.rahulsom.grooves.api.events.BaseEvent;
import com.github.rahulsom.grooves.api.snapshots.Snapshot;
import com.github.rahulsom.grooves.queries.QuerySupport;
import com.github.rahulsom.grooves.queries.internal.BaseQuery;
import grails.gorm.rx.RxEntity;
import rx.Observable;
import java.util.Date;
import java.util.List;
import static com.github.rahulsom.grooves.grails.QueryUtil.*;
import static org.codehaus.groovy.runtime.InvokerHelper.invokeStaticMethod;
import static rx.Observable.from;
/**
* Supplies Events from an Rx Gorm Source.
*
* @param The aggregate over which the query executes
* @param The type of the Event's id field
* @param The type of the Event
* @param The type of the Snapshot's id field
* @param The type of the Snapshot
*
* @author Rahul Somasunderam
*/
public interface RxEventSource<
AggregateIdT,
AggregateT extends AggregateType,
EventIdT,
EventT extends BaseEvent &
RxEntity,
SnapshotIdT,
SnapshotT extends Snapshot,
QueryT extends BaseQuery
> extends QuerySupport {
Class getEventClass();
@Override
default Observable getUncomputedEvents(
AggregateT aggregate, SnapshotT lastSnapshot, long version) {
final long position = lastSnapshot == null || lastSnapshot.getLastEventPosition() == null ?
0 : lastSnapshot.getLastEventPosition();
//noinspection unchecked
return (Observable) invokeStaticMethod(
getEventClass(),
UNCOMPUTED_EVENTS_BY_VERSION,
new Object[]{aggregate, position, version, INCREMENTAL_BY_POSITION});
}
@Override
default Observable getUncomputedEvents(
AggregateT aggregate, SnapshotT lastSnapshot, Date snapshotTime) {
final Date lastEventTimestamp = lastSnapshot.getLastEventTimestamp();
final String method = lastEventTimestamp == null ?
UNCOMPUTED_EVENTS_BEFORE_DATE :
UNCOMPUTED_EVENTS_BY_DATE_RANGE;
final Object[] params = lastEventTimestamp == null ?
new Object[]{aggregate, snapshotTime, INCREMENTAL_BY_TIMESTAMP} :
new Object[]{aggregate, lastEventTimestamp, snapshotTime, INCREMENTAL_BY_TIMESTAMP};
//noinspection unchecked
return (Observable) invokeStaticMethod(getEventClass(), method, params);
}
/**
* Hook to turn a proxy for an aggregate into an aggregate.
*
* @param aggregate The aggregate that may or may not be a proxy
*
* @return An observable of the aggregate
*/
Observable reattachAggregate(AggregateT aggregate);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy