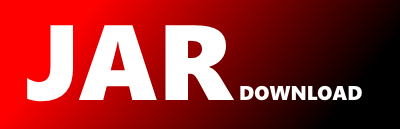
org.hl7.v3.COCTMT530000UVControlActEvent Maven / Gradle / Ivy
Show all versions of ihe-iti Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2013.05.03 at 09:18:53 PM PDT
//
package org.hl7.v3;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.apache.commons.lang.builder.ToStringStyle;
/**
* Java class for COCT_MT530000UV.ControlActEvent complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="COCT_MT530000UV.ControlActEvent">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <group ref="{urn:hl7-org:v3}InfrastructureRootElements"/>
* <element name="id" type="{urn:hl7-org:v3}II" minOccurs="0"/>
* <element name="code" type="{urn:hl7-org:v3}CD"/>
* <element name="statusCode" type="{urn:hl7-org:v3}CS"/>
* <element name="effectiveTime" type="{urn:hl7-org:v3}TS"/>
* <element name="reasonCode" type="{urn:hl7-org:v3}CV" minOccurs="0"/>
* <element name="responsibleParty" type="{urn:hl7-org:v3}COCT_MT530000UV.ResponsibleParty1" minOccurs="0"/>
* <element name="author" type="{urn:hl7-org:v3}COCT_MT530000UV.Author" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attGroup ref="{urn:hl7-org:v3}InfrastructureRootAttributes"/>
* <attribute name="nullFlavor" type="{urn:hl7-org:v3}NullFlavor" />
* <attribute name="classCode" type="{urn:hl7-org:v3}ActClassControlAct" fixed="CACT" />
* <attribute name="moodCode" type="{urn:hl7-org:v3}ActMood" fixed="EVN" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "COCT_MT530000UV.ControlActEvent", propOrder = {
"realmCode",
"typeId",
"templateId",
"id",
"code",
"statusCode",
"effectiveTime",
"reasonCode",
"responsibleParty",
"author"
})
public class COCTMT530000UVControlActEvent {
protected List realmCode;
protected II typeId;
protected List templateId;
protected II id;
@XmlElement(required = true)
protected CD code;
@XmlElement(required = true)
protected CS statusCode;
@XmlElement(required = true)
protected TS effectiveTime;
protected CV reasonCode;
@XmlElementRef(name = "responsibleParty", namespace = "urn:hl7-org:v3", type = JAXBElement.class, required = false)
protected JAXBElement responsibleParty;
@XmlElement(nillable = true)
protected List author;
@XmlAttribute(name = "nullFlavor")
protected List nullFlavor;
@XmlAttribute(name = "classCode")
protected ActClassControlAct classCode;
@XmlAttribute(name = "moodCode")
protected List moodCode;
/**
* Gets the value of the realmCode property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the realmCode property.
*
*
* For example, to add a new item, do as follows:
*
* getRealmCode().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CS }
*
*
*/
public List getRealmCode() {
if (realmCode == null) {
realmCode = new ArrayList();
}
return this.realmCode;
}
/**
* Gets the value of the typeId property.
*
* @return
* possible object is
* {@link II }
*
*/
public II getTypeId() {
return typeId;
}
/**
* Sets the value of the typeId property.
*
* @param value
* allowed object is
* {@link II }
*
*/
public void setTypeId(II value) {
this.typeId = value;
}
/**
* Gets the value of the templateId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the templateId property.
*
*
* For example, to add a new item, do as follows:
*
* getTemplateId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link II }
*
*
*/
public List getTemplateId() {
if (templateId == null) {
templateId = new ArrayList();
}
return this.templateId;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link II }
*
*/
public II getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link II }
*
*/
public void setId(II value) {
this.id = value;
}
/**
* Gets the value of the code property.
*
* @return
* possible object is
* {@link CD }
*
*/
public CD getCode() {
return code;
}
/**
* Sets the value of the code property.
*
* @param value
* allowed object is
* {@link CD }
*
*/
public void setCode(CD value) {
this.code = value;
}
/**
* Gets the value of the statusCode property.
*
* @return
* possible object is
* {@link CS }
*
*/
public CS getStatusCode() {
return statusCode;
}
/**
* Sets the value of the statusCode property.
*
* @param value
* allowed object is
* {@link CS }
*
*/
public void setStatusCode(CS value) {
this.statusCode = value;
}
/**
* Gets the value of the effectiveTime property.
*
* @return
* possible object is
* {@link TS }
*
*/
public TS getEffectiveTime() {
return effectiveTime;
}
/**
* Sets the value of the effectiveTime property.
*
* @param value
* allowed object is
* {@link TS }
*
*/
public void setEffectiveTime(TS value) {
this.effectiveTime = value;
}
/**
* Gets the value of the reasonCode property.
*
* @return
* possible object is
* {@link CV }
*
*/
public CV getReasonCode() {
return reasonCode;
}
/**
* Sets the value of the reasonCode property.
*
* @param value
* allowed object is
* {@link CV }
*
*/
public void setReasonCode(CV value) {
this.reasonCode = value;
}
/**
* Gets the value of the responsibleParty property.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link COCTMT530000UVResponsibleParty1 }{@code >}
*
*/
public JAXBElement getResponsibleParty() {
return responsibleParty;
}
/**
* Sets the value of the responsibleParty property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link COCTMT530000UVResponsibleParty1 }{@code >}
*
*/
public void setResponsibleParty(JAXBElement value) {
this.responsibleParty = value;
}
/**
* Gets the value of the author property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the author property.
*
*
* For example, to add a new item, do as follows:
*
* getAuthor().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link COCTMT530000UVAuthor }
*
*
*/
public List getAuthor() {
if (author == null) {
author = new ArrayList();
}
return this.author;
}
/**
* Gets the value of the nullFlavor property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the nullFlavor property.
*
*
* For example, to add a new item, do as follows:
*
* getNullFlavor().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getNullFlavor() {
if (nullFlavor == null) {
nullFlavor = new ArrayList();
}
return this.nullFlavor;
}
/**
* Gets the value of the classCode property.
*
* @return
* possible object is
* {@link ActClassControlAct }
*
*/
public ActClassControlAct getClassCode() {
if (classCode == null) {
return ActClassControlAct.CACT;
} else {
return classCode;
}
}
/**
* Sets the value of the classCode property.
*
* @param value
* allowed object is
* {@link ActClassControlAct }
*
*/
public void setClassCode(ActClassControlAct value) {
this.classCode = value;
}
/**
* Gets the value of the moodCode property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the moodCode property.
*
*
* For example, to add a new item, do as follows:
*
* getMoodCode().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getMoodCode() {
if (moodCode == null) {
moodCode = new ArrayList();
}
return this.moodCode;
}
public COCTMT530000UVControlActEvent withRealmCode(CS... values) {
if (values!= null) {
for (CS value: values) {
getRealmCode().add(value);
}
}
return this;
}
public COCTMT530000UVControlActEvent withRealmCode(Collection values) {
if (values!= null) {
getRealmCode().addAll(values);
}
return this;
}
public COCTMT530000UVControlActEvent withTypeId(II value) {
setTypeId(value);
return this;
}
public COCTMT530000UVControlActEvent withTemplateId(II... values) {
if (values!= null) {
for (II value: values) {
getTemplateId().add(value);
}
}
return this;
}
public COCTMT530000UVControlActEvent withTemplateId(Collection values) {
if (values!= null) {
getTemplateId().addAll(values);
}
return this;
}
public COCTMT530000UVControlActEvent withId(II value) {
setId(value);
return this;
}
public COCTMT530000UVControlActEvent withCode(CD value) {
setCode(value);
return this;
}
public COCTMT530000UVControlActEvent withStatusCode(CS value) {
setStatusCode(value);
return this;
}
public COCTMT530000UVControlActEvent withEffectiveTime(TS value) {
setEffectiveTime(value);
return this;
}
public COCTMT530000UVControlActEvent withReasonCode(CV value) {
setReasonCode(value);
return this;
}
public COCTMT530000UVControlActEvent withResponsibleParty(JAXBElement value) {
setResponsibleParty(value);
return this;
}
public COCTMT530000UVControlActEvent withAuthor(COCTMT530000UVAuthor... values) {
if (values!= null) {
for (COCTMT530000UVAuthor value: values) {
getAuthor().add(value);
}
}
return this;
}
public COCTMT530000UVControlActEvent withAuthor(Collection values) {
if (values!= null) {
getAuthor().addAll(values);
}
return this;
}
public COCTMT530000UVControlActEvent withNullFlavor(String... values) {
if (values!= null) {
for (String value: values) {
getNullFlavor().add(value);
}
}
return this;
}
public COCTMT530000UVControlActEvent withNullFlavor(Collection values) {
if (values!= null) {
getNullFlavor().addAll(values);
}
return this;
}
public COCTMT530000UVControlActEvent withClassCode(ActClassControlAct value) {
setClassCode(value);
return this;
}
public COCTMT530000UVControlActEvent withMoodCode(String... values) {
if (values!= null) {
for (String value: values) {
getMoodCode().add(value);
}
}
return this;
}
public COCTMT530000UVControlActEvent withMoodCode(Collection values) {
if (values!= null) {
getMoodCode().addAll(values);
}
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}