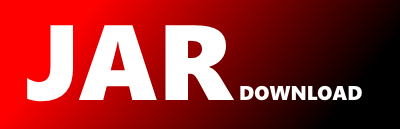
ihe.iti.svs._2008.ED Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ihe-iti Show documentation
Show all versions of ihe-iti Show documentation
Codegen for IHE ITI Profiles.
package ihe.iti.svs._2008;
import java.util.Collection;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.apache.commons.lang.builder.ToStringStyle;
/**
*
* Data that is primarily intended for human interpretation
* or for further machine processing is outside the scope of
* HL7. This includes unformatted or formatted written language,
* multimedia data, or structured information as defined by a
* different standard (e.g., XML-signatures.) Instead of the
* data itself, an ED may contain
* only a reference (see TEL.) Note
* that the ST data type is a
* specialization of
* when the is text/plain.
*
*
* Java class for ED complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ED">
* <complexContent>
* <extension base="{urn:ihe:iti:svs:2008}BIN">
* <sequence>
* <element name="reference" type="{urn:ihe:iti:svs:2008}TEL" minOccurs="0"/>
* <element name="thumbnail" type="{urn:ihe:iti:svs:2008}thumbnail" minOccurs="0"/>
* </sequence>
* <attribute name="mediaType" type="{urn:ihe:iti:svs:2008}cs" default="text/plain" />
* <attribute name="language" type="{urn:ihe:iti:svs:2008}cs" />
* <attribute name="compression" type="{urn:ihe:iti:svs:2008}CompressionAlgorithm" />
* <attribute name="integrityCheck" type="{urn:ihe:iti:svs:2008}bin" />
* <attribute name="integrityCheckAlgorithm" type="{urn:ihe:iti:svs:2008}IntegrityCheckAlgorithm" default="SHA-1" />
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ED", propOrder = {
"reference",
"thumbnail"
})
@XmlSeeAlso({
Thumbnail.class,
ST.class
})
public class ED
extends BIN
{
protected TEL reference;
protected Thumbnail thumbnail;
@XmlAttribute(name = "mediaType")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String mediaType;
@XmlAttribute(name = "language")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String language;
@XmlAttribute(name = "compression")
protected CompressionAlgorithm compression;
@XmlAttribute(name = "integrityCheck")
protected byte[] integrityCheck;
@XmlAttribute(name = "integrityCheckAlgorithm")
protected IntegrityCheckAlgorithm integrityCheckAlgorithm;
/**
* Gets the value of the reference property.
*
* @return
* possible object is
* {@link TEL }
*
*/
public TEL getReference() {
return reference;
}
/**
* Sets the value of the reference property.
*
* @param value
* allowed object is
* {@link TEL }
*
*/
public void setReference(TEL value) {
this.reference = value;
}
/**
* Gets the value of the thumbnail property.
*
* @return
* possible object is
* {@link Thumbnail }
*
*/
public Thumbnail getThumbnail() {
return thumbnail;
}
/**
* Sets the value of the thumbnail property.
*
* @param value
* allowed object is
* {@link Thumbnail }
*
*/
public void setThumbnail(Thumbnail value) {
this.thumbnail = value;
}
/**
* Gets the value of the mediaType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMediaType() {
if (mediaType == null) {
return "text/plain";
} else {
return mediaType;
}
}
/**
* Sets the value of the mediaType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMediaType(String value) {
this.mediaType = value;
}
/**
* Gets the value of the language property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLanguage() {
return language;
}
/**
* Sets the value of the language property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLanguage(String value) {
this.language = value;
}
/**
* Gets the value of the compression property.
*
* @return
* possible object is
* {@link CompressionAlgorithm }
*
*/
public CompressionAlgorithm getCompression() {
return compression;
}
/**
* Sets the value of the compression property.
*
* @param value
* allowed object is
* {@link CompressionAlgorithm }
*
*/
public void setCompression(CompressionAlgorithm value) {
this.compression = value;
}
/**
* Gets the value of the integrityCheck property.
*
* @return
* possible object is
* byte[]
*/
public byte[] getIntegrityCheck() {
return integrityCheck;
}
/**
* Sets the value of the integrityCheck property.
*
* @param value
* allowed object is
* byte[]
*/
public void setIntegrityCheck(byte[] value) {
this.integrityCheck = value;
}
/**
* Gets the value of the integrityCheckAlgorithm property.
*
* @return
* possible object is
* {@link IntegrityCheckAlgorithm }
*
*/
public IntegrityCheckAlgorithm getIntegrityCheckAlgorithm() {
if (integrityCheckAlgorithm == null) {
return IntegrityCheckAlgorithm.SHA_1;
} else {
return integrityCheckAlgorithm;
}
}
/**
* Sets the value of the integrityCheckAlgorithm property.
*
* @param value
* allowed object is
* {@link IntegrityCheckAlgorithm }
*
*/
public void setIntegrityCheckAlgorithm(IntegrityCheckAlgorithm value) {
this.integrityCheckAlgorithm = value;
}
public ED withReference(TEL value) {
setReference(value);
return this;
}
public ED withThumbnail(Thumbnail value) {
setThumbnail(value);
return this;
}
public ED withMediaType(String value) {
setMediaType(value);
return this;
}
public ED withLanguage(String value) {
setLanguage(value);
return this;
}
public ED withCompression(CompressionAlgorithm value) {
setCompression(value);
return this;
}
public ED withIntegrityCheck(byte[] value) {
setIntegrityCheck(value);
return this;
}
public ED withIntegrityCheckAlgorithm(IntegrityCheckAlgorithm value) {
setIntegrityCheckAlgorithm(value);
return this;
}
@Override
public ED withRepresentation(BinaryDataEncoding value) {
setRepresentation(value);
return this;
}
@Override
public ED withNullFlavor(String... values) {
if (values!= null) {
for (String value: values) {
getNullFlavor().add(value);
}
}
return this;
}
@Override
public ED withNullFlavor(Collection values) {
if (values!= null) {
getNullFlavor().addAll(values);
}
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy