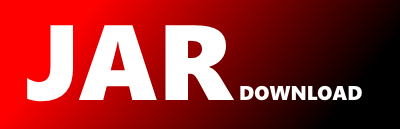
com.github.rahulsom.cda.ADXP Maven / Gradle / Ivy
Show all versions of ihe-iti Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2014.07.06 at 04:34:47 PM PDT
//
package com.github.rahulsom.cda;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.apache.commons.lang.builder.ToStringStyle;
/**
* A character string that may have a type-tag signifying its role in the address. Typical parts that exist in about every address are street, house number, or post box, postal code, city, country but other roles may be defined regionally, nationally, or on an enterprise level (e.g. in military addresses). Addresses are usually broken up into lines, which are indicated by special line-breaking delimiter elements (e.g., DEL).
*
* Java class for ADXP complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ADXP">
* <complexContent>
* <extension base="{urn:hl7-org:v3}ST">
* <attribute name="partType" type="{urn:hl7-org:v3}AddressPartType" />
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ADXP", namespace = "urn:hl7-org:v3")
@XmlSeeAlso({
AdxpDeliveryMode.class,
AdxpDeliveryInstallationType.class,
AdxpDelimiter.class,
AdxpPrecinct.class,
AdxpStreetAddressLine.class,
AdxpUnitType.class,
AdxpCountry.class,
AdxpHouseNumberNumeric.class,
AdxpUnitID.class,
AdxpCareOf.class,
AdxpDeliveryInstallationQualifier.class,
AdxpCounty.class,
AdxpHouseNumber.class,
AdxpBuildingNumberSuffix.class,
AdxpCensusTract.class,
AdxpDeliveryAddressLine.class,
AdxpDeliveryModeIdentifier.class,
AdxpStreetName.class,
AdxpStreetNameType.class,
AdxpDirection.class,
AdxpState.class,
AdxpCity.class,
AdxpPostalCode.class,
AdxpStreetNameBase.class,
AdxpAdditionalLocator.class,
AdxpPostBox.class,
AdxpDeliveryInstallationArea.class
})
public class ADXP
extends ST
{
@XmlAttribute(name = "partType")
protected List partType;
/**
* Gets the value of the partType property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the partType property.
*
*
* For example, to add a new item, do as follows:
*
* getPartType().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getPartType() {
if (partType == null) {
partType = new ArrayList();
}
return this.partType;
}
public ADXP withPartType(String... values) {
if (values!= null) {
for (String value: values) {
getPartType().add(value);
}
}
return this;
}
public ADXP withPartType(Collection values) {
if (values!= null) {
getPartType().addAll(values);
}
return this;
}
@Override
public ADXP withMediaType(String value) {
setMediaType(value);
return this;
}
@Override
public ADXP withLanguage(String value) {
setLanguage(value);
return this;
}
@Override
public ADXP withCompression(CompressionAlgorithm value) {
setCompression(value);
return this;
}
@Override
public ADXP withIntegrityCheck(byte[] value) {
setIntegrityCheck(value);
return this;
}
@Override
public ADXP withIntegrityCheckAlgorithm(IntegrityCheckAlgorithm value) {
setIntegrityCheckAlgorithm(value);
return this;
}
@Override
public ADXP withContent(Serializable... values) {
if (values!= null) {
for (Serializable value: values) {
getContent().add(value);
}
}
return this;
}
@Override
public ADXP withContent(Collection values) {
if (values!= null) {
getContent().addAll(values);
}
return this;
}
@Override
public ADXP withRepresentation(BinaryDataEncoding value) {
setRepresentation(value);
return this;
}
@Override
public ADXP withNullFlavor(String... values) {
if (values!= null) {
for (String value: values) {
getNullFlavor().add(value);
}
}
return this;
}
@Override
public ADXP withNullFlavor(Collection values) {
if (values!= null) {
getNullFlavor().addAll(values);
}
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}