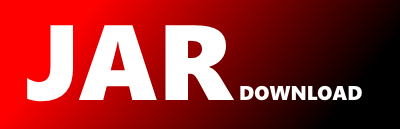
com.github.rahulsom.cda.ED Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ihe-iti Show documentation
Show all versions of ihe-iti Show documentation
Codegen for IHE ITI Profiles.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2014.07.06 at 04:34:47 PM PDT
//
package com.github.rahulsom.cda;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlElementRefs;
import javax.xml.bind.annotation.XmlMixed;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import com.sun.xml.bind.annotation.OverrideAnnotationOf;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.apache.commons.lang.builder.ToStringStyle;
/**
* Data that is primarily intended for human interpretation or for further machine processing is outside the scope of HL7. This includes unformatted or formatted written language, multimedia data, or structured information as defined by a different standard (e.g., XML-signatures.) Instead of the data itself, an ED may contain only a reference (see TEL.) Note that the ST data type is a specialization of the ED data type when the ED media type is text/plain.
*
* Java class for ED complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ED">
* <complexContent>
* <extension base="{urn:hl7-org:v3}BIN">
* <sequence>
* <element name="reference" type="{urn:hl7-org:v3}TEL" minOccurs="0"/>
* <element name="thumbnail" type="{urn:hl7-org:v3}thumbnail" minOccurs="0"/>
* </sequence>
* <attribute name="mediaType" type="{urn:hl7-org:v3}cs" default="text/plain" />
* <attribute name="language" type="{urn:hl7-org:v3}cs" />
* <attribute name="compression" type="{urn:hl7-org:v3}CompressionAlgorithm" />
* <attribute name="integrityCheck" type="{urn:hl7-org:v3}bin" />
* <attribute name="integrityCheckAlgorithm" type="{urn:hl7-org:v3}IntegrityCheckAlgorithm" default="SHA-1" />
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ED", namespace = "urn:hl7-org:v3")
@XmlSeeAlso({
Thumbnail.class,
ST.class
})
public class ED
extends BIN
{
@XmlElementRefs({
@XmlElementRef(name = "thumbnail", namespace = "urn:hl7-org:v3", type = JAXBElement.class, required = false),
@XmlElementRef(name = "reference", namespace = "urn:hl7-org:v3", type = JAXBElement.class, required = false)
})
@XmlMixed
@OverrideAnnotationOf
protected List contentOverrideForED;
@XmlAttribute(name = "mediaType")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String mediaType;
@XmlAttribute(name = "language")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String language;
@XmlAttribute(name = "compression")
protected CompressionAlgorithm compression;
@XmlAttribute(name = "integrityCheck")
protected byte[] integrityCheck;
@XmlAttribute(name = "integrityCheckAlgorithm")
protected IntegrityCheckAlgorithm integrityCheckAlgorithm;
/**
* Gets the value of the mediaType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMediaType() {
if (mediaType == null) {
return "text/plain";
} else {
return mediaType;
}
}
/**
* Sets the value of the mediaType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMediaType(String value) {
this.mediaType = value;
}
/**
* Gets the value of the language property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLanguage() {
return language;
}
/**
* Sets the value of the language property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLanguage(String value) {
this.language = value;
}
/**
* Gets the value of the compression property.
*
* @return
* possible object is
* {@link CompressionAlgorithm }
*
*/
public CompressionAlgorithm getCompression() {
return compression;
}
/**
* Sets the value of the compression property.
*
* @param value
* allowed object is
* {@link CompressionAlgorithm }
*
*/
public void setCompression(CompressionAlgorithm value) {
this.compression = value;
}
/**
* Gets the value of the integrityCheck property.
*
* @return
* possible object is
* byte[]
*/
public byte[] getIntegrityCheck() {
return integrityCheck;
}
/**
* Sets the value of the integrityCheck property.
*
* @param value
* allowed object is
* byte[]
*/
public void setIntegrityCheck(byte[] value) {
this.integrityCheck = value;
}
/**
* Gets the value of the integrityCheckAlgorithm property.
*
* @return
* possible object is
* {@link IntegrityCheckAlgorithm }
*
*/
public IntegrityCheckAlgorithm getIntegrityCheckAlgorithm() {
if (integrityCheckAlgorithm == null) {
return IntegrityCheckAlgorithm.SHA_1;
} else {
return integrityCheckAlgorithm;
}
}
/**
* Sets the value of the integrityCheckAlgorithm property.
*
* @param value
* allowed object is
* {@link IntegrityCheckAlgorithm }
*
*/
public void setIntegrityCheckAlgorithm(IntegrityCheckAlgorithm value) {
this.integrityCheckAlgorithm = value;
}
public ED withMediaType(String value) {
setMediaType(value);
return this;
}
public ED withLanguage(String value) {
setLanguage(value);
return this;
}
public ED withCompression(CompressionAlgorithm value) {
setCompression(value);
return this;
}
public ED withIntegrityCheck(byte[] value) {
setIntegrityCheck(value);
return this;
}
public ED withIntegrityCheckAlgorithm(IntegrityCheckAlgorithm value) {
setIntegrityCheckAlgorithm(value);
return this;
}
@Override
public ED withContent(Serializable... values) {
if (values!= null) {
for (Serializable value: values) {
getContent().add(value);
}
}
return this;
}
@Override
public ED withContent(Collection values) {
if (values!= null) {
getContent().addAll(values);
}
return this;
}
@Override
public ED withRepresentation(BinaryDataEncoding value) {
setRepresentation(value);
return this;
}
@Override
public ED withNullFlavor(String... values) {
if (values!= null) {
for (String value: values) {
getNullFlavor().add(value);
}
}
return this;
}
@Override
public ED withNullFlavor(Collection values) {
if (values!= null) {
getNullFlavor().addAll(values);
}
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy