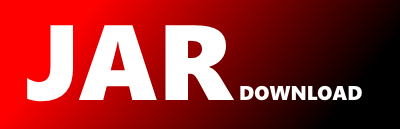
ihe.iti.svs._2008.CD Maven / Gradle / Ivy
Show all versions of ihe-iti Show documentation
package ihe.iti.svs._2008;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.apache.commons.lang.builder.ToStringStyle;
/**
*
* A concept descriptor represents any kind of concept usually
* by giving a code defined in a code system. A concept
* descriptor can contain the original text or phrase that
* served as the basis of the coding and one or more
* translations into different coding systems. A concept
* descriptor can also contain qualifiers to describe, e.g.,
* the concept of a "left foot" as a postcoordinated term built
* from the primary code "FOOT" and the qualifier "LEFT".
* In exceptional cases, the concept descriptor need not
* contain a code but only the original text describing
* that concept.
*
*
* Java class for CD complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="CD">
* <complexContent>
* <extension base="{urn:ihe:iti:svs:2008}ANY">
* <sequence>
* <element name="originalText" type="{urn:ihe:iti:svs:2008}ED" minOccurs="0"/>
* <element name="qualifier" type="{urn:ihe:iti:svs:2008}CR" maxOccurs="unbounded" minOccurs="0"/>
* <element name="translation" type="{urn:ihe:iti:svs:2008}CD" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="code" type="{urn:ihe:iti:svs:2008}cs" />
* <attribute name="codeSystem" type="{urn:ihe:iti:svs:2008}uid" />
* <attribute name="codeSystemName" type="{urn:ihe:iti:svs:2008}st" />
* <attribute name="codeSystemVersion" type="{urn:ihe:iti:svs:2008}st" />
* <attribute name="displayName" type="{urn:ihe:iti:svs:2008}st" />
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CD", propOrder = {
"originalText",
"qualifier",
"translation"
})
@XmlSeeAlso({
BXITCD.class,
SXCMCD.class,
CE.class
})
public class CD
extends ANY
{
protected ED originalText;
protected List qualifier;
protected List translation;
@XmlAttribute(name = "code")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String code;
@XmlAttribute(name = "codeSystem")
protected String codeSystem;
@XmlAttribute(name = "codeSystemName")
protected String codeSystemName;
@XmlAttribute(name = "codeSystemVersion")
protected String codeSystemVersion;
@XmlAttribute(name = "displayName")
protected String displayName;
/**
* Gets the value of the originalText property.
*
* @return
* possible object is
* {@link ED }
*
*/
public ED getOriginalText() {
return originalText;
}
/**
* Sets the value of the originalText property.
*
* @param value
* allowed object is
* {@link ED }
*
*/
public void setOriginalText(ED value) {
this.originalText = value;
}
/**
* Gets the value of the qualifier property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the qualifier property.
*
*
* For example, to add a new item, do as follows:
*
* getQualifier().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CR }
*
*
*/
public List getQualifier() {
if (qualifier == null) {
qualifier = new ArrayList();
}
return this.qualifier;
}
/**
* Gets the value of the translation property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the translation property.
*
*
* For example, to add a new item, do as follows:
*
* getTranslation().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CD }
*
*
*/
public List getTranslation() {
if (translation == null) {
translation = new ArrayList();
}
return this.translation;
}
/**
* Gets the value of the code property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCode() {
return code;
}
/**
* Sets the value of the code property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCode(String value) {
this.code = value;
}
/**
* Gets the value of the codeSystem property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCodeSystem() {
return codeSystem;
}
/**
* Sets the value of the codeSystem property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCodeSystem(String value) {
this.codeSystem = value;
}
/**
* Gets the value of the codeSystemName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCodeSystemName() {
return codeSystemName;
}
/**
* Sets the value of the codeSystemName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCodeSystemName(String value) {
this.codeSystemName = value;
}
/**
* Gets the value of the codeSystemVersion property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCodeSystemVersion() {
return codeSystemVersion;
}
/**
* Sets the value of the codeSystemVersion property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCodeSystemVersion(String value) {
this.codeSystemVersion = value;
}
/**
* Gets the value of the displayName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDisplayName() {
return displayName;
}
/**
* Sets the value of the displayName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDisplayName(String value) {
this.displayName = value;
}
public CD withOriginalText(ED value) {
setOriginalText(value);
return this;
}
public CD withQualifier(CR... values) {
if (values!= null) {
for (CR value: values) {
getQualifier().add(value);
}
}
return this;
}
public CD withQualifier(Collection values) {
if (values!= null) {
getQualifier().addAll(values);
}
return this;
}
public CD withTranslation(CD... values) {
if (values!= null) {
for (CD value: values) {
getTranslation().add(value);
}
}
return this;
}
public CD withTranslation(Collection values) {
if (values!= null) {
getTranslation().addAll(values);
}
return this;
}
public CD withCode(String value) {
setCode(value);
return this;
}
public CD withCodeSystem(String value) {
setCodeSystem(value);
return this;
}
public CD withCodeSystemName(String value) {
setCodeSystemName(value);
return this;
}
public CD withCodeSystemVersion(String value) {
setCodeSystemVersion(value);
return this;
}
public CD withDisplayName(String value) {
setDisplayName(value);
return this;
}
@Override
public CD withNullFlavor(String... values) {
if (values!= null) {
for (String value: values) {
getNullFlavor().add(value);
}
}
return this;
}
@Override
public CD withNullFlavor(Collection values) {
if (values!= null) {
getNullFlavor().addAll(values);
}
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}