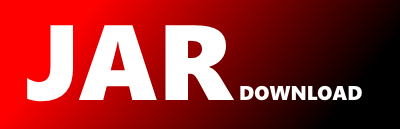
ihe.iti.svs._2008.ObjectFactory Maven / Gradle / Ivy
Show all versions of ihe-iti Show documentation
package ihe.iti.svs._2008;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the ihe.iti.svs._2008 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _RetrieveValueSetRequest_QNAME = new QName("urn:ihe:iti:svs:2008", "RetrieveValueSetRequest");
private final static QName _RetrieveValueSetResponse_QNAME = new QName("urn:ihe:iti:svs:2008", "RetrieveValueSetResponse");
private final static QName _IVLREALWidth_QNAME = new QName("urn:ihe:iti:svs:2008", "width");
private final static QName _IVLREALLow_QNAME = new QName("urn:ihe:iti:svs:2008", "low");
private final static QName _IVLREALCenter_QNAME = new QName("urn:ihe:iti:svs:2008", "center");
private final static QName _IVLREALHigh_QNAME = new QName("urn:ihe:iti:svs:2008", "high");
private final static QName _ADDeliveryInstallationQualifier_QNAME = new QName("urn:ihe:iti:svs:2008", "deliveryInstallationQualifier");
private final static QName _ADUnitID_QNAME = new QName("urn:ihe:iti:svs:2008", "unitID");
private final static QName _ADDeliveryInstallationType_QNAME = new QName("urn:ihe:iti:svs:2008", "deliveryInstallationType");
private final static QName _ADCareOf_QNAME = new QName("urn:ihe:iti:svs:2008", "careOf");
private final static QName _ADPrecinct_QNAME = new QName("urn:ihe:iti:svs:2008", "precinct");
private final static QName _ADDeliveryInstallationArea_QNAME = new QName("urn:ihe:iti:svs:2008", "deliveryInstallationArea");
private final static QName _ADDeliveryAddressLine_QNAME = new QName("urn:ihe:iti:svs:2008", "deliveryAddressLine");
private final static QName _ADStreetNameType_QNAME = new QName("urn:ihe:iti:svs:2008", "streetNameType");
private final static QName _ADDeliveryModeIdentifier_QNAME = new QName("urn:ihe:iti:svs:2008", "deliveryModeIdentifier");
private final static QName _ADAdditionalLocator_QNAME = new QName("urn:ihe:iti:svs:2008", "additionalLocator");
private final static QName _ADPostBox_QNAME = new QName("urn:ihe:iti:svs:2008", "postBox");
private final static QName _ADDirection_QNAME = new QName("urn:ihe:iti:svs:2008", "direction");
private final static QName _ADStreetAddressLine_QNAME = new QName("urn:ihe:iti:svs:2008", "streetAddressLine");
private final static QName _ADState_QNAME = new QName("urn:ihe:iti:svs:2008", "state");
private final static QName _ADDeliveryMode_QNAME = new QName("urn:ihe:iti:svs:2008", "deliveryMode");
private final static QName _ADDelimiter_QNAME = new QName("urn:ihe:iti:svs:2008", "delimiter");
private final static QName _ADUnitType_QNAME = new QName("urn:ihe:iti:svs:2008", "unitType");
private final static QName _ADStreetName_QNAME = new QName("urn:ihe:iti:svs:2008", "streetName");
private final static QName _ADUseablePeriod_QNAME = new QName("urn:ihe:iti:svs:2008", "useablePeriod");
private final static QName _ADCounty_QNAME = new QName("urn:ihe:iti:svs:2008", "county");
private final static QName _ADHouseNumber_QNAME = new QName("urn:ihe:iti:svs:2008", "houseNumber");
private final static QName _ADCensusTract_QNAME = new QName("urn:ihe:iti:svs:2008", "censusTract");
private final static QName _ADCity_QNAME = new QName("urn:ihe:iti:svs:2008", "city");
private final static QName _ADPostalCode_QNAME = new QName("urn:ihe:iti:svs:2008", "postalCode");
private final static QName _ADHouseNumberNumeric_QNAME = new QName("urn:ihe:iti:svs:2008", "houseNumberNumeric");
private final static QName _ADStreetNameBase_QNAME = new QName("urn:ihe:iti:svs:2008", "streetNameBase");
private final static QName _ADCountry_QNAME = new QName("urn:ihe:iti:svs:2008", "country");
private final static QName _ADBuildingNumberSuffix_QNAME = new QName("urn:ihe:iti:svs:2008", "buildingNumberSuffix");
private final static QName _ENFamily_QNAME = new QName("urn:ihe:iti:svs:2008", "family");
private final static QName _ENSuffix_QNAME = new QName("urn:ihe:iti:svs:2008", "suffix");
private final static QName _ENValidTime_QNAME = new QName("urn:ihe:iti:svs:2008", "validTime");
private final static QName _ENPrefix_QNAME = new QName("urn:ihe:iti:svs:2008", "prefix");
private final static QName _ENGiven_QNAME = new QName("urn:ihe:iti:svs:2008", "given");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: ihe.iti.svs._2008
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link RetrieveValueSetRequestType }
*
*/
public RetrieveValueSetRequestType createRetrieveValueSetRequestType() {
return new RetrieveValueSetRequestType();
}
/**
* Create an instance of {@link RetrieveValueSetResponseType }
*
*/
public RetrieveValueSetResponseType createRetrieveValueSetResponseType() {
return new RetrieveValueSetResponseType();
}
/**
* Create an instance of {@link ANYNonNull }
*
*/
public ANYNonNull createANYNonNull() {
return new ANYNonNull();
}
/**
* Create an instance of {@link RTOMOPQ }
*
*/
public RTOMOPQ createRTOMOPQ() {
return new RTOMOPQ();
}
/**
* Create an instance of {@link IVLPPDPQ }
*
*/
public IVLPPDPQ createIVLPPDPQ() {
return new IVLPPDPQ();
}
/**
* Create an instance of {@link AdxpPostalCode }
*
*/
public AdxpPostalCode createAdxpPostalCode() {
return new AdxpPostalCode();
}
/**
* Create an instance of {@link AdxpDeliveryInstallationArea }
*
*/
public AdxpDeliveryInstallationArea createAdxpDeliveryInstallationArea() {
return new AdxpDeliveryInstallationArea();
}
/**
* Create an instance of {@link IVLPPDTS }
*
*/
public IVLPPDTS createIVLPPDTS() {
return new IVLPPDTS();
}
/**
* Create an instance of {@link BXITCD }
*
*/
public BXITCD createBXITCD() {
return new BXITCD();
}
/**
* Create an instance of {@link RTOPQPQ }
*
*/
public RTOPQPQ createRTOPQPQ() {
return new RTOPQPQ();
}
/**
* Create an instance of {@link IVXBPPDTS }
*
*/
public IVXBPPDTS createIVXBPPDTS() {
return new IVXBPPDTS();
}
/**
* Create an instance of {@link AdxpBuildingNumberSuffix }
*
*/
public AdxpBuildingNumberSuffix createAdxpBuildingNumberSuffix() {
return new AdxpBuildingNumberSuffix();
}
/**
* Create an instance of {@link SXCMCD }
*
*/
public SXCMCD createSXCMCD() {
return new SXCMCD();
}
/**
* Create an instance of {@link IVXBPPDPQ }
*
*/
public IVXBPPDPQ createIVXBPPDPQ() {
return new IVXBPPDPQ();
}
/**
* Create an instance of {@link REAL }
*
*/
public REAL createREAL() {
return new REAL();
}
/**
* Create an instance of {@link SXCMPQ }
*
*/
public SXCMPQ createSXCMPQ() {
return new SXCMPQ();
}
/**
* Create an instance of {@link AdxpStreetAddressLine }
*
*/
public AdxpStreetAddressLine createAdxpStreetAddressLine() {
return new AdxpStreetAddressLine();
}
/**
* Create an instance of {@link AdxpDeliveryInstallationType }
*
*/
public AdxpDeliveryInstallationType createAdxpDeliveryInstallationType() {
return new AdxpDeliveryInstallationType();
}
/**
* Create an instance of {@link ValueSetRequestType }
*
*/
public ValueSetRequestType createValueSetRequestType() {
return new ValueSetRequestType();
}
/**
* Create an instance of {@link AdxpDeliveryAddressLine }
*
*/
public AdxpDeliveryAddressLine createAdxpDeliveryAddressLine() {
return new AdxpDeliveryAddressLine();
}
/**
* Create an instance of {@link EnFamily }
*
*/
public EnFamily createEnFamily() {
return new EnFamily();
}
/**
* Create an instance of {@link SXCMMO }
*
*/
public SXCMMO createSXCMMO() {
return new SXCMMO();
}
/**
* Create an instance of {@link AdxpPrecinct }
*
*/
public AdxpPrecinct createAdxpPrecinct() {
return new AdxpPrecinct();
}
/**
* Create an instance of {@link PIVLTS }
*
*/
public PIVLTS createPIVLTS() {
return new PIVLTS();
}
/**
* Create an instance of {@link IVXBPQ }
*
*/
public IVXBPQ createIVXBPQ() {
return new IVXBPQ();
}
/**
* Create an instance of {@link AdxpStreetNameBase }
*
*/
public AdxpStreetNameBase createAdxpStreetNameBase() {
return new AdxpStreetNameBase();
}
/**
* Create an instance of {@link IVXBMO }
*
*/
public IVXBMO createIVXBMO() {
return new IVXBMO();
}
/**
* Create an instance of {@link SXCMTS }
*
*/
public SXCMTS createSXCMTS() {
return new SXCMTS();
}
/**
* Create an instance of {@link EIVLEvent }
*
*/
public EIVLEvent createEIVLEvent() {
return new EIVLEvent();
}
/**
* Create an instance of {@link IVXBTS }
*
*/
public IVXBTS createIVXBTS() {
return new IVXBTS();
}
/**
* Create an instance of {@link EnPrefix }
*
*/
public EnPrefix createEnPrefix() {
return new EnPrefix();
}
/**
* Create an instance of {@link AdxpDeliveryInstallationQualifier }
*
*/
public AdxpDeliveryInstallationQualifier createAdxpDeliveryInstallationQualifier() {
return new AdxpDeliveryInstallationQualifier();
}
/**
* Create an instance of {@link TEL }
*
*/
public TEL createTEL() {
return new TEL();
}
/**
* Create an instance of {@link IVLMO }
*
*/
public IVLMO createIVLMO() {
return new IVLMO();
}
/**
* Create an instance of {@link IVLTS }
*
*/
public IVLTS createIVLTS() {
return new IVLTS();
}
/**
* Create an instance of {@link IVLPQ }
*
*/
public IVLPQ createIVLPQ() {
return new IVLPQ();
}
/**
* Create an instance of {@link EIVLPPDTS }
*
*/
public EIVLPPDTS createEIVLPPDTS() {
return new EIVLPPDTS();
}
/**
* Create an instance of {@link AdxpUnitID }
*
*/
public AdxpUnitID createAdxpUnitID() {
return new AdxpUnitID();
}
/**
* Create an instance of {@link SLISTTS }
*
*/
public SLISTTS createSLISTTS() {
return new SLISTTS();
}
/**
* Create an instance of {@link SLISTPQ }
*
*/
public SLISTPQ createSLISTPQ() {
return new SLISTPQ();
}
/**
* Create an instance of {@link ADXP }
*
*/
public ADXP createADXP() {
return new ADXP();
}
/**
* Create an instance of {@link PQR }
*
*/
public PQR createPQR() {
return new PQR();
}
/**
* Create an instance of {@link AdxpCareOf }
*
*/
public AdxpCareOf createAdxpCareOf() {
return new AdxpCareOf();
}
/**
* Create an instance of {@link IVLREAL }
*
*/
public IVLREAL createIVLREAL() {
return new IVLREAL();
}
/**
* Create an instance of {@link AdxpHouseNumber }
*
*/
public AdxpHouseNumber createAdxpHouseNumber() {
return new AdxpHouseNumber();
}
/**
* Create an instance of {@link AdxpCounty }
*
*/
public AdxpCounty createAdxpCounty() {
return new AdxpCounty();
}
/**
* Create an instance of {@link SXCMREAL }
*
*/
public SXCMREAL createSXCMREAL() {
return new SXCMREAL();
}
/**
* Create an instance of {@link EnGiven }
*
*/
public EnGiven createEnGiven() {
return new EnGiven();
}
/**
* Create an instance of {@link BXITIVLPQ }
*
*/
public BXITIVLPQ createBXITIVLPQ() {
return new BXITIVLPQ();
}
/**
* Create an instance of {@link AdxpDelimiter }
*
*/
public AdxpDelimiter createAdxpDelimiter() {
return new AdxpDelimiter();
}
/**
* Create an instance of {@link SXCMPPDPQ }
*
*/
public SXCMPPDPQ createSXCMPPDPQ() {
return new SXCMPPDPQ();
}
/**
* Create an instance of {@link AD }
*
*/
public AD createAD() {
return new AD();
}
/**
* Create an instance of {@link BL }
*
*/
public BL createBL() {
return new BL();
}
/**
* Create an instance of {@link BN }
*
*/
public BN createBN() {
return new BN();
}
/**
* Create an instance of {@link CD }
*
*/
public CD createCD() {
return new CD();
}
/**
* Create an instance of {@link CE }
*
*/
public CE createCE() {
return new CE();
}
/**
* Create an instance of {@link ConceptListType }
*
*/
public ConceptListType createConceptListType() {
return new ConceptListType();
}
/**
* Create an instance of {@link CO }
*
*/
public CO createCO() {
return new CO();
}
/**
* Create an instance of {@link CR }
*
*/
public CR createCR() {
return new CR();
}
/**
* Create an instance of {@link CS }
*
*/
public CS createCS() {
return new CS();
}
/**
* Create an instance of {@link CV }
*
*/
public CV createCV() {
return new CV();
}
/**
* Create an instance of {@link ED }
*
*/
public ED createED() {
return new ED();
}
/**
* Create an instance of {@link EN }
*
*/
public EN createEN() {
return new EN();
}
/**
* Create an instance of {@link AdxpDirection }
*
*/
public AdxpDirection createAdxpDirection() {
return new AdxpDirection();
}
/**
* Create an instance of {@link AdxpDeliveryMode }
*
*/
public AdxpDeliveryMode createAdxpDeliveryMode() {
return new AdxpDeliveryMode();
}
/**
* Create an instance of {@link II }
*
*/
public II createII() {
return new II();
}
/**
* Create an instance of {@link RTOQTYQTY }
*
*/
public RTOQTYQTY createRTOQTYQTY() {
return new RTOQTYQTY();
}
/**
* Create an instance of {@link SXCMPPDTS }
*
*/
public SXCMPPDTS createSXCMPPDTS() {
return new SXCMPPDTS();
}
/**
* Create an instance of {@link HXITPQ }
*
*/
public HXITPQ createHXITPQ() {
return new HXITPQ();
}
/**
* Create an instance of {@link MO }
*
*/
public MO createMO() {
return new MO();
}
/**
* Create an instance of {@link ON }
*
*/
public ON createON() {
return new ON();
}
/**
* Create an instance of {@link PN }
*
*/
public PN createPN() {
return new PN();
}
/**
* Create an instance of {@link PQ }
*
*/
public PQ createPQ() {
return new PQ();
}
/**
* Create an instance of {@link AdxpUnitType }
*
*/
public AdxpUnitType createAdxpUnitType() {
return new AdxpUnitType();
}
/**
* Create an instance of {@link SC }
*
*/
public SC createSC() {
return new SC();
}
/**
* Create an instance of {@link ST }
*
*/
public ST createST() {
return new ST();
}
/**
* Create an instance of {@link TN }
*
*/
public TN createTN() {
return new TN();
}
/**
* Create an instance of {@link TS }
*
*/
public TS createTS() {
return new TS();
}
/**
* Create an instance of {@link AdxpCity }
*
*/
public AdxpCity createAdxpCity() {
return new AdxpCity();
}
/**
* Create an instance of {@link IVXBINT }
*
*/
public IVXBINT createIVXBINT() {
return new IVXBINT();
}
/**
* Create an instance of {@link AdxpState }
*
*/
public AdxpState createAdxpState() {
return new AdxpState();
}
/**
* Create an instance of {@link SXCMINT }
*
*/
public SXCMINT createSXCMINT() {
return new SXCMINT();
}
/**
* Create an instance of {@link UVPTS }
*
*/
public UVPTS createUVPTS() {
return new UVPTS();
}
/**
* Create an instance of {@link AdxpStreetNameType }
*
*/
public AdxpStreetNameType createAdxpStreetNameType() {
return new AdxpStreetNameType();
}
/**
* Create an instance of {@link AdxpPostBox }
*
*/
public AdxpPostBox createAdxpPostBox() {
return new AdxpPostBox();
}
/**
* Create an instance of {@link AdxpDeliveryModeIdentifier }
*
*/
public AdxpDeliveryModeIdentifier createAdxpDeliveryModeIdentifier() {
return new AdxpDeliveryModeIdentifier();
}
/**
* Create an instance of {@link SXPRTS }
*
*/
public SXPRTS createSXPRTS() {
return new SXPRTS();
}
/**
* Create an instance of {@link INT }
*
*/
public INT createINT() {
return new INT();
}
/**
* Create an instance of {@link AdxpAdditionalLocator }
*
*/
public AdxpAdditionalLocator createAdxpAdditionalLocator() {
return new AdxpAdditionalLocator();
}
/**
* Create an instance of {@link HXITCE }
*
*/
public HXITCE createHXITCE() {
return new HXITCE();
}
/**
* Create an instance of {@link AdxpStreetName }
*
*/
public AdxpStreetName createAdxpStreetName() {
return new AdxpStreetName();
}
/**
* Create an instance of {@link IVLINT }
*
*/
public IVLINT createIVLINT() {
return new IVLINT();
}
/**
* Create an instance of {@link AdxpCountry }
*
*/
public AdxpCountry createAdxpCountry() {
return new AdxpCountry();
}
/**
* Create an instance of {@link PPDPQ }
*
*/
public PPDPQ createPPDPQ() {
return new PPDPQ();
}
/**
* Create an instance of {@link ENXP }
*
*/
public ENXP createENXP() {
return new ENXP();
}
/**
* Create an instance of {@link EnSuffix }
*
*/
public EnSuffix createEnSuffix() {
return new EnSuffix();
}
/**
* Create an instance of {@link EIVLTS }
*
*/
public EIVLTS createEIVLTS() {
return new EIVLTS();
}
/**
* Create an instance of {@link GLISTPQ }
*
*/
public GLISTPQ createGLISTPQ() {
return new GLISTPQ();
}
/**
* Create an instance of {@link ValueSetResponseType }
*
*/
public ValueSetResponseType createValueSetResponseType() {
return new ValueSetResponseType();
}
/**
* Create an instance of {@link IVXBREAL }
*
*/
public IVXBREAL createIVXBREAL() {
return new IVXBREAL();
}
/**
* Create an instance of {@link RTO }
*
*/
public RTO createRTO() {
return new RTO();
}
/**
* Create an instance of {@link GLISTTS }
*
*/
public GLISTTS createGLISTTS() {
return new GLISTTS();
}
/**
* Create an instance of {@link PIVLPPDTS }
*
*/
public PIVLPPDTS createPIVLPPDTS() {
return new PIVLPPDTS();
}
/**
* Create an instance of {@link EnDelimiter }
*
*/
public EnDelimiter createEnDelimiter() {
return new EnDelimiter();
}
/**
* Create an instance of {@link AdxpCensusTract }
*
*/
public AdxpCensusTract createAdxpCensusTract() {
return new AdxpCensusTract();
}
/**
* Create an instance of {@link AdxpHouseNumberNumeric }
*
*/
public AdxpHouseNumberNumeric createAdxpHouseNumberNumeric() {
return new AdxpHouseNumberNumeric();
}
/**
* Create an instance of {@link PPDTS }
*
*/
public PPDTS createPPDTS() {
return new PPDTS();
}
/**
* Create an instance of {@link Thumbnail }
*
*/
public Thumbnail createThumbnail() {
return new Thumbnail();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link RetrieveValueSetRequestType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "RetrieveValueSetRequest")
public JAXBElement createRetrieveValueSetRequest(RetrieveValueSetRequestType value) {
return new JAXBElement(_RetrieveValueSetRequest_QNAME, RetrieveValueSetRequestType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link RetrieveValueSetResponseType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "RetrieveValueSetResponse")
public JAXBElement createRetrieveValueSetResponse(RetrieveValueSetResponseType value) {
return new JAXBElement(_RetrieveValueSetResponse_QNAME, RetrieveValueSetResponseType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link REAL }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "width", scope = IVLREAL.class)
public JAXBElement createIVLREALWidth(REAL value) {
return new JAXBElement(_IVLREALWidth_QNAME, REAL.class, IVLREAL.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBREAL }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "low", scope = IVLREAL.class)
public JAXBElement createIVLREALLow(IVXBREAL value) {
return new JAXBElement(_IVLREALLow_QNAME, IVXBREAL.class, IVLREAL.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link REAL }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "center", scope = IVLREAL.class)
public JAXBElement createIVLREALCenter(REAL value) {
return new JAXBElement(_IVLREALCenter_QNAME, REAL.class, IVLREAL.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBREAL }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "high", scope = IVLREAL.class)
public JAXBElement createIVLREALHigh(IVXBREAL value) {
return new JAXBElement(_IVLREALHigh_QNAME, IVXBREAL.class, IVLREAL.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link INT }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "width", scope = IVLINT.class)
public JAXBElement createIVLINTWidth(INT value) {
return new JAXBElement(_IVLREALWidth_QNAME, INT.class, IVLINT.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBINT }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "low", scope = IVLINT.class)
public JAXBElement createIVLINTLow(IVXBINT value) {
return new JAXBElement(_IVLREALLow_QNAME, IVXBINT.class, IVLINT.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link INT }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "center", scope = IVLINT.class)
public JAXBElement createIVLINTCenter(INT value) {
return new JAXBElement(_IVLREALCenter_QNAME, INT.class, IVLINT.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBINT }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "high", scope = IVLINT.class)
public JAXBElement createIVLINTHigh(IVXBINT value) {
return new JAXBElement(_IVLREALHigh_QNAME, IVXBINT.class, IVLINT.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PQ }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "width", scope = IVLPQ.class)
public JAXBElement createIVLPQWidth(PQ value) {
return new JAXBElement(_IVLREALWidth_QNAME, PQ.class, IVLPQ.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBPQ }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "low", scope = IVLPQ.class)
public JAXBElement createIVLPQLow(IVXBPQ value) {
return new JAXBElement(_IVLREALLow_QNAME, IVXBPQ.class, IVLPQ.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PQ }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "center", scope = IVLPQ.class)
public JAXBElement createIVLPQCenter(PQ value) {
return new JAXBElement(_IVLREALCenter_QNAME, PQ.class, IVLPQ.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBPQ }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "high", scope = IVLPQ.class)
public JAXBElement createIVLPQHigh(IVXBPQ value) {
return new JAXBElement(_IVLREALHigh_QNAME, IVXBPQ.class, IVLPQ.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PPDPQ }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "width", scope = IVLPPDPQ.class)
public JAXBElement createIVLPPDPQWidth(PPDPQ value) {
return new JAXBElement(_IVLREALWidth_QNAME, PPDPQ.class, IVLPPDPQ.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBPPDPQ }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "low", scope = IVLPPDPQ.class)
public JAXBElement createIVLPPDPQLow(IVXBPPDPQ value) {
return new JAXBElement(_IVLREALLow_QNAME, IVXBPPDPQ.class, IVLPPDPQ.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PPDPQ }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "center", scope = IVLPPDPQ.class)
public JAXBElement createIVLPPDPQCenter(PPDPQ value) {
return new JAXBElement(_IVLREALCenter_QNAME, PPDPQ.class, IVLPPDPQ.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBPPDPQ }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "high", scope = IVLPPDPQ.class)
public JAXBElement createIVLPPDPQHigh(IVXBPPDPQ value) {
return new JAXBElement(_IVLREALHigh_QNAME, IVXBPPDPQ.class, IVLPPDPQ.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpDeliveryInstallationQualifier }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "deliveryInstallationQualifier", scope = AD.class)
public JAXBElement createADDeliveryInstallationQualifier(AdxpDeliveryInstallationQualifier value) {
return new JAXBElement(_ADDeliveryInstallationQualifier_QNAME, AdxpDeliveryInstallationQualifier.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpUnitID }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "unitID", scope = AD.class)
public JAXBElement createADUnitID(AdxpUnitID value) {
return new JAXBElement(_ADUnitID_QNAME, AdxpUnitID.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpDeliveryInstallationType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "deliveryInstallationType", scope = AD.class)
public JAXBElement createADDeliveryInstallationType(AdxpDeliveryInstallationType value) {
return new JAXBElement(_ADDeliveryInstallationType_QNAME, AdxpDeliveryInstallationType.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpCareOf }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "careOf", scope = AD.class)
public JAXBElement createADCareOf(AdxpCareOf value) {
return new JAXBElement(_ADCareOf_QNAME, AdxpCareOf.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpPrecinct }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "precinct", scope = AD.class)
public JAXBElement createADPrecinct(AdxpPrecinct value) {
return new JAXBElement(_ADPrecinct_QNAME, AdxpPrecinct.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpDeliveryInstallationArea }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "deliveryInstallationArea", scope = AD.class)
public JAXBElement createADDeliveryInstallationArea(AdxpDeliveryInstallationArea value) {
return new JAXBElement(_ADDeliveryInstallationArea_QNAME, AdxpDeliveryInstallationArea.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpDeliveryAddressLine }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "deliveryAddressLine", scope = AD.class)
public JAXBElement createADDeliveryAddressLine(AdxpDeliveryAddressLine value) {
return new JAXBElement(_ADDeliveryAddressLine_QNAME, AdxpDeliveryAddressLine.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpStreetNameType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "streetNameType", scope = AD.class)
public JAXBElement createADStreetNameType(AdxpStreetNameType value) {
return new JAXBElement(_ADStreetNameType_QNAME, AdxpStreetNameType.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpDeliveryModeIdentifier }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "deliveryModeIdentifier", scope = AD.class)
public JAXBElement createADDeliveryModeIdentifier(AdxpDeliveryModeIdentifier value) {
return new JAXBElement(_ADDeliveryModeIdentifier_QNAME, AdxpDeliveryModeIdentifier.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpAdditionalLocator }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "additionalLocator", scope = AD.class)
public JAXBElement createADAdditionalLocator(AdxpAdditionalLocator value) {
return new JAXBElement(_ADAdditionalLocator_QNAME, AdxpAdditionalLocator.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpPostBox }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "postBox", scope = AD.class)
public JAXBElement createADPostBox(AdxpPostBox value) {
return new JAXBElement(_ADPostBox_QNAME, AdxpPostBox.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpDirection }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "direction", scope = AD.class)
public JAXBElement createADDirection(AdxpDirection value) {
return new JAXBElement(_ADDirection_QNAME, AdxpDirection.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpStreetAddressLine }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "streetAddressLine", scope = AD.class)
public JAXBElement createADStreetAddressLine(AdxpStreetAddressLine value) {
return new JAXBElement(_ADStreetAddressLine_QNAME, AdxpStreetAddressLine.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpState }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "state", scope = AD.class)
public JAXBElement createADState(AdxpState value) {
return new JAXBElement(_ADState_QNAME, AdxpState.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpDeliveryMode }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "deliveryMode", scope = AD.class)
public JAXBElement createADDeliveryMode(AdxpDeliveryMode value) {
return new JAXBElement(_ADDeliveryMode_QNAME, AdxpDeliveryMode.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpDelimiter }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "delimiter", scope = AD.class)
public JAXBElement createADDelimiter(AdxpDelimiter value) {
return new JAXBElement(_ADDelimiter_QNAME, AdxpDelimiter.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpUnitType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "unitType", scope = AD.class)
public JAXBElement createADUnitType(AdxpUnitType value) {
return new JAXBElement(_ADUnitType_QNAME, AdxpUnitType.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpStreetName }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "streetName", scope = AD.class)
public JAXBElement createADStreetName(AdxpStreetName value) {
return new JAXBElement(_ADStreetName_QNAME, AdxpStreetName.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SXCMTS }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "useablePeriod", scope = AD.class)
public JAXBElement createADUseablePeriod(SXCMTS value) {
return new JAXBElement(_ADUseablePeriod_QNAME, SXCMTS.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpCounty }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "county", scope = AD.class)
public JAXBElement createADCounty(AdxpCounty value) {
return new JAXBElement(_ADCounty_QNAME, AdxpCounty.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpHouseNumber }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "houseNumber", scope = AD.class)
public JAXBElement createADHouseNumber(AdxpHouseNumber value) {
return new JAXBElement(_ADHouseNumber_QNAME, AdxpHouseNumber.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpCensusTract }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "censusTract", scope = AD.class)
public JAXBElement createADCensusTract(AdxpCensusTract value) {
return new JAXBElement(_ADCensusTract_QNAME, AdxpCensusTract.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpCity }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "city", scope = AD.class)
public JAXBElement createADCity(AdxpCity value) {
return new JAXBElement(_ADCity_QNAME, AdxpCity.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpPostalCode }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "postalCode", scope = AD.class)
public JAXBElement createADPostalCode(AdxpPostalCode value) {
return new JAXBElement(_ADPostalCode_QNAME, AdxpPostalCode.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpHouseNumberNumeric }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "houseNumberNumeric", scope = AD.class)
public JAXBElement createADHouseNumberNumeric(AdxpHouseNumberNumeric value) {
return new JAXBElement(_ADHouseNumberNumeric_QNAME, AdxpHouseNumberNumeric.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpStreetNameBase }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "streetNameBase", scope = AD.class)
public JAXBElement createADStreetNameBase(AdxpStreetNameBase value) {
return new JAXBElement(_ADStreetNameBase_QNAME, AdxpStreetNameBase.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpCountry }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "country", scope = AD.class)
public JAXBElement createADCountry(AdxpCountry value) {
return new JAXBElement(_ADCountry_QNAME, AdxpCountry.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AdxpBuildingNumberSuffix }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "buildingNumberSuffix", scope = AD.class)
public JAXBElement createADBuildingNumberSuffix(AdxpBuildingNumberSuffix value) {
return new JAXBElement(_ADBuildingNumberSuffix_QNAME, AdxpBuildingNumberSuffix.class, AD.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PQ }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "width", scope = IVLTS.class)
public JAXBElement createIVLTSWidth(PQ value) {
return new JAXBElement(_IVLREALWidth_QNAME, PQ.class, IVLTS.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBTS }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "low", scope = IVLTS.class)
public JAXBElement createIVLTSLow(IVXBTS value) {
return new JAXBElement(_IVLREALLow_QNAME, IVXBTS.class, IVLTS.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TS }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "center", scope = IVLTS.class)
public JAXBElement createIVLTSCenter(TS value) {
return new JAXBElement(_IVLREALCenter_QNAME, TS.class, IVLTS.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBTS }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "high", scope = IVLTS.class)
public JAXBElement createIVLTSHigh(IVXBTS value) {
return new JAXBElement(_IVLREALHigh_QNAME, IVXBTS.class, IVLTS.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PPDPQ }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "width", scope = IVLPPDTS.class)
public JAXBElement createIVLPPDTSWidth(PPDPQ value) {
return new JAXBElement(_IVLREALWidth_QNAME, PPDPQ.class, IVLPPDTS.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBPPDTS }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "low", scope = IVLPPDTS.class)
public JAXBElement createIVLPPDTSLow(IVXBPPDTS value) {
return new JAXBElement(_IVLREALLow_QNAME, IVXBPPDTS.class, IVLPPDTS.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PPDTS }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "center", scope = IVLPPDTS.class)
public JAXBElement createIVLPPDTSCenter(PPDTS value) {
return new JAXBElement(_IVLREALCenter_QNAME, PPDTS.class, IVLPPDTS.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBPPDTS }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "high", scope = IVLPPDTS.class)
public JAXBElement createIVLPPDTSHigh(IVXBPPDTS value) {
return new JAXBElement(_IVLREALHigh_QNAME, IVXBPPDTS.class, IVLPPDTS.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link EnFamily }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "family", scope = EN.class)
public JAXBElement createENFamily(EnFamily value) {
return new JAXBElement(_ENFamily_QNAME, EnFamily.class, EN.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link EnSuffix }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "suffix", scope = EN.class)
public JAXBElement createENSuffix(EnSuffix value) {
return new JAXBElement(_ENSuffix_QNAME, EnSuffix.class, EN.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVLTS }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "validTime", scope = EN.class)
public JAXBElement createENValidTime(IVLTS value) {
return new JAXBElement(_ENValidTime_QNAME, IVLTS.class, EN.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link EnDelimiter }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "delimiter", scope = EN.class)
public JAXBElement createENDelimiter(EnDelimiter value) {
return new JAXBElement(_ADDelimiter_QNAME, EnDelimiter.class, EN.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link EnPrefix }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "prefix", scope = EN.class)
public JAXBElement createENPrefix(EnPrefix value) {
return new JAXBElement(_ENPrefix_QNAME, EnPrefix.class, EN.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link EnGiven }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "given", scope = EN.class)
public JAXBElement createENGiven(EnGiven value) {
return new JAXBElement(_ENGiven_QNAME, EnGiven.class, EN.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link MO }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "width", scope = IVLMO.class)
public JAXBElement createIVLMOWidth(MO value) {
return new JAXBElement(_IVLREALWidth_QNAME, MO.class, IVLMO.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBMO }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "low", scope = IVLMO.class)
public JAXBElement createIVLMOLow(IVXBMO value) {
return new JAXBElement(_IVLREALLow_QNAME, IVXBMO.class, IVLMO.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link MO }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "center", scope = IVLMO.class)
public JAXBElement createIVLMOCenter(MO value) {
return new JAXBElement(_IVLREALCenter_QNAME, MO.class, IVLMO.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link IVXBMO }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:ihe:iti:svs:2008", name = "high", scope = IVLMO.class)
public JAXBElement createIVLMOHigh(IVXBMO value) {
return new JAXBElement(_IVLREALHigh_QNAME, IVXBMO.class, IVLMO.class, value);
}
}