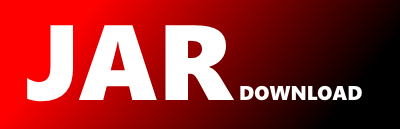
com.github.rapidark.preloader.facade.ServletFacade Maven / Gradle / Ivy
The newest version!
package com.github.rapidark.preloader.facade;
import java.io.IOException;
import java.util.HashMap;
import javax.servlet.Servlet;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.github.rapidark.preloader.PreClassLoader;
import com.github.rapidark.preloader.Reloader;
public class ServletFacade extends HttpServlet {
private static final long serialVersionUID = 1L;
private ServletConfig config;
private Servlet servlet;
private static HashMap instances = new HashMap();
public static HashMap getInstances() {
return instances;
}
public void init(ServletConfig config) throws ServletException {
this.config = config;
loadServlet();
instances.put(config.getInitParameter("class"), this);
}
public void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
if ((this.servlet != null) && (!Reloader.isReloading)) {
this.servlet.service(request, response);
}
}
public void unloadClass() {
if (this.servlet != null) {
try {
this.servlet.destroy();
} catch (Throwable localThrowable) {
}
}
this.servlet = null;
}
public void loadServlet() throws ServletException {
String className = this.config.getInitParameter("class");
if (className == null) {
return;
}
synchronized (this) {
try {
Class> clazz = PreClassLoader.load(className);
if ((clazz == null) || (!Servlet.class.isAssignableFrom(clazz))) {
throw new ServletException("Class " + className + " not found or not a servlet!");
}
this.servlet = ((Servlet) clazz.newInstance());
this.servlet.init(this.config);
} catch (Exception e) {
e.printStackTrace();
}
}
}
public void destroy() {
if (this.servlet != null) {
this.servlet.destroy();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy