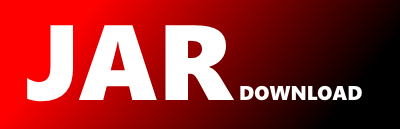
com.github.rauberprojects.client.context.DefaultContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of client-core Show documentation
Show all versions of client-core Show documentation
Reusable core classes for any client implementation.
package com.github.rauberprojects.client.context;
import com.github.rauberprojects.client.model.Uber;
import com.github.rauberprojects.client.profile.Profile;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
public class DefaultContext implements Context {
private static final Logger logger = LoggerFactory.getLogger(DefaultContext.class);
private final Uber uber;
private final List profiles = new ArrayList();
public DefaultContext(Uber uber) {
this.uber = uber;
}
/**
* Copy-Constructor
* @param context
*/
public DefaultContext(Context context) {
this.uber = new Uber(context.getUber());
this.profiles.addAll(context.getActiveProfiles());
}
@Override
public Uber getUber() {
return uber;
}
@Override
public Collection getActiveProfiles() {
return Collections.unmodifiableCollection(profiles);
}
public DefaultContext addActiveProfile(Profile profile) {
final DefaultContext clone = new DefaultContext(this);
clone.addActiveProfile(profile);
return clone;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof DefaultContext)) return false;
DefaultContext that = (DefaultContext) o;
if (uber != null ? !uber.equals(that.uber) : that.uber != null) return false;
return true;
}
@Override
public int hashCode() {
return uber != null ? uber.hashCode() : 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy