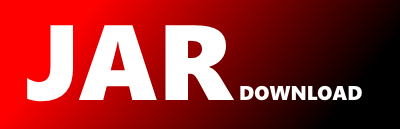
com.github.resource4j.extras.config.ConfigMapParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resource4j-extras Show documentation
Show all versions of resource4j-extras Show documentation
Integration of Resource4J with various parsers and other addons.
package com.github.resource4j.extras.config;
import static com.github.resource4j.extras.config.ConfigParser.config;
import static com.typesafe.config.ConfigParseOptions.defaults;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import com.github.resource4j.ResourceObject;
import com.github.resource4j.ResourceObjectException;
import com.github.resource4j.objects.parsers.AbstractValueParser;
import com.github.resource4j.objects.parsers.BundleParser;
import com.github.resource4j.resources.discovery.ContentType;
import com.typesafe.config.Config;
import com.typesafe.config.ConfigParseOptions;
import com.typesafe.config.ConfigSyntax;
import com.typesafe.config.ConfigValue;
@SuppressWarnings("WeakerAccess")
@ContentType(extension = ".config", mimeType = "text/x-java-properties")
public class ConfigMapParser extends AbstractValueParser
© 2015 - 2025 Weber Informatics LLC | Privacy Policy