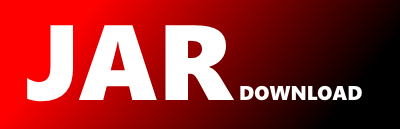
com.github.resource4j.extras.json.JacksonBundleParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resource4j-extras Show documentation
Show all versions of resource4j-extras Show documentation
Integration of Resource4J with various parsers and other addons.
package com.github.resource4j.extras.json;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.JsonNodeType;
import com.github.resource4j.ResourceKey;
import com.github.resource4j.ResourceObject;
import com.github.resource4j.ResourceObjectException;
import com.github.resource4j.objects.parsers.AbstractValueParser;
import com.github.resource4j.objects.parsers.BundleParser;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import static com.github.resource4j.extras.json.JacksonParser.json;
public class JacksonBundleParser extends AbstractValueParser
© 2015 - 2025 Weber Informatics LLC | Privacy Policy