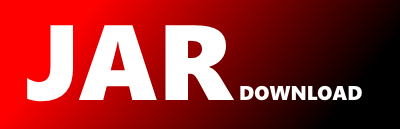
com.github.rexsheng.springboot.faster.system.auth.adapter.SecurityConfig Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.auth.adapter;
import com.github.rexsheng.springboot.faster.system.auth.application.AuthService;
import com.github.rexsheng.springboot.faster.system.auth.application.security.*;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.boot.autoconfigure.security.SecurityProperties;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.HttpMethod;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.authentication.ProviderManager;
import org.springframework.security.config.annotation.ObjectPostProcessor;
import org.springframework.security.config.annotation.method.configuration.EnableMethodSecurity;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.crypto.factory.PasswordEncoderFactories;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.web.SecurityFilterChain;
import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter;
import org.springframework.security.web.authentication.www.BasicAuthenticationConverter;
import org.springframework.security.web.authentication.www.BasicAuthenticationFilter;
import org.springframework.security.web.util.matcher.AntPathRequestMatcher;
import org.springframework.security.web.util.matcher.RequestMatcher;
import org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping;
@Configuration
@EnableWebSecurity
@EnableMethodSecurity
@EnableConfigurationProperties(TokenExpireProperties.class)
@ConditionalOnClass(SecurityFilterChain.class)
public class SecurityConfig {
/**
* @see org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter
* @see org.springframework.security.web.authentication.www.BasicAuthenticationFilter
* @see org.springframework.security.web.access.intercept.AuthorizationFilter
* @see org.springframework.security.access.expression.SecurityExpressionOperations
* @param http
* @param ignoredUrlsProperties
* @param authService
* @return
* @throws Exception
*/
@Bean
public SecurityFilterChain filterChain(HttpSecurity http,
IgnoredUrlsProperties ignoredUrlsProperties,
AuthService authService,
RequestMappingHandlerMapping requestMappingHandlerMapping,
AuthenticationManager authenticationManager) throws Exception{
http.authenticationManager(authenticationManager);
RequestMatcher ignoredMatcher = ignoredUrlsProperties.getUrlRequestMatcher();
RestHttpAuthenticationDetailsSource detailsSource=new RestHttpAuthenticationDetailsSource();
RequestHeaderTokenConverter headerTokenConverter=new RequestHeaderTokenConverter();
http.csrf((csrf)->csrf.disable()).cors(corConfig->{})
.sessionManagement(session->session.sessionCreationPolicy(SessionCreationPolicy.STATELESS))
.authorizeHttpRequests(authorize->authorize.requestMatchers(ignoredMatcher).permitAll()
.anyRequest().authenticated())
.httpBasic(httpSecurityHttpBasicConfigurer -> {
httpSecurityHttpBasicConfigurer.withObjectPostProcessor(new ObjectPostProcessor() {
@Override
public O postProcess(O filter) {
filter.setAuthenticationConverter(new BasicApiAuthenticationConverter());
return filter;
}
});
})
.securityMatcher(HttpHeaderAuthorizationConfigurer.customizerRequestMatcher(requestMappingHandlerMapping))
.with(HttpHeaderAuthorizationConfigurer.instance(), authorize->authorize
.authenticationDetailsSource(detailsSource)
.requestHeaderTokenConverter(headerTokenConverter)
.ignoreUrls(ignoredMatcher)
.enableRefreshToken()
// .withObjectPostProcessor(new ObjectPostProcessor() {
// @Override
// public O postProcess(O object) {
// return object;
// }
// })
)
.formLogin(form->form
.loginProcessingUrl("/token")
.usernameParameter("username")
.passwordParameter("password")
.successHandler(new RestHttpAuthenticationSuccessHandler())
.failureHandler(new RestHttpAuthenticationFailureHandler())
.authenticationDetailsSource(detailsSource))
.logout(log->log.logoutRequestMatcher(new AntPathRequestMatcher("/logout", HttpMethod.POST.name()))
.logoutSuccessHandler(new RestHttpLogoutSuccessHandler())
.addLogoutHandler(new RestHttpLogoutHandler(authService,headerTokenConverter)))
.exceptionHandling(exception->exception.authenticationEntryPoint(new RestHttpAuthenticationEntryPoint())
.accessDeniedHandler(new RestHttpAccessDeniedHandler()))
;
return http.build();
}
@Bean
public PasswordEncoder passwordEncoder(){
return PasswordEncoderFactories.createDelegatingPasswordEncoder();
}
@Bean
public AuthenticationManager authenticationManager(
AuthService authService, SecurityProperties securityProperties) {
BasicApiAuthenticationProvider tokenAuthenticationProvider = new BasicApiAuthenticationProvider(authService,securityProperties);
MultiLoginParamAuthenticationProvider authenticationProvider = new MultiLoginParamAuthenticationProvider(authService);
ProviderManager providerManager = new ProviderManager(tokenAuthenticationProvider,authenticationProvider);
providerManager.setEraseCredentialsAfterAuthentication(true);
return providerManager;
}
@Bean("ss")
public PermissionChecker permissionChecker(){
return new PermissionChecker();
}
// @Autowired
// public void configure(AuthenticationManagerBuilder builder,AuthService authService) {
// MultiLoginParamAuthenticationProvider authenticationProvider = new MultiLoginParamAuthenticationProvider(authService);
// ApiTokenAuthenticationProvider tokenAuthenticationProvider = new ApiTokenAuthenticationProvider(authService);
// builder.authenticationProvider(authenticationProvider);
// builder.authenticationProvider(tokenAuthenticationProvider);
// builder.eraseCredentials(true);
// }
// @Bean
// public WebSecurityCustomizer webSecurityCustomizer() {
// // 忽略 /error 页面
// return web -> web.ignoring().requestMatchers("/error",
// // 忽略 swagger 接口路径
// "/swagger-ui/**", "/v3/api-docs/**", "/swagger-ui.html")
// .requestMatchers(PathRequest.toStaticResources().at(StaticResourceLocation.FAVICON))
// // 忽略常见的静态资源路径
//// .requestMatchers(PathRequest.toStaticResources().atCommonLocations())
// ;
// }
// @Bean
// public FilterRegistrationBean preAuthorizationFilterRegistration(HttpHeaderAuthorizationFilter httpHeaderAuthorizationFilter) {
// FilterRegistrationBean registration = new FilterRegistrationBean<>(httpHeaderAuthorizationFilter);
// registration.setEnabled(false);
// return registration;
// }
// @Bean
// public AuthenticationManager authenticationManager(
// UserDetailsService userDetailsService,
// PasswordEncoder passwordEncoder) {
// DaoAuthenticationProvider authenticationProvider = new DaoAuthenticationProvider();
// authenticationProvider.setUserDetailsService(userDetailsService);
// authenticationProvider.setPasswordEncoder(passwordEncoder);
//
// ProviderManager providerManager = new ProviderManager(authenticationProvider);
// providerManager.setEraseCredentialsAfterAuthentication(false);
//
// return providerManager;
// }
//
// @Bean
// public UserDetailsService userDetailsService() {
// UserDetails userDetails = User.withDefaultPasswordEncoder()
// .username("user")
// .password("password")
// .roles("USER")
// .build();
//
// return new InMemoryUserDetailsManager(userDetails);
// }
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy