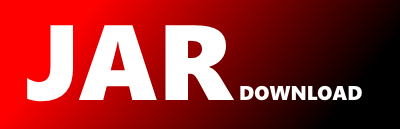
com.github.rexsheng.springboot.faster.system.cache.CaffeineConfig Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.cache;
import com.github.benmanes.caffeine.cache.*;
import com.github.rexsheng.springboot.faster.system.entity.User;
import com.github.rexsheng.springboot.faster.system.modular.SpringModule;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.context.annotation.Bean;
import java.time.Duration;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.UUID;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
@ConditionalOnClass(Caffeine.class)
//@SpringModule
public class CaffeineConfig {
private static final Logger logger= LoggerFactory.getLogger(CaffeineConfig.class);
// @Bean
// public CacheManager cacheManager(){
// CaffeineCacheManager caffeineCacheManager=new CaffeineCacheManager();
// caffeineCacheManager.setCaffeine(Caffeine.newBuilder().expireAfterWrite(Duration.ofSeconds(20)).maximumSize(200));
// return caffeineCacheManager;
// }
//
// @Bean
// public CacheManagerCustomizer caffeineCacheManagerCustomizer(){
// return cacheManager -> {
//
// };
// }
@Bean("caffeineCache")
@ConditionalOnMissingBean(name = "caffeineCache")
public Cache caffeineCache(){
Cache cache = Caffeine.newBuilder()
.expireAfterWrite(Duration.ofSeconds(20))
// .refreshAfterWrite(Duration.ofSeconds(5))
.initialCapacity(100)
.maximumSize(200)
.recordStats()
.evictionListener(new RemovalListener() {
@Override
public void onRemoval(@Nullable String key, @Nullable Object value, RemovalCause cause) {
logger.info("evict Listener key:{},value:{},cause:{}",key,value,cause);
}
})
.removalListener((key, value, cause) -> {
logger.info("remove Listener key:{},value:{},cause:{}",key,value,cause);
})
.build();
return cache;
}
@Bean("loadingCaffeineCache")
@ConditionalOnMissingBean(name = "loadingCaffeineCache")
public LoadingCache loadingCaffeineCache(){
LoadingCache cache = Caffeine.newBuilder()
.expireAfterWrite(Duration.ofSeconds(20))
// .refreshAfterWrite(Duration.ofSeconds(5))
.initialCapacity(100)
.maximumSize(200)
.build(new CacheLoader() {
@Override
public @Nullable Object load(String key) throws Exception {
User user=new User();
user.setId(1L);
user.setName(key);
return user;
}
@Override
public Map extends String, ?> loadAll(Set extends String> keys) throws Exception {
Map map=new HashMap<>();
for(String key:keys){
User user=new User();
user.setId(-1L);
user.setName(key);
map.put(key,user);
}
return map;
}
});
return cache;
}
@Bean("asyncCaffeineCache")
@ConditionalOnMissingBean(name = "asyncCaffeineCache")
public AsyncCache asyncCaffeineCache() throws ExecutionException, InterruptedException {
AsyncCache cache = Caffeine.newBuilder()
.expireAfterWrite(Duration.ofSeconds(20))
// .refreshAfterWrite(Duration.ofSeconds(5))
.initialCapacity(100)
.maximumSize(200)
.buildAsync();
CompletableFuture
© 2015 - 2024 Weber Informatics LLC | Privacy Policy