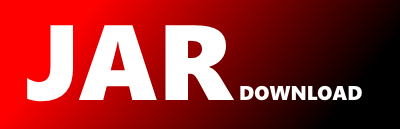
com.github.rexsheng.springboot.faster.system.config.AsyncConfig Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.config;
import com.github.rexsheng.springboot.faster.io.MixPropertySourceFactory;
import com.github.rexsheng.springboot.faster.logging.RequestLogFactory;
import com.github.rexsheng.springboot.faster.system.modular.SpringModule;
import org.slf4j.MDC;
import org.springframework.boot.task.ThreadPoolTaskExecutorCustomizer;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
import org.springframework.core.task.TaskDecorator;
import org.springframework.core.task.TaskExecutor;
import org.springframework.scheduling.annotation.EnableAsync;
import org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor;
import org.springframework.security.core.context.SecurityContext;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.web.context.request.RequestAttributes;
import org.springframework.web.context.request.RequestContextHolder;
import java.util.Map;
import java.util.concurrent.ThreadPoolExecutor;
@Configuration
@EnableAsync
@SpringModule
public class AsyncConfig implements ThreadPoolTaskExecutorCustomizer{
/**
* 核心线程数(线程池维护线程的最小数量)
*/
private int corePoolSize = 10;
/**
* 最大线程数(线程池维护线程的最大数量)
*/
private int maxPoolSize = 200;
/**
* 队列最大长度
*/
private int queueCapacity = 50;
// @Bean
// public ThreadPoolTaskExecutorCustomizer taskExecutor() {
//// org.springframework.boot.autoconfigure.task.TaskExecutionAutoConfiguration a;
//
// ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
// executor.setCorePoolSize(corePoolSize);
// executor.setMaxPoolSize(maxPoolSize);
// executor.setQueueCapacity(queueCapacity);
// executor.setThreadNamePrefix("Async-");
// // for passing in request scope context 转换请求范围的上下文
// executor.setTaskDecorator(new ContextCopyingDecorator());
// // rejection-policy:当pool已经达到max size的时候,如何处理新任务
// // CALLER_RUNS:不在新线程中执行任务,而是有调用者所在的线程来执行
// executor.setRejectedExecutionHandler(new ThreadPoolExecutor.CallerRunsPolicy());
// executor.setWaitForTasksToCompleteOnShutdown(true);
// executor.initialize();
// return executor;
// }
@Override
public void customize(ThreadPoolTaskExecutor executor) {
executor.setCorePoolSize(corePoolSize);
executor.setMaxPoolSize(maxPoolSize);
executor.setQueueCapacity(queueCapacity);
executor.setThreadNamePrefix("Async-");
// for passing in request scope context 转换请求范围的上下文
executor.setTaskDecorator(new ContextCopyingDecorator());
// rejection-policy:当pool已经达到max size的时候,如何处理新任务
// CALLER_RUNS:不在新线程中执行任务,而是有调用者所在的线程来执行
executor.setRejectedExecutionHandler(new ThreadPoolExecutor.CallerRunsPolicy());
executor.setWaitForTasksToCompleteOnShutdown(true);
}
public static class ContextCopyingDecorator implements TaskDecorator {
@Override
public Runnable decorate(Runnable runnable) {
try {
// 从父线程中获取上下文,然后应用到子线程中
RequestAttributes requestAttributes = RequestContextHolder.currentRequestAttributes();
Map previous = MDC.getCopyOfContextMap();
SecurityContext securityContext = SecurityContextHolder.getContext();
return () -> {
try {
if (previous == null) {
MDC.clear();
} else {
MDC.setContextMap(previous);
}
RequestContextHolder.setRequestAttributes(requestAttributes);
SecurityContextHolder.setContext(securityContext);
runnable.run();
} finally {
// 清除请求数据
MDC.clear();
RequestContextHolder.resetRequestAttributes();
SecurityContextHolder.clearContext();
}
};
} catch (IllegalStateException e) {
return runnable;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy