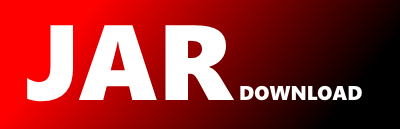
com.github.rexsheng.springboot.faster.system.dept.application.DeptServiceImpl Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.dept.application;
import com.github.rexsheng.springboot.faster.common.constant.CommonConstant;
import com.github.rexsheng.springboot.faster.spring.SpringContext;
import com.github.rexsheng.springboot.faster.system.dept.application.dto.AddDeptRequest;
import com.github.rexsheng.springboot.faster.system.dept.application.dto.DeptDetailResponse;
import com.github.rexsheng.springboot.faster.system.dept.application.dto.QueryDeptRequest;
import com.github.rexsheng.springboot.faster.system.dept.application.dto.UpdateDeptRequest;
import com.github.rexsheng.springboot.faster.system.dept.domain.DeptStateChangedEvent;
import com.github.rexsheng.springboot.faster.system.dept.domain.SysDept;
import com.github.rexsheng.springboot.faster.system.dept.domain.gateway.DeptGateway;
import com.github.rexsheng.springboot.faster.system.dept.domain.gateway.QueryDeptDO;
import jakarta.annotation.Resource;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.stereotype.Service;
import org.springframework.util.Assert;
import java.util.List;
import java.util.stream.Collectors;
@Service
@ConditionalOnClass(SqlSessionFactoryBean.class)
public class DeptServiceImpl implements DeptService{
@Resource
private DeptGateway deptGateway;
@Override
public void add(AddDeptRequest request) {
deptGateway.insertDept(request.toDept());
SpringContext.getApplicationContext().publishEvent(new DeptStateChangedEvent(DeptStateChangedEvent.DeptState.ADD));
}
@Override
public List queryList(QueryDeptRequest request) {
QueryDeptDO queryDeptDO=new QueryDeptDO();
queryDeptDO.setStatus(request.getStatus());
queryDeptDO.setName(request.getDeptName());
List deptList=deptGateway.queryDepts(queryDeptDO);
if(Boolean.TRUE.equals(request.getExcludeNonParent())){
List sources=deptList.stream()
.filter(source->source.getParentId()==null || deptList.stream().anyMatch(a->a.getDeptId().equals(source.getParentId())))
.collect(Collectors.toList());
return SysDept.mapTree(sources).stream().map(DeptDetailResponse::of).collect(Collectors.toList());
}
return SysDept.mapTree(deptList).stream().map(DeptDetailResponse::of).collect(Collectors.toList());
}
@Override
public List queryWithParent(Integer id) {
QueryDeptDO queryDeptDO=new QueryDeptDO();
queryDeptDO.setStatus(CommonConstant.STATUS_RUNNING);
List deptList=deptGateway.queryDepts(queryDeptDO);
List deptAndParentList=SysDept.getParents(deptList,id);
return deptAndParentList.stream().map(DeptDetailResponse::of).collect(Collectors.toList());
}
@Override
public List queryWithChildren(Integer id) {
QueryDeptDO queryDeptDO=new QueryDeptDO();
queryDeptDO.setStatus(CommonConstant.STATUS_RUNNING);
List deptList=deptGateway.queryDepts(queryDeptDO);
List deptAndChildrenList=SysDept.getChildrens(deptList,id);
return deptAndChildrenList.stream().map(DeptDetailResponse::of).collect(Collectors.toList());
}
@Override
public DeptDetailResponse get(Integer id) {
SysDept dept= deptGateway.getDept(id);
Assert.notNull(dept,"部门不存在");
return DeptDetailResponse.of(dept);
}
@Override
public void update(UpdateDeptRequest request) {
SysDept dept=request.toDept();
deptGateway.updateDeptById(dept);
}
@Override
public void updateStatus(List request) {
List deptList=request.stream().map(UpdateDeptRequest::toDept).collect(Collectors.toList());
deptGateway.updateDeptStatus(deptList);
SpringContext.getApplicationContext().publishEvent(new DeptStateChangedEvent(DeptStateChangedEvent.DeptState.STATUS_CHANGED));
}
@Override
public void delete(List ids) {
deptGateway.deleteDepts(SysDept.of(ids,true));
SpringContext.getApplicationContext().publishEvent(new DeptStateChangedEvent(DeptStateChangedEvent.DeptState.DELETE));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy