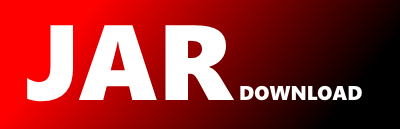
com.github.rexsheng.springboot.faster.system.dept.domain.SysDept Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.dept.domain;
import com.github.rexsheng.springboot.faster.system.utils.AuthenticationUtil;
import com.github.rexsheng.springboot.faster.util.DateUtil;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class SysDept {
private Integer deptId;
private String deptName;
private Integer parentId;
private Integer deptOrder;
private Integer status;
private Boolean isDel;
private LocalDateTime createTime;
private Long createUserId;
private LocalDateTime updateTime;
private Long updateUserId;
private List children;
public static List of(List ids, Boolean isDel){
return ids.stream().map(a->{
SysDept sysDept=new SysDept();
sysDept.setDeptId(a);
sysDept.setDel(isDel);
sysDept.setUpdateTime(DateUtil.currentDateTime());
sysDept.setUpdateUserId(AuthenticationUtil.currentUserId());
return sysDept;
}).collect(Collectors.toList());
}
public static List mapTreeByParentId(List sourceList, Integer parentId){
List dataList=sourceList.stream()
.filter(a->parentId==null?a.getParentId()==null:parentId.equals(a.getParentId()))
.peek(dept->dept.setChildren(SysDept.mapTreeByParentId(sourceList,dept.getDeptId()))).collect(Collectors.toList());
return dataList;
}
public static List mapTree(List sourceList){
List dataList=new ArrayList<>();
for(SysDept source:sourceList){
if(source.getParentId()==null || !sourceList.stream().anyMatch(a->a.getDeptId().equals(source.getParentId()))){
source.setChildren(SysDept.mapTreeByParentId(sourceList,source.getDeptId()));
dataList.add(source);
}
}
return dataList;
}
public static List getParents(List sourceList, Integer currentId){
List dataList=new ArrayList<>();
sourceList.stream().filter(a->a.getDeptId().equals(currentId)).forEach(a->{
dataList.add(a);
if(a.getParentId()!=null){
dataList.addAll(getParents(sourceList,a.getParentId()));
}
});
return dataList;
}
public static List getChildrens(List sourceList, Integer currentId){
List dataList=new ArrayList<>();
sourceList.stream().filter(a->a.getDeptId().equals(currentId)).forEach(a->{
dataList.add(a);
sourceList.stream().filter(b->b.getParentId()!=null && b.getParentId().equals(currentId)).forEach(b->{
dataList.addAll(getChildrens(sourceList,b.getDeptId()));
});
});
return dataList;
}
public Integer getDeptId() {
return deptId;
}
public void setDeptId(Integer deptId) {
this.deptId = deptId;
}
public String getDeptName() {
return deptName;
}
public void setDeptName(String deptName) {
this.deptName = deptName;
}
public Integer getParentId() {
return parentId;
}
public void setParentId(Integer parentId) {
this.parentId = parentId;
}
public Integer getDeptOrder() {
return deptOrder;
}
public void setDeptOrder(Integer deptOrder) {
this.deptOrder = deptOrder;
}
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
public Boolean getDel() {
return isDel;
}
public void setDel(Boolean del) {
isDel = del;
}
public LocalDateTime getCreateTime() {
return createTime;
}
public void setCreateTime(LocalDateTime createTime) {
this.createTime = createTime;
}
public Long getCreateUserId() {
return createUserId;
}
public void setCreateUserId(Long createUserId) {
this.createUserId = createUserId;
}
public LocalDateTime getUpdateTime() {
return updateTime;
}
public void setUpdateTime(LocalDateTime updateTime) {
this.updateTime = updateTime;
}
public Long getUpdateUserId() {
return updateUserId;
}
public void setUpdateUserId(Long updateUserId) {
this.updateUserId = updateUserId;
}
public List getChildren() {
return children;
}
public void setChildren(List children) {
this.children = children;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy