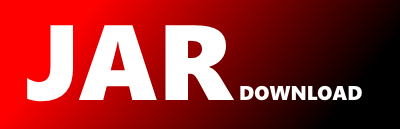
com.github.rexsheng.springboot.faster.system.dept.infrastructure.DeptGatewayImpl Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.dept.infrastructure;
import com.github.rexsheng.springboot.faster.system.dept.domain.SysDept;
import com.github.rexsheng.springboot.faster.system.dept.domain.gateway.DeptGateway;
import com.github.rexsheng.springboot.faster.system.dept.domain.gateway.QueryDeptDO;
import com.github.rexsheng.springboot.faster.system.entity.Dept;
import com.github.rexsheng.springboot.faster.system.entity.table.DeptTableDef;
import com.github.rexsheng.springboot.faster.system.mapper.DeptMapper;
import com.mybatisflex.core.BaseMapper;
import com.mybatisflex.core.query.QueryWrapper;
import com.mybatisflex.core.row.Db;
import com.mybatisflex.core.update.UpdateChain;
import com.mybatisflex.core.util.UpdateEntity;
import jakarta.annotation.Resource;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.stereotype.Repository;
import org.springframework.util.StringUtils;
import java.util.List;
import java.util.stream.Collectors;
@Repository
@ConditionalOnClass(BaseMapper.class)
public class DeptGatewayImpl implements DeptGateway {
@Resource
private DeptMapper deptMapper;
@Override
public void insertDept(SysDept dept) {
Dept target=new Dept();
target.setParentId(dept.getParentId());
target.setName(dept.getDeptName());
target.setOrder(dept.getDeptOrder());
target.setStatus(dept.getStatus());
target.setIsDel(dept.getDel());
target.setCreateTime(dept.getCreateTime());
target.setCreateUser(dept.getCreateUserId());
deptMapper.insert(target);
}
@Override
public List queryDepts(QueryDeptDO query) {
QueryWrapper queryWrapper=QueryWrapper.create().select()
.where(DeptTableDef.DEPT.IS_DEL.eq(false))
.orderBy(DeptTableDef.DEPT.ORDER,true)
.orderBy(DeptTableDef.DEPT.ID,true)
;
if(StringUtils.hasText(query.getName())){
queryWrapper.and(DeptTableDef.DEPT.NAME.like(query.getName()));
}
if(query.getStatus()!=null){
queryWrapper.and(DeptTableDef.DEPT.STATUS.eq(query.getStatus()));
}
List dataList=deptMapper.selectListByQuery(queryWrapper);
return dataList.stream().map(this::map).collect(Collectors.toList());
}
@Override
public SysDept getDept(Integer deptId) {
Dept entity=deptMapper.selectOneById(deptId);
return map(entity);
}
private SysDept map(Dept source){
SysDept target=new SysDept();
target.setDeptId(source.getId());
target.setDeptName(source.getName());
target.setParentId(source.getParentId());
target.setDeptOrder(source.getOrder());
target.setStatus(source.getStatus());
target.setDel(source.getIsDel());
target.setCreateTime(source.getCreateTime());
target.setCreateUserId(source.getCreateUser());
target.setUpdateTime(source.getUpdateTime());
target.setUpdateUserId(source.getUpdateUser());
return target;
}
@Override
public void updateDeptById(SysDept dept) {
Dept entity= UpdateEntity.of(Dept.class,dept.getDeptId());
entity.setParentId(dept.getParentId());
entity.setName(dept.getDeptName());
entity.setOrder(dept.getDeptOrder());
entity.setUpdateTime(dept.getUpdateTime());
entity.setUpdateUser(dept.getUpdateUserId());
deptMapper.update(entity);
}
@Override
public void updateDeptStatus(List depts) {
Db.executeBatch(depts, 100, DeptMapper.class
, (mapper, row) -> {
UpdateChain.of(mapper)
.set(Dept::getStatus, row.getStatus())
.set(Dept::getUpdateTime,row.getUpdateTime())
.set(Dept::getUpdateUser,row.getUpdateUserId())
.where(Dept::getId).eq(row.getDeptId())
.update();
});
}
@Override
public void deleteDepts(List depts) {
Db.executeBatch(depts, 100, DeptMapper.class
, (mapper, row) -> {
UpdateChain.of(mapper)
.set(Dept::getIsDel, row.getDel())
.set(Dept::getUpdateTime,row.getUpdateTime())
.set(Dept::getUpdateUser,row.getUpdateUserId())
.where(Dept::getId).eq(row.getDeptId())
.update();
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy