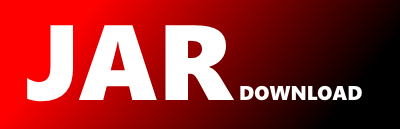
com.github.rexsheng.springboot.faster.system.dict.application.DictServiceImpl Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.dict.application;
import com.github.rexsheng.springboot.faster.common.domain.PagedList;
import com.github.rexsheng.springboot.faster.spring.SpringContext;
import com.github.rexsheng.springboot.faster.system.dict.application.dto.AddDictRequest;
import com.github.rexsheng.springboot.faster.system.dict.application.dto.DictDetailResponse;
import com.github.rexsheng.springboot.faster.system.dict.application.dto.QueryDictRequest;
import com.github.rexsheng.springboot.faster.system.dict.application.dto.UpdateDictRequest;
import com.github.rexsheng.springboot.faster.system.dict.domain.DictStateChangedEvent;
import com.github.rexsheng.springboot.faster.system.dict.domain.SysDict;
import com.github.rexsheng.springboot.faster.system.dict.domain.gateway.DictGateway;
import com.github.rexsheng.springboot.faster.system.dict.domain.gateway.QueryDictDO;
import jakarta.annotation.Resource;
import org.springframework.context.annotation.Lazy;
import org.springframework.stereotype.Service;
import org.springframework.util.Assert;
import java.util.List;
import java.util.stream.Collectors;
@Service
@Lazy
public class DictServiceImpl implements DictService{
@Resource
@Lazy
private DictGateway dictGateway;
@Override
public void add(AddDictRequest request) {
SysDict data=request.toDict();
dictGateway.insertDict(data);
SpringContext.getApplicationContext().publishEvent(new DictStateChangedEvent(data.getDictId(),DictStateChangedEvent.DictState.ADD));
}
@Override
public List queryList(QueryDictRequest request) {
QueryDictDO query=new QueryDictDO();
query.setLabel(request.getLabel());
query.setDictType(request.getDictType());
query.setStatus(request.getStatus());
query.setFetchDeleted(request.getFetchDeleted());
query.setJoinDictType(request.getFetchTypeCode());
List dataList=dictGateway.queryDicts(query);
return dataList.stream().map(DictDetailResponse::of).collect(Collectors.toList());
}
@Override
public PagedList pagedList(QueryDictRequest request) {
QueryDictDO query=new QueryDictDO();
query.setLabel(request.getLabel());
query.setDictType(request.getDictType());
query.setPageIndex(request.getPageIndex());
query.setPageSize(request.getPageSize());
PagedList dataList=dictGateway.paginateDicts(query);
return PagedList.of(dataList, DictDetailResponse::of);
}
@Override
public DictDetailResponse get(Long id) {
SysDict data= dictGateway.getDict(id);
Assert.notNull(data,"字典不存在");
return DictDetailResponse.of(data);
}
@Override
public void update(UpdateDictRequest request) {
SysDict data=request.toDict();
dictGateway.updateDictById(data);
}
@Override
public void updateStatus(List request) {
List dataList=request.stream().map(UpdateDictRequest::toDict).collect(Collectors.toList());
dictGateway.updateDictStatus(dataList);
SpringContext.getApplicationContext().publishEvent(new DictStateChangedEvent(DictStateChangedEvent.DictState.STATUS_CHANGED));
}
@Override
public void delete(List ids) {
dictGateway.deleteDicts(SysDict.of(ids,true));
SpringContext.getApplicationContext().publishEvent(new DictStateChangedEvent(ids,DictStateChangedEvent.DictState.DELETE));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy