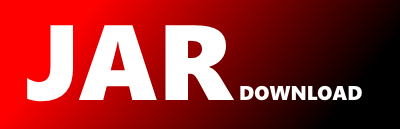
com.github.rexsheng.springboot.faster.system.dict.infrastructure.DictGatewayImpl Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.dict.infrastructure;
import com.github.rexsheng.springboot.faster.common.domain.PagedList;
import com.github.rexsheng.springboot.faster.system.dict.domain.SysDict;
import com.github.rexsheng.springboot.faster.system.dict.domain.SysDictType;
import com.github.rexsheng.springboot.faster.system.dict.domain.gateway.DictGateway;
import com.github.rexsheng.springboot.faster.system.dict.domain.gateway.QueryDictDO;
import com.github.rexsheng.springboot.faster.system.entity.Dict;
import com.github.rexsheng.springboot.faster.system.entity.table.DictTableDef;
import com.github.rexsheng.springboot.faster.system.entity.table.DictTypeTableDef;
import com.github.rexsheng.springboot.faster.system.mapper.DictMapper;
import com.github.rexsheng.springboot.faster.system.utils.PageConverter;
import com.mybatisflex.core.BaseMapper;
import com.mybatisflex.core.paginate.Page;
import com.mybatisflex.core.query.QueryWrapper;
import com.mybatisflex.core.row.Db;
import com.mybatisflex.core.update.UpdateChain;
import com.mybatisflex.core.util.UpdateEntity;
import jakarta.annotation.Resource;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.stereotype.Repository;
import org.springframework.util.StringUtils;
import java.util.List;
import java.util.stream.Collectors;
@Repository
@ConditionalOnClass(BaseMapper.class)
public class DictGatewayImpl implements DictGateway {
@Resource
private DictMapper dictMapper;
@Override
public void insertDict(SysDict dict) {
Dict target=new Dict();
target.setValue(dict.getDictValue());
target.setLabelZh(dict.getZhLabel());
target.setLabelEn(dict.getEnLabel());
target.setTypeId(dict.getDictType().getDictTypeId());
target.setRemark(dict.getRemark());
target.setStatus(dict.getStatus());
target.setIsDel(dict.getDel());
target.setCreateTime(dict.getCreateTime());
target.setCreateUser(dict.getCreateUserId());
dictMapper.insert(target);
dict.setDictId(target.getId());
}
@Override
public List queryDicts(QueryDictDO query) {
QueryWrapper queryWrapper=QueryWrapper.create()
.where(DictTableDef.DICT.IS_DEL.eq(false).when(!Boolean.TRUE.equals(query.getFetchDeleted())))
.orderBy(DictTableDef.DICT.TYPE_ID,true)
.orderBy(DictTableDef.DICT.ID,true);
if(query.getDictType()!=null){
queryWrapper.where(DictTableDef.DICT.TYPE_ID.eq(query.getDictType()));
}
if(StringUtils.hasText(query.getLabel())){
queryWrapper.where(DictTableDef.DICT.LABEL_ZH.like(query.getLabel()).or(DictTableDef.DICT.LABEL_EN.like(query.getLabel())));
}
if(query.getStatus()!=null){
queryWrapper.and(DictTableDef.DICT.STATUS.eq(query.getStatus()));
}
if(Boolean.TRUE.equals(query.getJoinDictType())){
queryWrapper.select(DictTableDef.DICT.ALL_COLUMNS,
DictTypeTableDef.DICT_TYPE.CODE.as(DictDO::getDictTypeCode),
DictTypeTableDef.DICT_TYPE.STATUS.as(DictDO::getDictTypeStatus),
DictTypeTableDef.DICT_TYPE.IS_DEL.as(DictDO::getDictTypeDel))
.innerJoin(DictTypeTableDef.DICT_TYPE).on(DictTableDef.DICT.TYPE_ID.eq(DictTypeTableDef.DICT_TYPE.ID))
.where(DictTypeTableDef.DICT_TYPE.IS_DEL.eq(false).when(!Boolean.TRUE.equals(query.getFetchDeleted())));
}
List dataList=dictMapper.selectListByQueryAs(queryWrapper,DictDO.class);
return dataList.stream().map(this::map).collect(Collectors.toList());
}
@Override
public PagedList paginateDicts(QueryDictDO query) {
QueryWrapper queryWrapper=QueryWrapper.create().select()
.where(DictTableDef.DICT.IS_DEL.eq(false))
.orderBy(DictTableDef.DICT.TYPE_ID,true)
.orderBy(DictTableDef.DICT.CREATE_TIME,true);
if(query.getDictType()!=null){
queryWrapper.where(DictTableDef.DICT.TYPE_ID.eq(query.getDictType()));
}
if(StringUtils.hasText(query.getLabel())){
queryWrapper.where(DictTableDef.DICT.LABEL_ZH.like(query.getLabel()).or(DictTableDef.DICT.LABEL_EN.like(query.getLabel())));
}
Page sourceList=dictMapper.paginate(query.getPageIndex(),query.getPageSize(),queryWrapper);
return PageConverter.convert(sourceList,this::map);
}
@Override
public SysDict getDict(Long dictId) {
Dict entity=dictMapper.selectOneById(dictId);
return map(entity);
}
private SysDict map(Dict dict){
SysDict target=new SysDict();
target.setDictId(dict.getId());
target.setDictValue(dict.getValue());
target.setZhLabel(dict.getLabelZh());
target.setEnLabel(dict.getLabelEn());
SysDictType sysDictType=new SysDictType(dict.getTypeId());
if(dict instanceof DictDO dictDO){
sysDictType.setDictTypeCode(dictDO.getDictTypeCode());
sysDictType.setStatus(dictDO.getDictTypeStatus());
sysDictType.setDel(dictDO.getDictTypeDel());
}
target.setDictType(sysDictType);
target.setRemark(dict.getRemark());
target.setStatus(dict.getStatus());
target.setDel(dict.getIsDel());
target.setCreateTime(dict.getCreateTime());
target.setCreateUserId(dict.getCreateUser());
target.setUpdateTime(dict.getUpdateTime());
target.setUpdateUserId(dict.getUpdateUser());
return target;
}
@Override
public void updateDictById(SysDict dict) {
Dict entity= UpdateEntity.of(Dict.class,dict.getDictId());
entity.setValue(dict.getDictValue());
entity.setTypeId(dict.getDictType().getDictTypeId());
entity.setLabelZh(dict.getZhLabel());
entity.setLabelEn(dict.getEnLabel());
entity.setUpdateTime(dict.getUpdateTime());
entity.setUpdateUser(dict.getUpdateUserId());
dictMapper.update(entity);
}
@Override
public void updateDictStatus(List dicts) {
Db.executeBatch(dicts, 100, DictMapper.class
, (mapper, row) -> {
UpdateChain.of(mapper)
.set(Dict::getStatus, row.getStatus())
.set(Dict::getUpdateTime,row.getUpdateTime())
.set(Dict::getUpdateUser,row.getUpdateUserId())
.where(Dict::getId).eq(row.getDictId())
.update();
});
}
@Override
public void deleteDicts(List dicts) {
Db.executeBatch(dicts, 100, DictMapper.class
, (mapper, row) -> {
UpdateChain.of(mapper)
.set(Dict::getIsDel, row.getDel())
.set(Dict::getUpdateTime,row.getUpdateTime())
.set(Dict::getUpdateUser,row.getUpdateUserId())
.where(Dict::getId).eq(row.getDictId())
.update();
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy