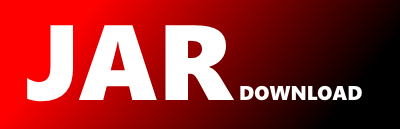
com.github.rexsheng.springboot.faster.system.entity.Menu Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.entity;
import com.mybatisflex.annotation.Id;
import com.mybatisflex.annotation.KeyType;
import com.mybatisflex.annotation.Table;
import java.io.Serializable;
import java.time.LocalDateTime;
import java.io.Serial;
/**
* 菜单表 实体类。
*
* @author sheng
* @since 2024-04-06
*/
@Table(value = "sys_menu")
public class Menu implements Serializable {
@Serial
private static final long serialVersionUID = 1L;
/**
* 菜单ID
*/
@Id(keyType = KeyType.Auto)
private Integer id;
/**
* 菜单编码
*/
private String code;
/**
* 菜单名称
*/
private String name;
/**
* 菜单序号
*/
private Integer order;
/**
* 路由地址
*/
private String path;
/**
* 路由参数
*/
private String query;
/**
* 前端组件地址
*/
private String component;
/**
* 上级菜单ID
*/
private Integer parentId;
/**
* 菜单图标
*/
private String icon;
/**
* 菜单类型(0菜单,1按钮)
*/
private Integer type;
/**
* 是否可见(0显示,1隐藏)
*/
private Boolean isVisible;
/**
* 是否为外部链接(0否,1是)
*/
private Boolean isFrame;
/**
* 是否缓存(0否,1是)
*/
private Boolean isCache;
/**
* 是否全屏(0否,1是)
*/
private Boolean isFullscreen;
/**
* 是否新标签(0否,1是)
*/
private Boolean isTag;
/**
* 状态(0正常,1停用)
*/
private Integer status;
/**
* 是否删除(0未删除,1已删除)
*/
private Boolean isDel;
/**
* 创建时间
*/
private LocalDateTime createTime;
/**
* 创建人
*/
private Long createUser;
/**
* 修改时间
*/
private LocalDateTime updateTime;
/**
* 修改人
*/
private Long updateUser;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getOrder() {
return order;
}
public void setOrder(Integer order) {
this.order = order;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
public String getQuery() {
return query;
}
public void setQuery(String query) {
this.query = query;
}
public String getComponent() {
return component;
}
public void setComponent(String component) {
this.component = component;
}
public Integer getParentId() {
return parentId;
}
public void setParentId(Integer parentId) {
this.parentId = parentId;
}
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = icon;
}
public Integer getType() {
return type;
}
public void setType(Integer type) {
this.type = type;
}
public Boolean getIsVisible() {
return isVisible;
}
public void setIsVisible(Boolean isVisible) {
this.isVisible = isVisible;
}
public Boolean getIsFrame() {
return isFrame;
}
public void setIsFrame(Boolean isFrame) {
this.isFrame = isFrame;
}
public Boolean getIsCache() {
return isCache;
}
public void setIsCache(Boolean isCache) {
this.isCache = isCache;
}
public Boolean getIsFullscreen() {
return isFullscreen;
}
public void setIsFullscreen(Boolean isFullscreen) {
this.isFullscreen = isFullscreen;
}
public Boolean getIsTag() {
return isTag;
}
public void setIsTag(Boolean isTag) {
this.isTag = isTag;
}
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
public Boolean getIsDel() {
return isDel;
}
public void setIsDel(Boolean isDel) {
this.isDel = isDel;
}
public LocalDateTime getCreateTime() {
return createTime;
}
public void setCreateTime(LocalDateTime createTime) {
this.createTime = createTime;
}
public Long getCreateUser() {
return createUser;
}
public void setCreateUser(Long createUser) {
this.createUser = createUser;
}
public LocalDateTime getUpdateTime() {
return updateTime;
}
public void setUpdateTime(LocalDateTime updateTime) {
this.updateTime = updateTime;
}
public Long getUpdateUser() {
return updateUser;
}
public void setUpdateUser(Long updateUser) {
this.updateUser = updateUser;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy