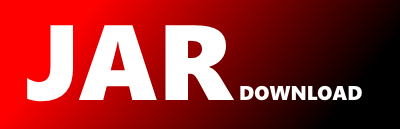
com.github.rexsheng.springboot.faster.system.file.infrastructure.FileGatewayImpl Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.file.infrastructure;
import com.github.rexsheng.springboot.faster.system.entity.File;
import com.github.rexsheng.springboot.faster.system.entity.table.FileTableDef;
import com.github.rexsheng.springboot.faster.system.file.domain.SysFile;
import com.github.rexsheng.springboot.faster.system.file.domain.gateway.DeleteFileDO;
import com.github.rexsheng.springboot.faster.system.file.domain.gateway.FileGateway;
import com.github.rexsheng.springboot.faster.system.file.domain.gateway.QueryFileDO;
import com.github.rexsheng.springboot.faster.system.mapper.FileMapper;
import com.github.rexsheng.springboot.faster.system.utils.AuthenticationUtil;
import com.github.rexsheng.springboot.faster.util.DateUtil;
import com.mybatisflex.core.BaseMapper;
import com.mybatisflex.core.query.QueryWrapper;
import com.mybatisflex.core.row.Db;
import com.mybatisflex.core.update.UpdateChain;
import jakarta.annotation.Resource;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.stereotype.Repository;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.util.ObjectUtils;
import org.springframework.util.StringUtils;
import java.util.List;
import java.util.stream.Collectors;
@Repository
@ConditionalOnClass(BaseMapper.class)
public class FileGatewayImpl implements FileGateway {
private static final Logger logger = LoggerFactory.getLogger(FileGatewayImpl.class);
@Resource
private FileMapper fileMapper;
@Override
public Long insertFile(SysFile file) {
File entity = new File();
entity.setBusinessType(file.getBusinessType());
entity.setBusinessId(file.getBusinessId());
entity.setService(file.getServiceName());
entity.setBucket(file.getBucketName());
entity.setKey(file.getObjectKey());
entity.setName(file.getFileName());
entity.setSize(file.getFileSize());
entity.setOriginalName(file.getOriginalFileName());
entity.setIsDel(file.getDel());
entity.setCreateTime(file.getCreateTime());
entity.setCreateUser(file.getCreateUserId());
fileMapper.insert(entity);
return entity.getId();
}
@Override
public SysFile getFile(Long id) {
File entity = fileMapper.selectOneById(id);
return map(entity);
}
@Override
public List queryFiles(QueryFileDO query) {
QueryWrapper queryWrapper = QueryWrapper.create().select()
.from(FileTableDef.FILE)
.where(FileTableDef.FILE.BUSINESS_TYPE.eq(query.getBusinessType(), StringUtils::hasText))
.and(FileTableDef.FILE.BUSINESS_ID.eq(query.getBusinessId(),StringUtils::hasText))
;
if(!ObjectUtils.isEmpty(query.getFileIds())){
queryWrapper.and(FileTableDef.FILE.ID.in(query.getFileIds().stream().map(SysFile::decryptFileId).collect(Collectors.toList())));
}
queryWrapper.and(FileTableDef.FILE.IS_DEL.eq(false));
List dataList=fileMapper.selectListByQuery(queryWrapper);
return dataList.stream().map(this::map).collect(Collectors.toList());
}
private SysFile map(File source){
SysFile target=new SysFile();
target.setFileId(source.getId());
target.setBusinessType(source.getBusinessType());
target.setBusinessId(source.getBusinessId());
target.setServiceName(source.getService());
target.setBucketName(source.getBucket());
target.setObjectKey(source.getKey());
target.setFileName(source.getName());
target.setFileSize(source.getSize());
target.setOriginalFileName(source.getOriginalName());
target.setDel(source.getIsDel());
target.setCreateTime(source.getCreateTime());
target.setCreateUserId(source.getCreateUser());
target.setUpdateTime(source.getUpdateTime());
target.setUpdateUserId(source.getUpdateUser());
return target;
}
@Override
@Transactional
public void deleteFiles(List files) {
Db.executeBatch(files, 20, FileMapper.class
, (mapper, row) -> {
UpdateChain.of(mapper)
.set(File::getIsDel, row.getDel())
.set(File::getUpdateTime,row.getUpdateTime())
.set(File::getUpdateUser,row.getUpdateUserId())
.where(File::getId).eq(row.getFileId())
.update();
});
}
@Override
public void deleteFiles(DeleteFileDO file) {
QueryWrapper queryWrapper = QueryWrapper.create().select()
.from(FileTableDef.FILE)
.where(FileTableDef.FILE.BUSINESS_TYPE.eq(file.getBusinessType(),StringUtils::hasText))
.and(FileTableDef.FILE.BUSINESS_ID.eq(file.getBusinessId(),StringUtils::hasText))
.and(FileTableDef.FILE.ID.in(file.getFileIds().stream().map(SysFile::decryptFileId).collect(Collectors.toList()), !ObjectUtils.isEmpty(file.getFileIds())))
.and(FileTableDef.FILE.IS_DEL.eq(false))
;
File entity = new File();
entity.setIsDel(true);
entity.setUpdateTime(DateUtil.currentDateTime());
entity.setUpdateUser(AuthenticationUtil.currentUserId());
fileMapper.updateByQuery(entity,queryWrapper);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy