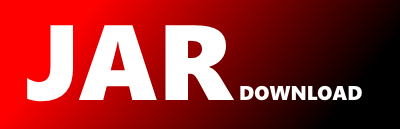
com.github.rexsheng.springboot.faster.system.job.infrastructure.JobGatewayImpl Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.job.infrastructure;
import com.github.rexsheng.springboot.faster.common.constant.CommonConstant;
import com.github.rexsheng.springboot.faster.common.domain.PagedList;
import com.github.rexsheng.springboot.faster.system.entity.*;
import com.github.rexsheng.springboot.faster.system.entity.table.JobLogDetailTableDef;
import com.github.rexsheng.springboot.faster.system.entity.table.JobLogTableDef;
import com.github.rexsheng.springboot.faster.system.entity.table.JobTableDef;
import com.github.rexsheng.springboot.faster.system.entity.table.JobTriggerTableDef;
import com.github.rexsheng.springboot.faster.system.job.domain.SysJob;
import com.github.rexsheng.springboot.faster.system.job.domain.gateway.JobGateway;
import com.github.rexsheng.springboot.faster.system.job.domain.gateway.QueryJobDO;
import com.github.rexsheng.springboot.faster.system.job.domain.gateway.QueryJobLogDO;
import com.github.rexsheng.springboot.faster.system.job.domain.gateway.QueryTriggerDO;
import com.github.rexsheng.springboot.faster.system.mapper.JobLogDetailMapper;
import com.github.rexsheng.springboot.faster.system.mapper.JobLogMapper;
import com.github.rexsheng.springboot.faster.system.mapper.JobMapper;
import com.github.rexsheng.springboot.faster.system.mapper.JobTriggerMapper;
import com.github.rexsheng.springboot.faster.system.utils.PageConverter;
import com.mybatisflex.core.BaseMapper;
import com.mybatisflex.core.paginate.Page;
import com.mybatisflex.core.query.QueryWrapper;
import com.mybatisflex.core.row.Db;
import com.mybatisflex.core.update.UpdateChain;
import com.mybatisflex.core.util.UpdateEntity;
import jakarta.annotation.Resource;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.stereotype.Repository;
import org.springframework.util.StringUtils;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
@Repository
@ConditionalOnClass(BaseMapper.class)
public class JobGatewayImpl implements JobGateway {
@Resource
private JobMapper jobMapper;
@Resource
private JobTriggerMapper jobTriggerMapper;
@Resource
private JobLogMapper jobLogMapper;
@Resource
private JobLogDetailMapper jobLogDetailMapper;
@Override
public PagedList paginateJobs(QueryJobDO query) {
QueryWrapper queryWrapper=QueryWrapper.create().select()
.where(JobTableDef.JOB.IS_DEL.eq(false))
;
queryWrapper.and(JobTableDef.JOB.STATUS.eq(query.getStatus()).when(query.getStatus()!=null));
if(StringUtils.hasText(query.getName())){
queryWrapper.and(JobTableDef.JOB.NAME.like(query.getName()).or(JobTableDef.JOB.EXEC_CLAZZ.like(query.getName())));
}
Page sourceList=jobMapper.paginate(query.getPageIndex(),query.getPageSize(),queryWrapper);
return PageConverter.convert(sourceList,this::map);
}
@Override
public List queryJobs(QueryJobDO query) {
QueryWrapper queryWrapper=QueryWrapper.create().select()
.where(JobTableDef.JOB.IS_DEL.eq(false))
;
queryWrapper.and(JobTableDef.JOB.STATUS.eq(query.getStatus()).when(query.getStatus()!=null));
if(StringUtils.hasText(query.getName())){
queryWrapper.and(JobTableDef.JOB.NAME.like(query.getName()).or(JobTableDef.JOB.EXEC_CLAZZ.like(query.getName())));
}
if(query.isPagerRequest()){
queryWrapper.limit(query.offset(),query.getPageSize());
}
List sourceList=jobMapper.selectListByQuery(queryWrapper);
return sourceList.stream().map(this::map).collect(Collectors.toList());
}
@Override
public Long insertJob(SysJob job) {
Job target = new Job();
target.setName(job.getJobName());
target.setGroup(job.getJobGroup());
target.setConcurrent(job.getConcurrency());
target.setExecClazz(job.getExecuteClass());
target.setExecMethod(job.getExecuteMethod());
target.setExecParam(SysJob.mapToJson(job.getExecuteParameter()));
target.setRemark(job.getRemark());
target.setErrorMail(job.getErrorMail());
target.setStatus(job.getStatus());
target.setIsDel(job.getDel());
target.setCreateTime(job.getCreateTime());
target.setCreateUser(job.getCreateUserId());
jobMapper.insert(target);
return target.getId();
}
@Override
public Long insertTrigger(SysJob job) {
JobTrigger target = new JobTrigger();
SysJob.SysJobTrigger source=job.getTriggerList().get(0);
target.setJobId(source.getJobId());
target.setName(source.getTriggerName());
target.setGroup(source.getTriggerGroup());
target.setExecType(source.getExecuteType());
target.setExecExpression(CommonConstant.JOB_TRIGGER_TYPE_CRON.equals(source.getExecuteType())?source.getExecuteExpression(): SysJob.mapToJson(source.getTriggerInterval()));
target.setExecStart(source.getStartTime());
target.setExecEnd(source.getEndTime());
target.setMisfireStrategy(source.getMisfireStrategy());
target.setPriority(source.getPriority());
target.setStatus(source.getStatus());
target.setIsDel(source.getDel());
target.setCreateTime(source.getCreateTime());
target.setCreateUser(source.getCreateUserId());
jobTriggerMapper.insert(target);
return target.getId();
}
@Override
public void insertJobLog(SysJob job) {
JobLog entity = new JobLog();
entity.setJobId(job.getJobId());
entity.setId(job.getJobLog().getJobLogId());
entity.setTriggerId(job.getJobLog().getTriggerId());
entity.setStartTime(job.getJobLog().getStartTime());
entity.setEndTime(job.getJobLog().getEndTime());
entity.setCostTime(job.getJobLog().getCostTime());
entity.setState(job.getJobLog().getState());
entity.setExecClazz(job.getJobLog().getExecuteClass());
entity.setExecMethod(job.getJobLog().getExecuteMethod());
entity.setExecParam(job.getJobLog().getExecuteParameter());
entity.setJobResult(job.getJobLog().getJobResult());
entity.setHostIp(job.getJobLog().getHostIp());
entity.setHostName(job.getJobLog().getHostName());
jobLogMapper.insertOrUpdate(entity,true);
job.getJobLog().setJobLogId(entity.getId());
}
@Override
public void insertJobLogDetail(SysJob job) {
Optional.ofNullable(job.getJobLog().getLogDetails()).ifPresent(list->{
list.stream().filter(log->log.getLogDetailId()==null).forEach(log->{
JobLogDetail entity = new JobLogDetail();
entity.setCreateTime(log.getCreateTime());
entity.setLevel(log.getLevel());
entity.setMsg(log.getMsg());
entity.setJobLogId(job.getJobLog().getJobLogId());
jobLogDetailMapper.insert(entity);
log.setLogDetailId(entity.getId());
});
});
}
@Override
public SysJob getJob(Long id) {
Job entity=jobMapper.selectOneById(id);
return map(entity);
}
@Override
public void updateJobById(SysJob job) {
Job target= UpdateEntity.of(Job.class,job.getJobId());
target.setName(job.getJobName());
target.setGroup(job.getJobGroup());
target.setConcurrent(job.getConcurrency());
target.setExecClazz(job.getExecuteClass());
target.setExecMethod(job.getExecuteMethod());
target.setExecParam(SysJob.mapToJson(job.getExecuteParameter()));
target.setRemark(job.getRemark());
target.setErrorMail(job.getErrorMail());
target.setStatus(job.getStatus());
target.setUpdateTime(job.getUpdateTime());
target.setUpdateUser(job.getUpdateUserId());
jobMapper.update(target);
}
@Override
public void updateJobStatus(List jobs) {
Db.executeBatch(jobs, 100, JobMapper.class
, (mapper, row) -> {
UpdateChain.of(mapper)
.set(Job::getStatus, row.getStatus())
.set(Job::getUpdateTime,row.getUpdateTime())
.set(Job::getUpdateUser,row.getUpdateUserId())
.where(Job::getId).eq(row.getJobId())
.update();
});
}
@Override
public void deleteJobs(List jobs) {
Db.executeBatch(jobs, 100, JobMapper.class
, (mapper, row) -> {
UpdateChain.of(mapper)
.set(Job::getIsDel, row.getDel())
.set(Job::getUpdateTime,row.getUpdateTime())
.set(Job::getUpdateUser,row.getUpdateUserId())
.where(Job::getId).eq(row.getJobId())
.update();
});
}
private SysJob map(Job source){
SysJob target=new SysJob();
target.setJobId(source.getId());
target.setJobName(source.getName());
target.setRemark(source.getRemark());
target.setExecuteClass(source.getExecClazz());
target.setExecuteMethod(source.getExecMethod());
target.setExecuteParameter(SysJob.jsonToMap(source.getExecParam()));
target.setConcurrency(source.getConcurrent());
target.setErrorMail(source.getErrorMail());
target.setStatus(source.getStatus());
target.setDel(source.getIsDel());
target.setCreateTime(source.getCreateTime());
target.setCreateUserId(source.getCreateUser());
target.setUpdateTime(source.getUpdateTime());
target.setUpdateUserId(source.getUpdateUser());
return target;
}
private SysJob map(JobLog source){
SysJob target=new SysJob();
target.setJobId(source.getJobId());
SysJob.SysJobLog jobLog=new SysJob.SysJobLog();
jobLog.setJobLogId(source.getId());
jobLog.setTriggerId(source.getTriggerId());
jobLog.setStartTime(source.getStartTime());
jobLog.setEndTime(source.getEndTime());
jobLog.setCostTime(source.getCostTime());
jobLog.setState(source.getState());
jobLog.setExecuteClass(source.getExecClazz());
jobLog.setExecuteMethod(source.getExecMethod());
jobLog.setExecuteParameter(source.getExecParam());
jobLog.setJobResult(source.getJobResult());
jobLog.setHostName(source.getHostName());
jobLog.setHostIp(source.getHostIp());
target.setJobLog(jobLog);
return target;
}
private SysJob.SysJobLogDetail map(JobLogDetail source){
SysJob.SysJobLogDetail target=new SysJob.SysJobLogDetail();
target.setLogDetailId(source.getId());
target.setLevel(source.getLevel());
target.setMsg(source.getMsg());
target.setCreateTime(source.getCreateTime());
return target;
}
private SysJob.SysJobTrigger map(JobTrigger source){
SysJob.SysJobTrigger target=new SysJob.SysJobTrigger();
target.setJobId(source.getJobId());
target.setTriggerId(source.getId());
target.setTriggerName(source.getName());
target.setTriggerGroup(source.getGroup());
target.setExecuteType(source.getExecType());
if(CommonConstant.JOB_TRIGGER_TYPE_CRON.equals(source.getExecType())){
target.setExecuteExpression(source.getExecExpression());
}
else{
target.setTriggerInterval(SysJob.jsonToMap(source.getExecExpression()));
}
target.setStartTime(source.getExecStart());
target.setEndTime(source.getExecEnd());
target.setMisfireStrategy(source.getMisfireStrategy());
target.setPriority(source.getPriority());
target.setStatus(source.getStatus());
target.setDel(source.getIsDel());
target.setCreateTime(source.getCreateTime());
target.setCreateUserId(source.getCreateUser());
target.setUpdateTime(source.getUpdateTime());
target.setUpdateUserId(source.getUpdateUser());
return target;
}
@Override
public PagedList paginateJobLogs(QueryJobLogDO query) {
QueryWrapper queryWrapper=QueryWrapper.create().select().from(JobLogTableDef.JOB_LOG)
.where(JobLogTableDef.JOB_LOG.JOB_ID.eq(query.getJobId())).orderBy(JobLogTableDef.JOB_LOG.START_TIME.desc());
if(query.getState()!=null){
queryWrapper.and(JobLogTableDef.JOB_LOG.STATE.eq(query.getState()));
}
if(query.getTriggerId()!=null){
queryWrapper.and(JobLogTableDef.JOB_LOG.TRIGGER_ID.eq(query.getTriggerId()));
}
if(query.getStartTime()!=null){
queryWrapper.and(JobLogTableDef.JOB_LOG.START_TIME.ge(query.getStartTime()));
}
if(query.getEndTime()!=null){
queryWrapper.and(JobLogTableDef.JOB_LOG.END_TIME.le(query.getEndTime()));
}
Page sourceList=jobLogMapper.paginate(query.getPageIndex(),query.getPageSize(),queryWrapper);
return PageConverter.convert(sourceList,this::map);
}
@Override
public PagedList paginateJobLogDetails(QueryJobLogDO query) {
QueryWrapper queryWrapper=QueryWrapper.create().select()
.where(JobLogDetailTableDef.JOB_LOG_DETAIL.JOB_LOG_ID.eq(query.getJobLogId())).orderBy(JobLogDetailTableDef.JOB_LOG_DETAIL.ID.asc());
Page sourceList=jobLogDetailMapper.paginate(query.getPageIndex(),query.getPageSize(),queryWrapper);
return PageConverter.convert(sourceList,this::map);
}
@Override
public SysJob.SysJobTrigger getTrigger(Long id) {
JobTrigger entity=jobTriggerMapper.selectOneById(id);
return map(entity);
}
@Override
public void updateTriggerById(SysJob job) {
SysJob.SysJobTrigger source=job.getTriggerList().get(0);
JobTrigger target= UpdateEntity.of(JobTrigger.class,source.getTriggerId());
target.setName(source.getTriggerName());
target.setGroup(source.getTriggerGroup());
target.setExecType(source.getExecuteType());
target.setExecExpression(CommonConstant.JOB_TRIGGER_TYPE_CRON.equals(source.getExecuteType())?source.getExecuteExpression(): SysJob.mapToJson(source.getTriggerInterval()));
target.setExecStart(source.getStartTime());
target.setExecEnd(source.getEndTime());
target.setMisfireStrategy(source.getMisfireStrategy());
target.setPriority(source.getPriority());
target.setStatus(source.getStatus());
target.setUpdateTime(source.getUpdateTime());
target.setUpdateUser(source.getUpdateUserId());
jobTriggerMapper.update(target);
}
@Override
public void updateTriggerStatus(List jobs) {
Db.executeBatch(jobs, 100, JobTriggerMapper.class
, (mapper, row) -> {
SysJob.SysJobTrigger source=row.getTriggerList().get(0);
UpdateChain.of(mapper)
.set(JobTrigger::getStatus, source.getStatus())
.set(JobTrigger::getUpdateTime,source.getUpdateTime())
.set(JobTrigger::getUpdateUser,source.getUpdateUserId())
.where(JobTrigger::getId).eq(source.getTriggerId())
.update();
});
}
@Override
public void updateTriggerStatus(Long id, Integer status) {
JobTrigger target= UpdateEntity.of(JobTrigger.class,id);
target.setStatus(status);
jobTriggerMapper.update(target);
}
@Override
public void deleteTriggers(List triggers) {
Db.executeBatch(triggers, 100, JobTriggerMapper.class
, (mapper, row) -> {
UpdateChain.of(mapper)
.set(JobTrigger::getIsDel, row.getDel())
.set(JobTrigger::getUpdateTime,row.getUpdateTime())
.set(JobTrigger::getUpdateUser,row.getUpdateUserId())
.where(JobTrigger::getId).eq(row.getTriggerId())
.update();
});
}
@Override
public List queryTriggers(QueryTriggerDO query) {
QueryWrapper queryWrapper=QueryWrapper.create().select()
.and(JobTriggerTableDef.JOB_TRIGGER.IS_DEL.eq(false))
.and(JobTriggerTableDef.JOB_TRIGGER.JOB_ID.eq(query.getJobId(),query.getJobId()!=null))
.and(JobTriggerTableDef.JOB_TRIGGER.STATUS.eq(query.getStatus()).when(query.getStatus()!=null));
List sourceList=jobTriggerMapper.selectListByQuery(queryWrapper);
List dataList=sourceList.stream().map(this::map).collect(Collectors.toList());
return dataList;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy