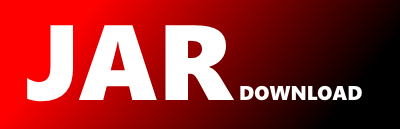
com.github.rexsheng.springboot.faster.system.locale.application.LocaleServiceImpl Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.locale.application;
import com.github.rexsheng.springboot.faster.function.Tuple2;
import com.github.rexsheng.springboot.faster.i18n.event.LocaleChangedEvent;
import com.github.rexsheng.springboot.faster.system.locale.application.dto.LocaleDetailOutput;
import com.github.rexsheng.springboot.faster.system.locale.application.dto.RefreshLocaleSourceRequest;
import com.github.rexsheng.springboot.faster.system.locale.domain.DictDomainService;
import com.github.rexsheng.springboot.faster.system.locale.domain.LocaleRefreshEvent;
import com.github.rexsheng.springboot.faster.system.locale.domain.SysLocaleSource;
import com.github.rexsheng.springboot.faster.system.locale.domain.gateway.LocaleGateway;
import jakarta.annotation.Resource;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.context.ApplicationListener;
import org.springframework.context.event.EventListener;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
import java.util.*;
import java.util.stream.Collectors;
@Service
@ConditionalOnClass(RedisTemplate.class)
public class LocaleServiceImpl implements LocaleService, ApplicationListener {
private static final Logger logger= LoggerFactory.getLogger(LocaleServiceImpl.class);
@Resource
private RedisTemplate redisTemplate;
@Resource
private StringRedisTemplate stringRedisTemplate;
@Resource
private DictDomainService dictDomainService;
@Resource
private LocaleGateway localeGateway;
@Override
public List getActivedLocaleSource() {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy