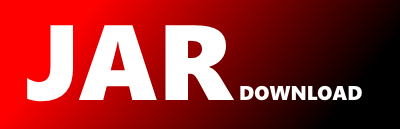
com.github.rexsheng.springboot.faster.system.locale.domain.SysLocaleSource Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.locale.domain;
import com.github.rexsheng.springboot.faster.common.constant.CommonConstant;
import com.github.rexsheng.springboot.faster.i18n.ObjectMessageSourceHelper;
import com.github.rexsheng.springboot.faster.system.dict.application.dto.DictDetailResponse;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Component;
import org.springframework.util.ObjectUtils;
import java.util.*;
import java.util.stream.Collectors;
@Component
@ConditionalOnClass(RedisTemplate.class)
public class SysLocaleSource {
private RedisTemplate redisTemplate;
private Locale locale;
private String dictValue;
private String zhLabel;
private String enLabel;
private Integer dictStatus;
private Boolean dictDel;
private Integer dictTypeId;
private String dictTypeCode;
private Integer dictTypeStatus;
private Boolean dictTypeDel;
public SysLocaleSource() {
}
public SysLocaleSource(RedisTemplate redisTemplate){
this.redisTemplate=redisTemplate;
}
public static SysLocaleSource of(DictDetailResponse dict){
SysLocaleSource target=new SysLocaleSource();
target.setDictValue(dict.getDictValue());
target.setZhLabel(dict.getZhLabel());
target.setEnLabel(dict.getEnLabel());
target.setDictStatus(dict.getStatus());
target.setDictDel(dict.getDel());
target.setDictTypeId(dict.getDictType());
target.setDictTypeCode(dict.getDictTypeCode());
target.setDictTypeDel(dict.getDictTypeDel());
target.setDictTypeStatus(dict.getDictTypeStatus());
return target;
}
public static Map> toLocaleMap(List list){
Map> resultMap=new LinkedHashMap<>();
if(!ObjectUtils.isEmpty(list)){
List activeList=filterByActived(list);
List inactiveList=list.stream().filter(a->!activeList.contains(a)).collect(Collectors.toList());
if(!ObjectUtils.isEmpty(activeList)){
Map zhMap=activeList.stream()
.filter(a->a.getZhLabel()!=null)
.collect(Collectors.toMap(a->ObjectMessageSourceHelper.combineKey(a.getDictTypeCode(),a.getDictValue()),
a->a.getZhLabel(),(oldValue, newValue)->newValue,LinkedHashMap::new));
resultMap.put(Locale.CHINA.toLanguageTag(),zhMap);
Map enMap=activeList.stream()
.filter(a->a.getEnLabel()!=null)
.collect(Collectors.toMap(a->ObjectMessageSourceHelper.combineKey(a.getDictTypeCode(),a.getDictValue()),
a->a.getEnLabel(),(oldValue,newValue)->newValue,LinkedHashMap::new));
resultMap.put(Locale.US.toLanguageTag(),enMap);
}
if(!ObjectUtils.isEmpty(inactiveList)){
Map zhMap=inactiveList.stream()
.filter(a->a.getZhLabel()!=null)
.collect(Collectors.toMap(a->ObjectMessageSourceHelper.combineKey(a.getDictTypeCode(),a.getDictValue()),
a->a.getZhLabel(),(oldValue, newValue)->newValue,LinkedHashMap::new));
resultMap.put(Locale.CHINA.toLanguageTag()+"_1",zhMap);
Map enMap=inactiveList.stream()
.filter(a->a.getEnLabel()!=null)
.collect(Collectors.toMap(a->ObjectMessageSourceHelper.combineKey(a.getDictTypeCode(),a.getDictValue()),
a->a.getEnLabel(),(oldValue,newValue)->newValue,LinkedHashMap::new));
resultMap.put(Locale.US.toLanguageTag()+"_1",enMap);
}
}
return resultMap;
}
public static List activeLocaleRedisKeys(){
return Arrays.asList(Locale.CHINA.toLanguageTag(),Locale.US.toLanguageTag());
}
public static List filterByActived(List list){
return list.stream()
.filter(a-> CommonConstant.STATUS_RUNNING.equals(a.getDictStatus())
&& !Boolean.TRUE.equals(a.getDictDel())
&& CommonConstant.STATUS_RUNNING.equals(a.getDictTypeStatus())
&& !Boolean.TRUE.equals(a.getDictTypeDel())
)
.collect(Collectors.toList());
}
public static List ofList(Map zhSource, Map enSource){
List list=new ArrayList<>();
if(zhSource!=null){
for(Map.Entry entry:zhSource.entrySet()){
SysLocaleSource target=new SysLocaleSource();
String[] keys=ObjectMessageSourceHelper.splitKey(entry.getKey());
if(keys.length>1){
target.setDictTypeCode(keys[0]);
target.setDictValue(keys[1]);
}
else{
target.setDictValue(keys[0]);
}
target.setZhLabel(entry.getValue());
if(enSource!=null){
target.setEnLabel(enSource.get(entry.getKey()));
}
list.add(target);
}
}
return list;
}
public Locale getLocale() {
return locale;
}
public void setLocale(Locale locale) {
this.locale = locale;
}
public String getDictValue() {
return dictValue;
}
public void setDictValue(String dictValue) {
this.dictValue = dictValue;
}
public String getZhLabel() {
return zhLabel;
}
public void setZhLabel(String zhLabel) {
this.zhLabel = zhLabel;
}
public String getEnLabel() {
return enLabel;
}
public void setEnLabel(String enLabel) {
this.enLabel = enLabel;
}
public Boolean getDictDel() {
return dictDel;
}
public void setDictDel(Boolean dictDel) {
this.dictDel = dictDel;
}
public Integer getDictTypeId() {
return dictTypeId;
}
public void setDictTypeId(Integer dictTypeId) {
this.dictTypeId = dictTypeId;
}
public Boolean getDictTypeDel() {
return dictTypeDel;
}
public void setDictTypeDel(Boolean dictTypeDel) {
this.dictTypeDel = dictTypeDel;
}
public String getDictTypeCode() {
return dictTypeCode;
}
public void setDictTypeCode(String dictTypeCode) {
this.dictTypeCode = dictTypeCode;
}
public RedisTemplate getRedisTemplate() {
return redisTemplate;
}
public void setRedisTemplate(RedisTemplate redisTemplate) {
this.redisTemplate = redisTemplate;
}
public Integer getDictStatus() {
return dictStatus;
}
public void setDictStatus(Integer dictStatus) {
this.dictStatus = dictStatus;
}
public Integer getDictTypeStatus() {
return dictTypeStatus;
}
public void setDictTypeStatus(Integer dictTypeStatus) {
this.dictTypeStatus = dictTypeStatus;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy